Automate PDF form filling with Java and PDFtk
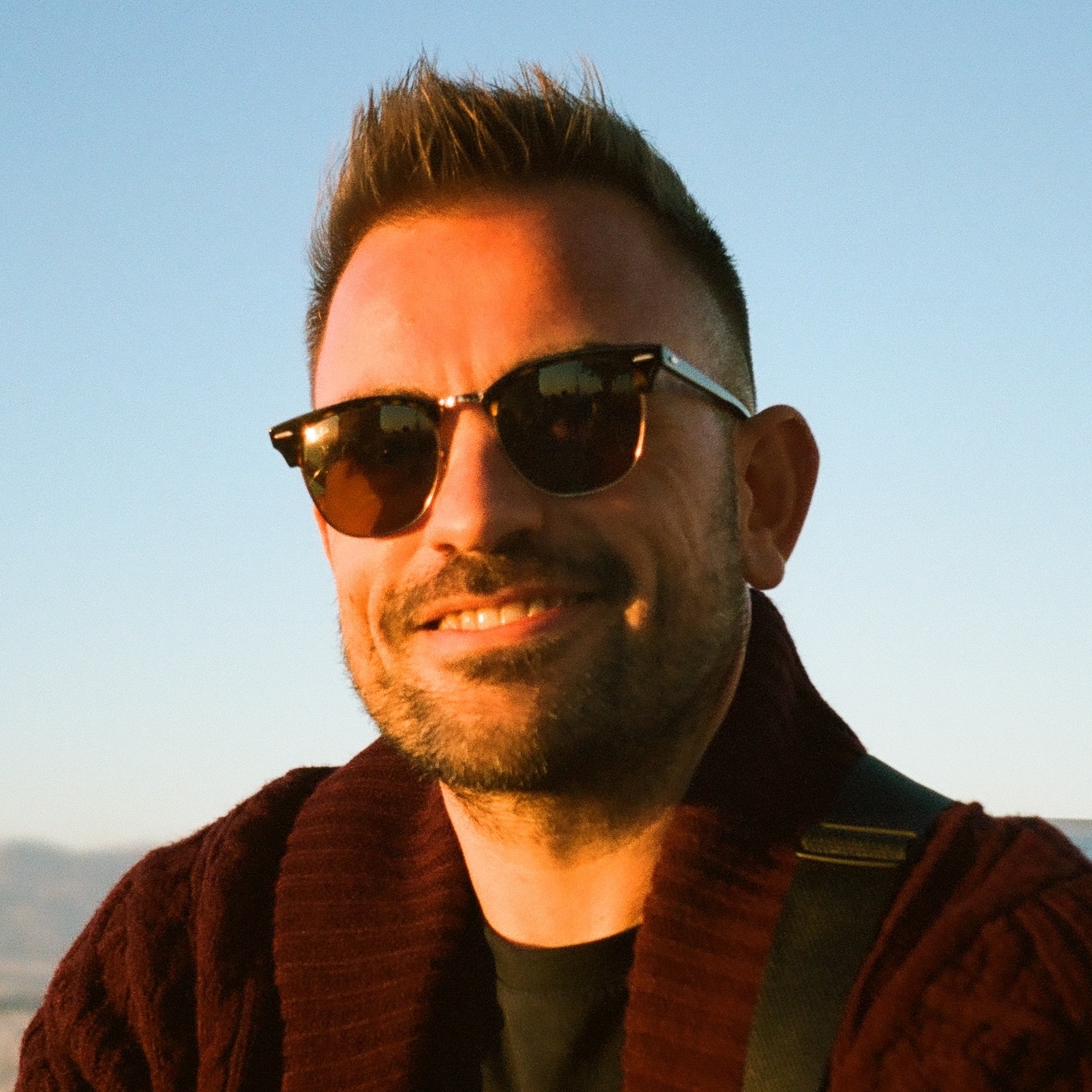
Filling out PDF forms manually can be tedious and error-prone, especially when dealing with repetitive tasks like tax forms, invoices, or character sheets. Thankfully, you can automate this process using Java and the command-line tool PDFtk (PDF Toolkit), significantly streamlining your document workflows.
Introduction to PDFtk and Java integration
PDFtk is a powerful, cross-platform command-line tool for manipulating PDF documents. It allows you to merge, split, encrypt, decrypt, and, importantly for this guide, fill PDF forms programmatically. By integrating PDFtk with Java, you can build robust applications that automate these PDF manipulation tasks efficiently.
Prerequisites
Before getting started, ensure you have:
- Java Runtime Environment (JRE) or Java Development Kit (JDK) 8 or higher installed
- PDFtk installed on your system (see installation steps below)
- Basic knowledge of Java programming
- A PDF form with fillable fields (e.g.,
template.pdf
)
Setting up PDFtk in your Java environment
First, install PDFtk on your system.
For Ubuntu/Debian:
sudo apt-get update
sudo apt-get install pdftk-java
For macOS (using Homebrew):
brew install pdftk
For Windows, download the installer from the PDFtk website.
