Reimagining Media ETL for scalable media workflows
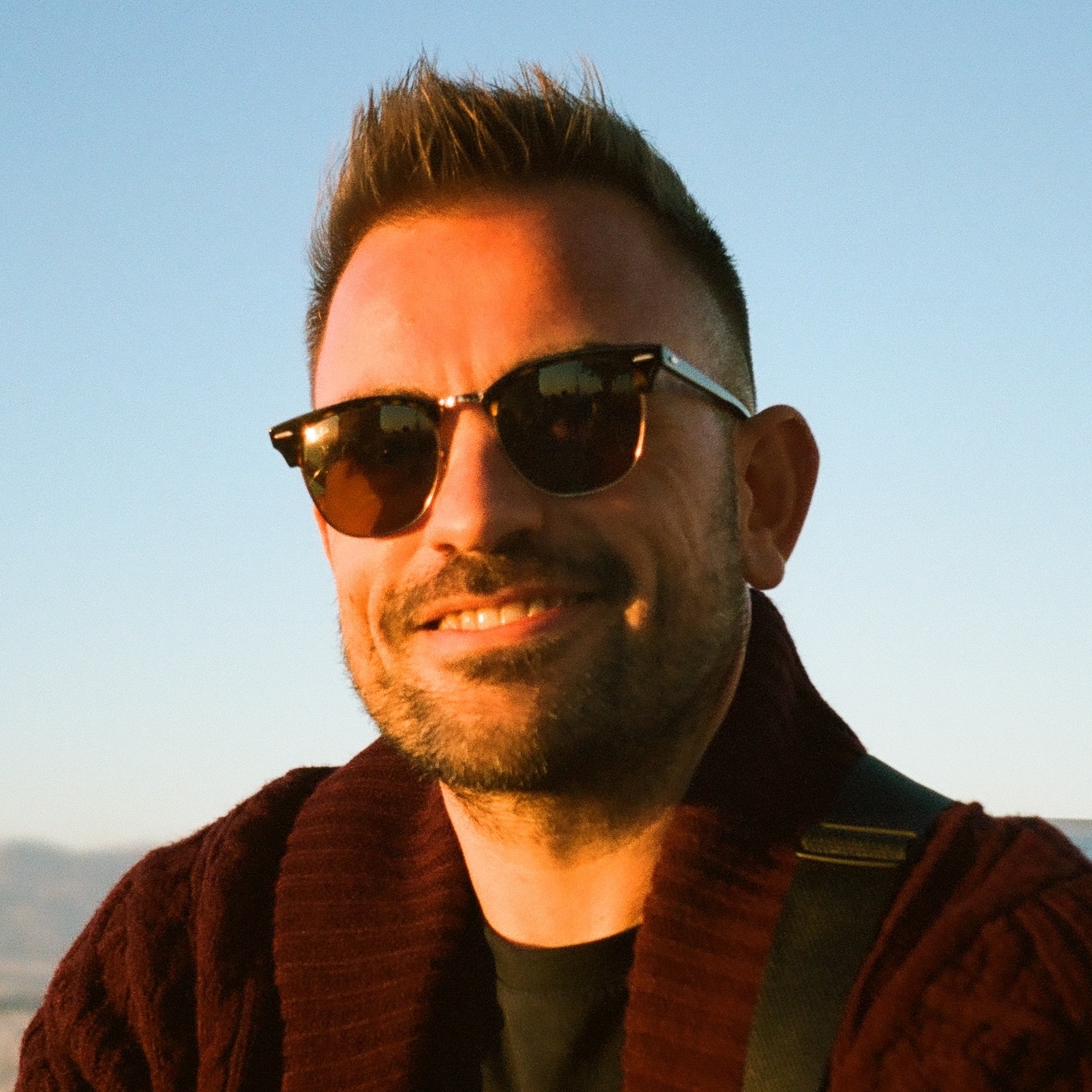
In the world of data engineering, ETL (Extract, Transform, Load) has been a cornerstone methodology for decades. But as digital media continues to explode in volume and importance, traditional ETL approaches fall short when handling videos, images, and audio files. This is where Transloadit comes in – offering what we like to call "Media ETL" – a specialized approach to processing media assets with the same reliability and scalability that data engineers expect from their ETL pipelines.
