Content-aware resizing with PHP & ImageMagick
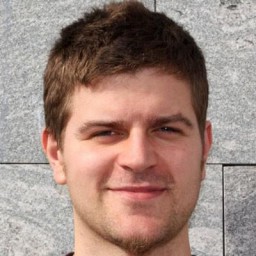
In this post, we explore a powerful technique for resizing images in a content-aware manner using PHP and ImageMagick. By leveraging the liquid rescale feature available via the Imagick extension, you can intelligently adjust image dimensions while preserving key details.
What is content-aware resizing?
Content-aware resizing—often referred to as seam carving—allows you to adjust image dimensions by removing or inserting pixels in areas with less important visual information, rather than uniformly scaling the entire image. Unlike traditional resizing methods, this approach minimizes distortion of the subject in your images.
Prerequisites
Before getting started, ensure you have the following:
- PHP 7.4 or later (tested up to PHP 8.3)
- The Imagick extension enabled in your PHP installation
- ImageMagick version 6.5.3-10 or later (both version 6 and 7 are supported)
Additional prerequisites
For content-aware resizing functionality:
- liblqr library must be installed (package
liblqr-1-0
on Debian/Ubuntu) - ImageMagick must be compiled with liblqr support
How to use liquid rescale in PHP
ImageMagick provides the liquidRescaleImage
method, which performs content-aware resizing. Here's
how to implement it:
<?php
try {
// Create a new Imagick object from an input image
$image = new Imagick('input.jpg');
// Define your target dimensions
$targetWidth = 400;
$targetHeight = 300;
// Parameters for liquid rescale
$deltaX = 1; // Controls horizontal pixel movement (1-100)
$rigidity = 0; // Determines resistance to pixel adjustment (0-100)
// Perform content-aware resizing
$image->liquidRescaleImage($targetWidth, $targetHeight, $deltaX, $rigidity);
// Write the result
$image->writeImage('output.jpg');
$image->destroy();
} catch (ImagickException $e) {
echo "Error: " . $e->getMessage();
}
Parameter fine-tuning
The liquid rescale operation is controlled by two key parameters:
- deltaX (1-100): Controls horizontal pixel movement
- Lower values (1-10) enable more aggressive seam removal
- Higher values preserve original image structure
- rigidity (0-100): Determines resistance to pixel adjustment
- 0 allows maximum flexibility for content-aware resizing
- Higher values protect important features from distortion
Performance considerations
Content-aware resizing can be resource-intensive, especially for large images. Here are some optimization strategies:
- Process images in smaller sections for memory efficiency
- Implement queue systems for batch processing
- Combine standard resizing with liquid rescale:
try {
$image = new Imagick('input.jpg');
// Initial standard resize
$image->resizeImage(800, 600, Imagick::FILTER_LANCZOS, 1);
// Follow with content-aware adjustment
$image->liquidRescaleImage(400, 300, 1, 0);
$image->writeImage('optimized-output.jpg');
$image->destroy();
} catch (ImagickException $e) {
echo "Error: " . $e->getMessage();
}
Common issues and solutions
Memory allocation errors
ini_set('memory_limit', '512M'); // Adjust based on image size
Missing liblqr support
Verify installation with:
convert -list configure | grep LIBLQR
If no output appears, reinstall ImageMagick with liblqr support.
Quality preservation
For significant size reductions:
- Apply multiple gradual resizing operations
- Combine with sharpening filters after resizing
- Maintain original aspect ratio when possible
Wrapping up
Content-aware resizing enables you to maintain image integrity while adjusting dimensions to focus on important areas. By combining PHP with ImageMagick's liquid rescale functionality, you can create adaptive image processing solutions that respond to visual content.
For enterprise-scale image processing needs, consider Transloadit's Image Manipulation Service which handles complex workflows automatically.
Happy coding!