Verify PDFtk is accessible from your command line and check its version:
pdftk --version
This command should output the installed version details. If it fails, check your installation and system PATH.
Next, set up your Java project. We'll use Java's built-in ProcessBuilder
to interact with the
PDFtk command-line tool from our code.
Extracting form data fields from PDFs using PDFtk
Before filling a form, you need to know the names of its fillable fields. You can use PDFtk to extract this information:
pdftk template.pdf dump_data_fields
Replace template.pdf
with the path to your PDF form. This command outputs details about each
field, including its name (FieldName
), type (FieldType
), and possible options
(FieldStateOption
for checkboxes/radio buttons). The output will look something like this:
---
FieldType: Text
FieldName: Name
FieldFlags: 0
FieldJustification: Left
---
FieldType: Text
FieldName: Date
FieldFlags: 0
FieldJustification: Left
---
FieldType: Button
FieldName: SubscribeNewsletter
FieldFlags: 0
FieldStateOption: Yes
FieldStateOption: Off
---
Note the FieldName
values; you'll use these as keys in your Java code.
Automating form filling with Java
Here's a practical Java example demonstrating how to automate PDF form filling. This code generates a temporary FDF (Forms Data Format) file, uses PDFtk to merge the data with the template PDF, and includes proper error handling and resource cleanup.
import java.io.*;
import java.util.*;
public class PdfFormFiller {
/**
* Fills a PDF form template with provided data using PDFtk.
*
* @param templatePdf Path to the template PDF form.
* @param outputPdf Path where the filled PDF will be saved.
* @param formData A Map where keys are PDF field names and values are the data to fill in.
* @throws IOException If an I/O error occurs during file handling or process execution.
* @throws InterruptedException If the PDFtk process is interrupted.
*/
public static void fillForm(String templatePdf, String outputPdf, Map<String, String> formData) throws IOException, InterruptedException {
File tempFdf = null;
try {
// Create a temporary FDF file
tempFdf = File.createTempFile("form_data_", ".fdf");
// Write form data to the FDF file
try (PrintWriter writer = new PrintWriter(new FileWriter(tempFdf))) {
writer.println("%FDF-1.2");
writer.println("1 0 obj << /FDF << /Fields [");
formData.forEach((key, value) -> {
// Basic escaping for special characters in values (parentheses, backslash)
String escapedValue = value.replace("\\", "\\\\").replace("(", "\\(").replace(")", "\\)");
writer.printf("<< /T (%s) /V (%s) >>\n", key, escapedValue);
});
writer.println("] >> >> endobj");
writer.println("trailer << /Root 1 0 R >>");
writer.println("%%EOF");
}
// Prepare and execute the PDFtk command
ProcessBuilder pb = new ProcessBuilder(
"pdftk",
templatePdf,
"fill_form", tempFdf.getAbsolutePath(),
"output", outputPdf,
"flatten" // Optional: Makes the filled form non-editable
);
Process process = pb.start();
// Capture standard error stream for troubleshooting
StringBuilder errorOutput = new StringBuilder();
try (BufferedReader reader = new BufferedReader(new InputStreamReader(process.getErrorStream()))) {
String line;
while ((line = reader.readLine()) != null) {
errorOutput.append(line).append("\n"); // Use escaped newline for JSON compatibility
}
}
// Wait for the process to complete and check the exit code
int exitCode = process.waitFor();
if (exitCode != 0) {
throw new IOException("PDFtk process failed with exit code " + exitCode + ". Error output:\n" + errorOutput.toString());
}
} finally {
// Ensure the temporary FDF file is deleted
if (tempFdf != null && tempFdf.exists()) {
if (!tempFdf.delete()) {
System.err.println("Warning: Failed to delete temporary FDF file: " + tempFdf.getAbsolutePath());
}
}
}
}
public static void main(String[] args) {
try {
// Example data - replace with your actual field names and values
Map<String, String> data = new HashMap<>();
data.put("Name", "Jane Doe");
data.put("Date", "2025-04-01");
// Add more fields as needed based on dump_data_fields output
// data.put("Address", "456 Oak Avenue");
// data.put("SubscribeNewsletter", "Yes"); // For checkboxes
String templateFile = "template.pdf"; // Path to your template PDF
String outputFile = "filled_form.pdf"; // Path for the output PDF
fillForm(templateFile, outputFile, data);
System.out.println("Form '" + outputFile + "' filled successfully!");
} catch (IOException | InterruptedException e) {
System.err.println("Error filling PDF form: " + e.getMessage());
// Consider more specific error handling or logging
}
}
}
This script:
- Takes the template PDF path, output PDF path, and a
Map
of form data as input. - Creates a temporary FDF file to hold the form data.
- Writes the data into the FDF file in the required format.
- Uses
ProcessBuilder
to execute thepdftk
command, passing the template PDF, the temporary FDF file, and the desired output path. Theflatten
option makes the output PDF non-editable, embedding the form data directly. - Captures any error output from the PDFtk process for better debugging.
- Checks the exit code of the PDFtk process; a non-zero code indicates an error.
- Crucially, uses a
finally
block to ensure the temporary FDF file is deleted, even if errors occur.