Traditional vs. Media ETL
Traditional ETL
Traditional ETL processes typically deal with structured data from databases, APIs, and applications. The workflow usually looks like this:
- Extract: Pull data from source systems like CRMs, ERPs, or operational databases
- Transform: Cleanse, normalize, and restructure the data
- Load: Place the transformed data into a target system like a data warehouse
These processes excel at handling tabular data but struggle with unstructured media files that require specialized processing.
Media ETL with Transloadit
Media ETL applies the same principles but is specifically designed for rich media assets:
- Extract: Ingest videos, images, audio, and documents from various sources
- Transform: Apply specialized media operations like transcoding, compression, resizing, and metadata extraction
- Load: Deliver processed media to storage services, CDNs, or applications
Why traditional ETL tools fall short for media
If you've ever tried to process videos or images at scale using traditional ETL tools, you've likely encountered these challenges:
- Resource intensity: Media processing requires significant CPU/GPU resources
- Format complexity: Dealing with hundreds of media formats and codecs
- Scale issues: Processing large media files can overwhelm standard ETL pipelines
- Specialized operations: Operations like video transcoding have no equivalent in data ETL
- Metadata extraction: Pulling metadata from media requires specialized tools
The Transloadit approach to Media ETL
Transloadit was built from the ground up to handle media ETL workflows with the same reliability that data engineers expect from their data pipelines. Here's how we approach it:
1. Media-specific extractors
Our platform can extract media content from virtually anywhere:
- Direct uploads from browsers and mobile devices
- Cloud storage services (S3, Google Cloud Storage, Azure Blob Storage)
- Existing web URLs
- FTP servers
- And more
// Example: Extracting a video from S3
{
"steps": {
"import": {
"robot": "/s3/import",
"bucket": "my-videos-bucket",
"path": "raw-footage/interview.mp4"
}
// Further steps follow
}
}
2. Powerful media transformations
Once your media is ingested, Transloadit offers hundreds of transformation options:
- Video: Transcoding, thumbnail extraction, watermarking, trimming
- Audio: Format conversion, normalization, waveform generation
- Images: Resizing, optimization, face detection, color correction
- Documents: Text extraction, preview generation, format conversion
// Example: Transforming a video into multiple formats
{
"steps": {
"encode": {
"use": "import",
"robot": "/video/encode",
"preset": "web/mp4/4k",
"ffmpeg_stack": "v6.0.0"
},
"thumbnail": {
"use": "import",
"robot": "/video/thumbs",
"count": 5,
"format": "jpg"
}
}
}
3. Flexible loading options
After transformation, Transloadit can load your processed media to virtually any destination:
- S3-compatible storage
- Google Cloud Storage
- Microsoft Azure
- Backblaze B2
- Direct download URLs
- Webhook notifications when processing completes
// Example: Loading processed videos to a different S3 bucket
{
"steps": {
"export": {
"use": ["encode", "thumbnail"],
"robot": "/s3/store",
"bucket": "my-processed-videos",
"path": "processed/${file.meta.videoId}/"
}
}
}
Intergration with Transloadit
To integrate Media ETL into your applications, you can use one of our many SDKs. Here's a quickstart with our SDK for Node.js:
npm install transloadit
Basic setup:
import Transloadit from 'transloadit'
const transloadit = new Transloadit({
authKey: 'YOUR_AUTH_KEY',
authSecret: 'YOUR_AUTH_SECRET',
})
Sign up for Transloadit to get your API key and secret.
