Launching official Go SDK for enhanced integration
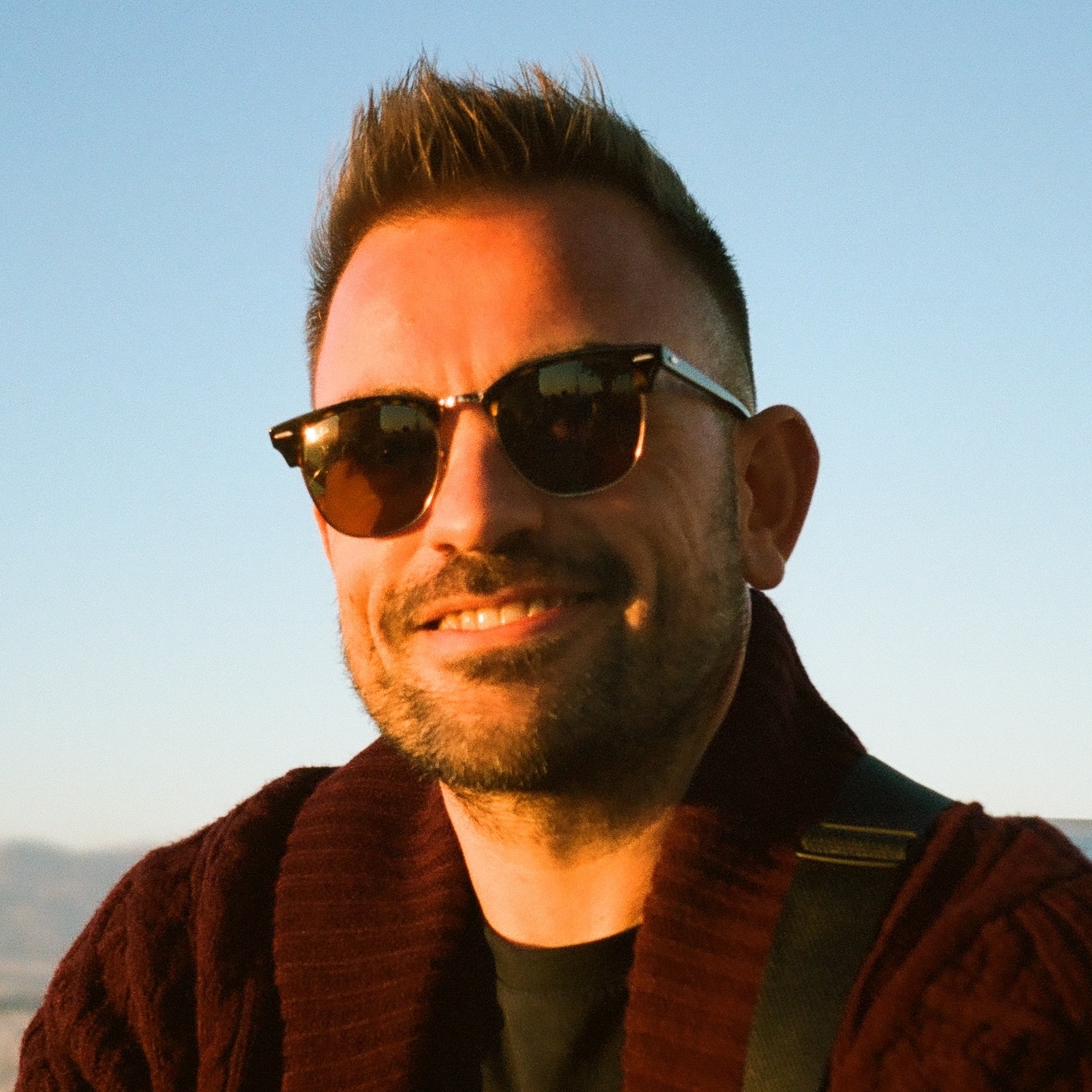
Today, our team is excited to announce the launch of the official Transloadit Go SDK. If you are not familiar with Go, it is a really powerful open source language that is growing fast. Even though our API is written in Node.js, we have been happily using Go internally for scaling and system utilities. We are, therefore, always looking for more ways to leverage the power of this fun language.
