Crafting a seamless upload experience with Uppy and xhr
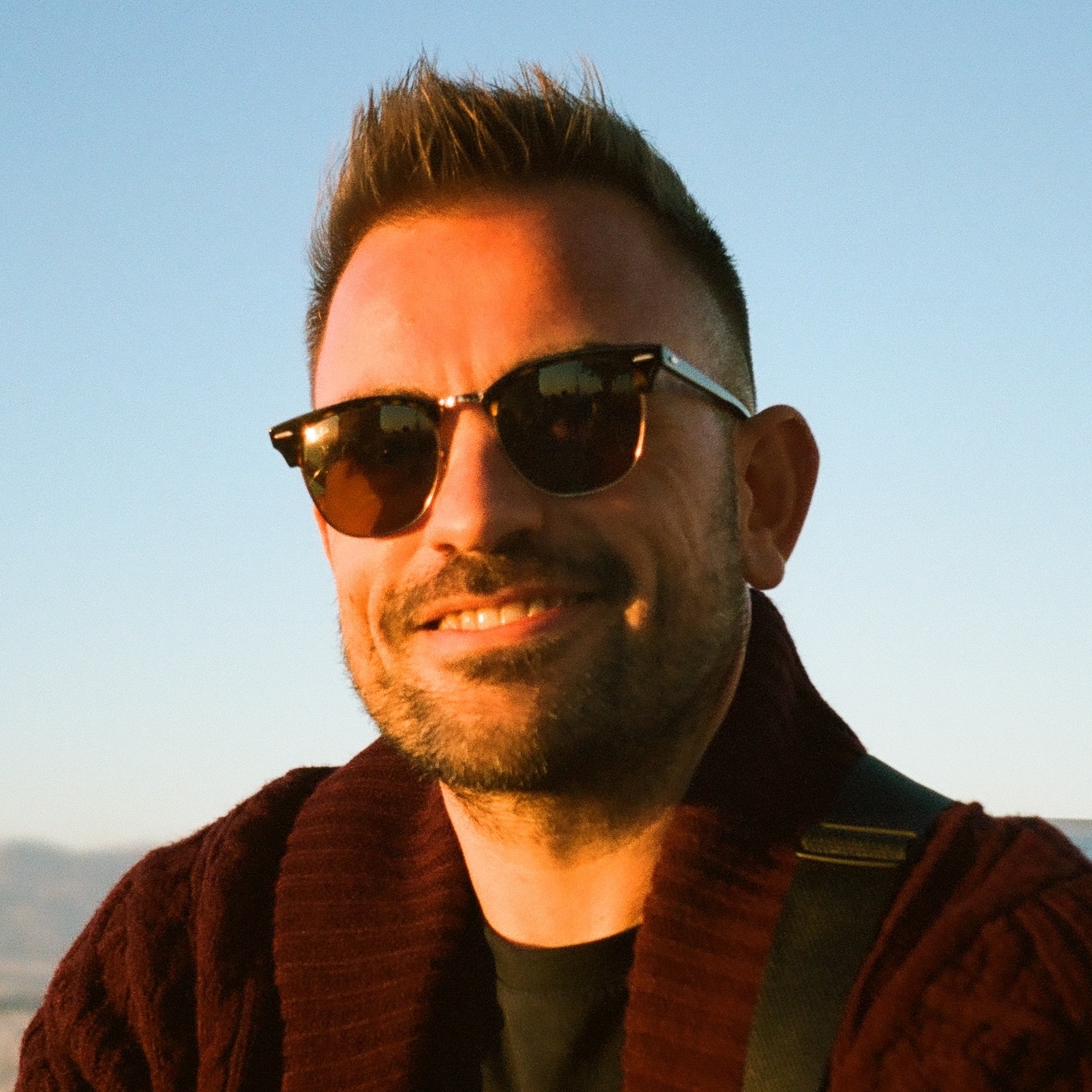
Enhancing file uploads in web applications can significantly improve user experience and performance. In this DevTip, we'll explore how to integrate Uppy with XMLHttpRequest to create an efficient and seamless file upload process in the browser.
Introduction
Handling file uploads in web applications is a common yet challenging task. Developers often seek efficient and user-friendly solutions to manage file uploads while ensuring security and performance. Uppy is a lightweight, modular JavaScript file uploader that simplifies this process.
In this guide, we'll demonstrate how to set up Uppy in a browser environment and integrate it with XMLHttpRequest to handle browser uploads efficiently.
What tools are popular for handling browser uploads?