Real-world use cases
Streaming service video pipeline
A streaming service uses Transloadit to process thousands of hours of video content daily:
- Extract: Ingest raw video files from content providers via S3
- Transform:
- Transcode into multiple adaptive bitrate formats (HLS, DASH)
- Generate thumbnails at various timestamps
- Extract metadata and closed captions
- Apply DRM protection
- Load: Deliver processed files to origin servers and CDNs
E-commerce product image processing
An e-commerce platform processes millions of product images:
- Extract: Receive raw product photos from vendors and photographers
- Transform:
- Generate multiple sizes for responsive web display
- Optimize for web delivery
- Create variants (zoom views, thumbnails)
- Remove backgrounds automatically
- Apply consistent white balance
- Load: Store in CDN-connected storage for fast delivery
Audio podcast distribution network
A podcast hosting company processes audio files for multi-platform distribution:
- Extract: Ingest raw podcast recordings from creators
- Transform:
- Normalize audio levels
- Generate compressed versions for streaming
- Create waveform visualizations
- Extract transcriptions using AI
- Split into chapters based on silence detection
- Load: Distribute to podcast platforms and archive in long-term storage
Technical comparison
Aspect | Traditional ETL | Media ETL with Transloadit |
---|---|---|
Data Types | Structured data (rows, columns) | Binary media files (video, audio, images) |
Processing Units | Records, rows, or documents | Media files, frames, or segments |
Resource Requirements | CPU and memory intensive | CPU, GPU, and specialized codec requirements |
Transformation Logic | SQL, scripting languages | Media-specific algorithms and codecs |
Scalability Challenges | Row volume, query complexity | File size, encoding complexity, resolution |
Error Handling | Field validation, type checking | Format compatibility, codec issues, corruption |
Processing Time | Often near real-time | Can require significant processing time for large media |
Building your Media ETL pipeline with Transloadit
Getting started with Media ETL is straightforward. Here's a simple workflow to convert videos for multi-platform delivery:
{
"steps": {
// Extract
"import": {
"robot": "/http/import",
"url": "https://example.com/raw-video.mp4"
},
// Transform - Create multiple formats
"mp4_high": {
"use": "import",
"robot": "/video/encode",
"preset": "web/mp4/1080p",
"ffmpeg_stack": "v6.0.0"
},
"mp4_low": {
"use": "import",
"robot": "/video/encode",
"preset": "web/mp4/480p",
"ffmpeg_stack": "v6.0.0"
},
"webm": {
"use": "import",
"robot": "/video/encode",
"preset": "web/webm/1080p",
"ffmpeg_stack": "v6.0.0"
},
// Create thumbnails
"thumbnails": {
"use": "import",
"robot": "/video/thumbs",
"count": 3,
"format": "jpg"
},
// Load to destination
"export": {
"use": ["mp4_high", "mp4_low", "webm", "thumbnails"],
"robot": "/s3/store",
"bucket": "processed-videos",
"path": "${file.originalPath}/${file.name}"
}
}
}
You would save this workflow as a Template in your Transloadit, and refer to it with the SDK.
When to choose Media ETL
Consider Transloadit's Media ETL approach when:
- Your data is primarily media files (videos, images, audio, documents)
- You need specialized media processing beyond what general-purpose ETL tools provide
- Scale matters – you're processing large volumes of media files
- Time-to-market is critical – building media processing pipelines from scratch is time-consuming
- You need a unified workflow for all your media processing needs
Integrating Media ETL with traditional data pipelines
Many organizations maintain both traditional data ETL pipelines and media ETL pipelines. Transloadit can integrate seamlessly with your existing data architecture:
- Metadata extraction: Extract metadata from media and feed it into your data warehouse
- Event-based triggers: Trigger Transloadit workflows from your existing ETL pipeline events
- Webhook notifications: Notify your data pipeline when media processing completes
- Joint reporting: Combine media processing metrics with your data pipeline metrics
Conclusion
As organizations continue to generate and consume more media content, the need for robust Media ETL pipelines will only grow. Traditional ETL approaches that work well for structured data simply aren't equipped to handle the unique challenges of media processing at scale.
Transloadit bridges this gap by providing a specialized Media ETL platform that applies the reliability, scalability, and flexibility that engineers expect from ETL processes to the world of video, audio, images, and documents.
Whether you're a media company managing thousands of assets, an e-commerce platform processing product imagery, or a content platform handling user-generated media, Transloadit's Media ETL approach offers a powerful solution for your media processing needs.
Ready to reimagine your media workflows with the power of ETL principles? Get started with Transloadit today.