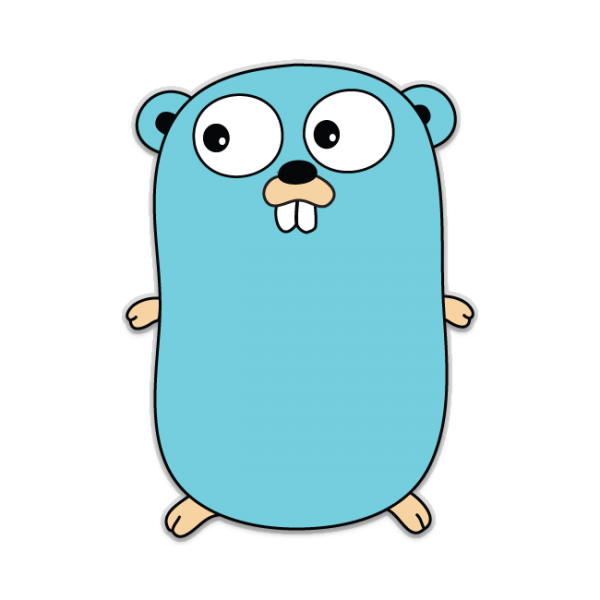
While it was already possible to talk to our REST API using any language, we are hoping that this release will make it easier than ever for developers with an interest in Go to integrate our file import & encoding services into their own projects.
The code and docs are up on GitHub and
Godoc, but we would also like to give you an idea
of how our SDK could be used to resize a lol_cat.jpg
image to 75×75 pixels.
After a quick go get github.com/transloadit/go-sdk
, this is what the integration code could look
like:
import "github.com/transloadit/go-sdk"
import "fmt"
// Create client
options := transloadit.DefaultConfig
options.AuthKey = "YOUR_TRANSLOADIT_KEY"
options.AuthSecret = "YOUR_TRANSLOADIT_SECRET"
client, err := transloadit.NewClient(options)
if err != nil {
panic(err)
}
// Initialize new assembly
assembly := client.CreateAssembly()
// Add file to upload
err = assembly.AddFile("./lol_cat.jpg")
if err != nil {
panic(err)
}
// Add Instructions, e.g. resize image to 75x75px
assembly.AddStep("resize", map[string]interface{}{
"robot": "/image/resize",
"width": 75,
"height": 75,
"resize_strategy": "crop",
})
// Wait until Transloadit is done processing all uploads
// and is ready for you to download the results
assembly.Blocking = true
// Start the upload
info, err := assembly.Upload()
if err != nil {
panic(err)
}
fmt.Printf("You can view the result at: %s\n", info.Results["resize"][0].Url)
While this may seem like a lot of trouble to simply resize an image, it should be noted that this same snippet could also be used to optimize video for tablets, convert to HLS in order to prepare your app's videos for submission to the App Store, generate animated gif previews of documents, and to work with all other cool conversions that Transloadit's Robots can do for you.
As a bonus, this SDK ships with a built-in feature that allows you to automatically create
Assemblies from a local directory. It will not only upload all files to Transloadit and
convert them using the Steps you supplied, but also immediately download all the
results.
In addition to that, this function can monitor a directory for changes and automatically process
them as they occur. Let's have a look at the code needed for that:
options := &transloadit.WatchOptions{
// Use Encoding instructions as stored in a Template in your Transloadit Account
TemplateId: "02a8693053cd11e49b9ba916b58830db",
// The directory which the files will be taken from
Input: "./input",
// The directory where the results are downloaded into
Output: "./output",
// Watch the input directory for changes
Watch: true,
// Put the original files from the input directory
// into the output directory (recommended as the input dir gets cleared)
Preserve: true,
}
watcher := client.Watch(options)
Now you can just drop files in the ./input
directory and the converted results will then be saved
in ./output
. Pretty convenient, right? 😄
To a degree, yes. But not yet as convenient as we would like, because you will still have to write a few lines of code to profit from this.
That is why we have collaborated with the young and gifted Acconut to not only build this SDK, but also build a CLI tool based on this SDK that lets you use Transloadit on your own servers / workstations without writing a single line of code. Stay tuned for a separate blog post that will give this tool the attention that it deserves!
If you have any feedback, we would love to hear from you either in the project's GitHub issues, the comments section here, our in our inbox over at mailto:hello@transloadit.com.
Lastly, we would like to give a shout-out to our emeritus Felix who did a thorough review on this project. 😄