When it comes to handling file uploads in browsers, Uppy stands out due to its modularity and ease of use. It provides a sleek user interface and supports various plugins and customizations, making it a versatile tool for managing file uploads.
Why use Uppy?
Uppy offers features like drag-and-drop functionality, progress indicators, and supports resumable uploads via the tus protocol. Its extensible architecture allows developers to customize the uploader to meet specific requirements, enhancing the file upload process.
Setting up Uppy in a browser environment
First, let's set up Uppy in your project. You can install Uppy via npm:
npm install @uppy/core @uppy/dashboard
Include the necessary CSS and JavaScript in your HTML:
<!-- Include Uppy CSS -->
<link href="https://releases.transloadit.com/uppy/v2.11.3/uppy.min.css" rel="stylesheet" />
<!-- Include Uppy JavaScript -->
<script src="https://releases.transloadit.com/uppy/v2.11.3/uppy.min.js"></script>
Alternatively, you can include Uppy via CDN as shown above.
Creating xmlhttprequest for handling file data
While Uppy provides built-in upload methods, integrating it with XMLHttpRequest gives you greater control over the upload process. XMLHttpRequest allows you to monitor the progress of uploads and handle them asynchronously, enhancing upload efficiency.
Implementing the Uppy instance
Here's how you can set up Uppy:
const uppy = Uppy.Core().use(Uppy.Dashboard, {
inline: true,
target: '#drag-drop-area',
})
This initializes Uppy with the Dashboard plugin, which provides a user interface for file selection.
Packaging Uppy and xhr together for better performance
To upload files using XMLHttpRequest, we'll add an event listener that triggers when files are added and when the upload button is clicked.
uppy.on('file-added', (file) => {
console.log(`Added file: ${file.name}`)
})
document.getElementById('upload-button').addEventListener('click', () => {
uppy.getFiles().forEach((file) => {
uploadFile(file)
})
})
Upload function with xmlhttprequest
Now, let's define the uploadFile
function using XMLHttpRequest:
function uploadFile(file) {
const xhr = new XMLHttpRequest()
xhr.open('POST', '/upload', true)
// Monitor upload progress
xhr.upload.onprogress = (event) => {
if (event.lengthComputable) {
const percentage = (event.loaded / event.total) * 100
console.log(`Upload progress: ${percentage.toFixed(2)}%`)
}
}
xhr.onload = () => {
if (xhr.status === 200) {
console.log(`File uploaded successfully: ${file.name}`)
} else {
console.error(`Error uploading file: ${file.name}`)
}
}
xhr.onerror = () => {
console.error('Error uploading file.')
}
const formData = new FormData()
formData.append('file', file.data)
xhr.send(formData)
}
This function creates a new XMLHttpRequest, sets up event handlers to monitor the upload progress, and sends the file data to the server.
Implementing a basic upload feature
In your HTML, include a container for the Uppy Dashboard and an upload button:
<div id="drag-drop-area"></div>
<button id="upload-button">Upload Files</button>
With this setup, users can select files using the Uppy Dashboard, and clicking the upload button will initiate the file uploads using XMLHttpRequest.
How does Uppy enhance file upload processes?
Uppy simplifies the file upload process by providing an intuitive interface and handling complex tasks behind the scenes. It manages file selection, handles large files efficiently, and provides progress indicators, improving the overall user experience.
What is the role of xmlhttprequest in file uploads?
XMLHttpRequest allows you to send HTTP requests directly from JavaScript, enabling asynchronous file uploads without requiring a page reload. It provides detailed progress information, which can be used to update progress bars and inform users about the upload status.
Best practices for secure and efficient uploads
- Validate Files on the Server: Always perform server-side validation of uploaded files to ensure security.
- Implement Authentication: Secure your upload endpoints to prevent unauthorized access.
- Limit File Sizes: Implement file size limits to prevent excessively large uploads.
- Provide User Feedback: Use Uppy's progress indicators to inform users about the upload status.
- Handle Errors Gracefully: Provide clear error messages and allow users to retry failed uploads.
Troubleshooting common issues
- CORS Errors: Ensure your server has the correct CORS headers if the frontend and backend are on different domains.
- Network Errors: Implement retry logic or resumable uploads for unreliable network conditions.
- File Compatibility: Verify that the server correctly handles different file types and encodings.
Conclusion
Integrating Uppy with XMLHttpRequest allows for a customizable and efficient browser upload experience in web applications. By leveraging these tools, developers can enhance user experience while maintaining control over the upload process.
For advanced file processing and storage solutions, consider exploring Transloadit's Handling Uploads service and Uppy.