Releasing our newly rewritten Android SDK
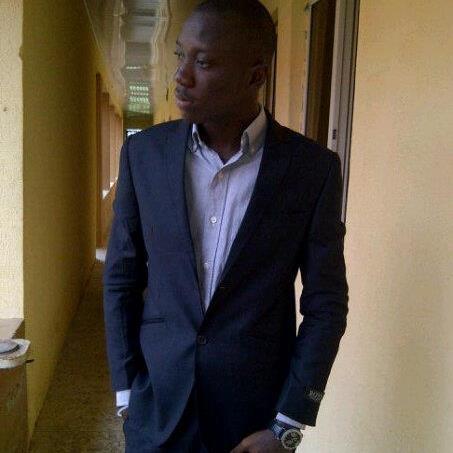
Although the Java SDK could already be used for Android projects, and we also had a previously written Android integration (which has since been deprecated), we are excited to announce the release of our newly rewritten Android SDK. This latest devkit offers an API that is more consistent with the rest of our SDKs.
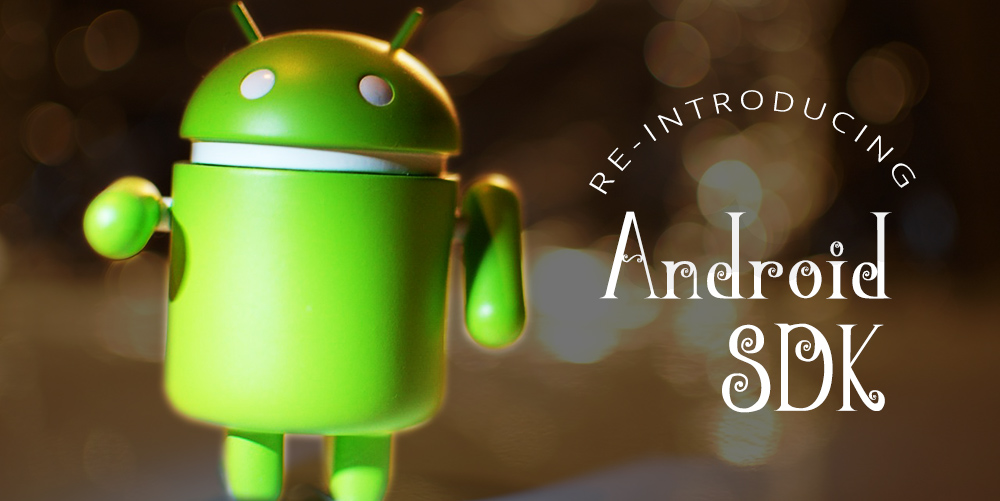
Since this SDK is an extension of our Java SDK, it automatically inherits all of its features. And in addition to that, it supports background(asynchronous) assembly submission with the ability to pause and resume uploads. Sweet, isn't it?
Here are a few quick steps to help you get started:
1. Install the SDK by downloading it directly from our Maven and Jcenter repositories.
Gradle:
compile 'com.transloadit.android.sdk:transloadit-android:0.0.2'
Maven:
<dependency>
<groupId>com.transloadit.android.sdk</groupId>
<artifactId>transloadit-android</artifactId>
<version>0.0.2</version>
<type>pom</type>
</dependency>
2. We'll walk you through the creation of a background Assembly. To get started,
first we create a MyAssemblyListener
class to serve as our callback. This class will listen to the
state of our background Assembly from the point of submission until it has finished
executing.
import com.transloadit.sdk.async.AssemblyProgressListener;
import com.transloadit.sdk.response.AssemblyResponse;
// to create a listener, we must implement the AssemblyProgressListener interface
public class MyAssemblyListener implements AssemblyProgressListener {
@Override
public void onUploadPogress(long uploadedBytes, long totalBytes) {
// as the files of your new assembly get uploaded, this callback
// gets called on every upload progress received
System.out.println("uploaded " + uploadedBytes + " of " + totalBytes);
}
@Override
public void onUploadFinished() {
// when all your assmebly files are uploaded, this callback gets called
System.out.println("all file uploads completed");
}
@Override
public void onUploadFailed(Exception exception) {
// An error occured during file upload
exception.printStackTrace();
}
@Override
public void onAssemblyFinished(AssemblyResponse response) {
// the assembly is done executing
System.out.println("Our assembly is done with the status " + response.json().getString("ok"));
}
@Override
public void onAssemblyStatusUpdateFailed(Exception exception) {
// background execution failed while trying to fetch the
// status of the assembly.
exception.printStackTrace();
}
}
3. Create a new activity from which to start the Assembly. In this example, we will create an Assembly to resize an image:
import com.transloadit.android.sdk.AndroidAsyncAssembly;
import com.transloadit.android.sdk.AndroidTransloadit;
import com.transloadit.sdk.exceptions.LocalOperationException;
import com.transloadit.sdk.exceptions.RequestException;
import java.io.File;
import java.util.HashMap;
import java.util.Map;
public class AssemblyActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
AndroidTransloadit transloadit = new AndroidTransloadit("key", "secret");
// pass an instance of our MyAssemblyListener class as argument.
AndroidAsyncAssembly androidAsyncAssembly = transloadit.newAssembly(
new MyAssemblyListener(),
this
);
File image = new File("path/to/image.jpg");
assembly.addFile(image);
// add instructions resize the image
Map<String, Object> stepOptions = new HashMap<String, Object>();
stepOptions.put("width", 75);
stepOptions.put("height", 75);
stepOptions.put("resize_strategy", "pad");
assembly.addStep("resize", "/image/resize", stepOptions);
}
}
4. Submit the Assembly to Transloadit by calling the save
method. Our updated
AssemblyActivity
class should now look like this:
import com.transloadit.android.sdk.AndroidAsyncAssembly;
import com.transloadit.android.sdk.AndroidTransloadit;
import com.transloadit.sdk.exceptions.LocalOperationException;
import com.transloadit.sdk.exceptions.RequestException;
import java.io.File;
import java.util.HashMap;
import java.util.Map;
public class AssemblyActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
AndroidTransloadit transloadit = new AndroidTransloadit("key", "secret");
// pass an instance of our MyAssemblyListener class as argument.
AndroidAsyncAssembly androidAsyncAssembly = transloadit.newAssembly(
new MyAssemblyListener(),
this
);
File image = new File("path/to/image.jpg");
assembly.addFile(image);
// add instructions resize the image
Map<String, Object> stepOptions = new HashMap<String, Object>();
stepOptions.put("width", 75);
stepOptions.put("height", 75);
stepOptions.put("resize_strategy", "pad");
assembly.addStep("resize", "/image/resize", stepOptions);
try {
assembly.save();
} catch (RequestException | LocalOperationException e) {
e.printStackTrace();
}
// continue with other operations while assembly runs in the background
}
}
And that's all you need to do! For every status update on the Assembly, your defined listener will be called accordingly.
For more detailed documentation and examples, please take a look at:
- Examples: https://github.com/transloadit/android-sdk/tree/master/examples
- Source Code: https://github.com/transloadit/android-sdk
- Documentation: https://javadoc.io/doc/com.transloadit.android.sdk/transloadit-android/0.0.2
We hope you'll have a better experience with this new release! 😄