Practical examples: automating tax forms and character sheets
This technique is useful in various scenarios:
- Tax Forms: Automate filling repetitive fields like name, address, and tax identification numbers across multiple forms.
Map<String, String> taxFormData = new HashMap<>();
taxFormData.put("FullName", "John Doe");
taxFormData.put("SSN", "123-45-6789"); // Ensure secure handling of sensitive data
taxFormData.put("Address", "123 Main Street");
taxFormData.put("City", "Anytown");
taxFormData.put("State", "CA");
taxFormData.put("ZipCode", "12345");
taxFormData.put("FilingStatus", "Single"); // Use exact value expected by the form field
// fillForm("tax_form_1040.pdf", "filled_tax_form_johndoe.pdf", taxFormData);
- Character Sheets: Quickly generate filled character sheets for tabletop RPGs based on player data.
Map<String, String> characterData = new HashMap<>();
characterData.put("CharacterName", "Elara Meadowlight");
characterData.put("Class", "Ranger");
characterData.put("Level", "5");
characterData.put("Strength", "12");
characterData.put("Dexterity", "18");
characterData.put("Constitution", "14");
characterData.put("Intelligence", "10");
characterData.put("Wisdom", "16");
characterData.put("Charisma", "13");
// fillForm("dnd_character_sheet.pdf", "elara_character.pdf", characterData);
Advanced tips for handling complex PDF forms
PDF forms can contain various field types beyond simple text boxes. Use the output from
dump_data_fields
to understand how to populate them:
- Checkboxes: Typically expect
Yes
for checked andOff
for unchecked. Check theFieldStateOption
values from the dump.data.put("SubscribeNewsletter", "Yes"); data.put("AgreeToTerms", "Yes");
- Radio Buttons: These usually belong to a group with the same
FieldName
. Set the field to the specificFieldStateOption
value corresponding to the desired choice.// Assuming 'Gender' is the FieldName and 'Male'/'Female'/'Other' are FieldStateOptions data.put("Gender", "Female");
- Dropdowns (Choice Fields): Set the
FieldName
to the exact text of the desired option as it appears in the dropdown list.data.put("Country", "Canada");
Troubleshooting common issues
PDFtk not found
If your Java application throws an error like
"Cannot run program 'pdftk': error=2, No such file or directory"
or similar:
- Verify Installation: Open your terminal or command prompt and run
pdftk --version
. If this fails, PDFtk is not installed correctly or not in your system's PATH. Revisit the installation steps. - Check PATH: Ensure the directory containing the
pdftk
executable is included in your system's PATH environment variable. How to do this varies by operating system. - Use Absolute Path: As a workaround, you can specify the full path to the
pdftk
executable in your Java code, although this makes the code less portable:// Example for Linux/macOS, adjust the path as needed for your system ProcessBuilder pb = new ProcessBuilder("/usr/local/bin/pdftk", templatePdf, "fill_form", ...);
Permission issues
If you encounter errors related to file access (e.g., "Permission denied"):
- File Permissions: Ensure your Java application has read permissions for the template PDF
(
template.pdf
) and write permissions for the output directory wherefilled_form.pdf
will be created. - Execution Permissions: Ensure the
pdftk
executable itself has execute permissions (usually handled by the installer, but worth checking on Linux/macOS).
Handling encrypted PDFs
If your template PDF is password-protected, PDFtk needs the password to open it. Modify the
ProcessBuilder
command to include the input_pw
option:
// Add "input_pw" and the password before "fill_form"
ProcessBuilder pb = new ProcessBuilder(
"pdftk",
templatePdf,
"input_pw", "your_pdf_password", // Add this line
"fill_form", tempFdf.getAbsolutePath(),
"output", outputPdf,
"flatten"
);
Replace "your_pdf_password"
with the actual password. Remember to handle passwords securely in
your application and avoid hardcoding them directly in the source code for production systems.
Incorrect field names or values
If the form doesn't fill as expected, or some fields remain blank:
- Verify Field Names: Double-check that the keys in your
formData
map exactly match theFieldName
values from thedump_data_fields
output. Field names are case-sensitive. - Check Field Types/Values: Ensure the values you provide are appropriate for the field type
(e.g.,
Yes
/Off
for checkboxes, specific export values for radio buttons, correct option text for dropdowns). Refer to thedump_data_fields
output. - Special Characters: Ensure values containing special characters like parentheses
()
or backslashes\
are properly escaped when writing the FDF file, as shown in the example code.
Conclusion
Automating PDF form filling with Java and PDFtk saves time, reduces errors, and enhances productivity. Whether you're handling invoices, contracts, reports, or even gaming character sheets, this approach can significantly streamline your document processing workflow, especially for repetitive tasks involving structured data.
While PDFtk is great for command-line manipulation on a local machine or server, cloud-based workflows often require different tools. For instance, Transloadit offers the 🤖 /document/merge Robot for concatenating PDF documents, utilizing technology optimized for cloud operations rather than relying on PDFtk directly. Explore our Document Processing service to see how Transloadit can further enhance your document workflows in the cloud.