Custom HTML file upload button with drag-and-drop
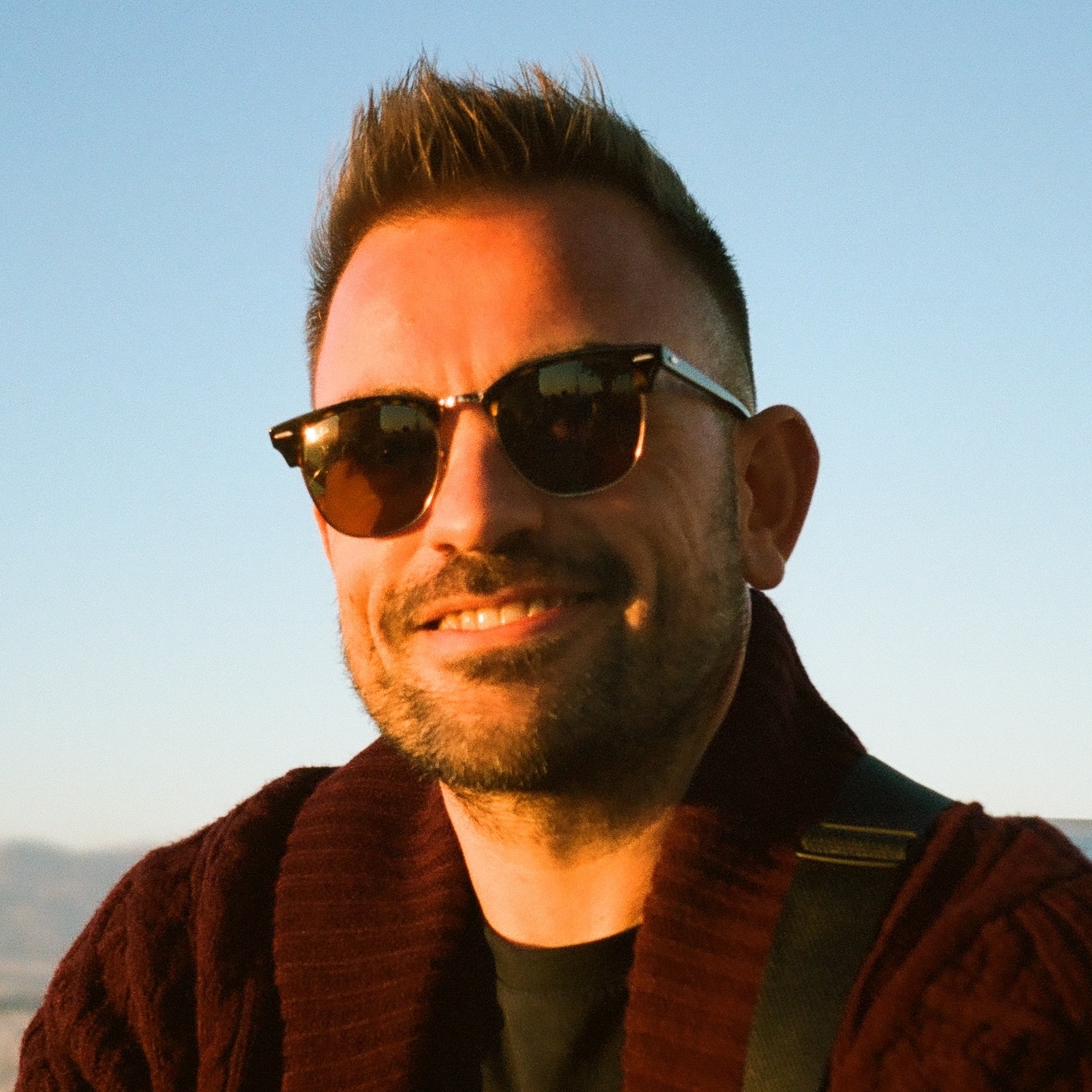
File uploads are essential for many web applications, but the default HTML file input often falls short in terms of user experience. In this guide, we'll show you how to create a custom HTML file upload button with drag-and-drop functionality, enhancing your web application's interface and making file uploads more intuitive for users.
Let's explore how to create a custom file upload button in HTML with drag-and-drop capabilities.
Introduction to HTML file upload buttons
The standard HTML <input type="file">
element allows users to select files from their device for
uploading. However, it doesn't offer much in terms of customization or user experience. By creating
a custom file upload button and incorporating drag-and-drop functionality, you can provide a more
engaging and user-friendly interface.
Basic HTML file upload setup
Let's start with a simple HTML file upload form:
<form id="upload-form" action="/upload" method="post" enctype="multipart/form-data">
<input type="file" id="file-input" name="file" />
<button type="submit">Upload</button>
</form>
This form creates a file input and an upload button. While functional, it's not visually appealing and lacks modern UX features.
Creating a custom file upload button in HTML
To create a custom file upload button in HTML, we'll hide the default file input and use a label element that triggers the file selection dialog when clicked.
Here's how you can modify your form:
<form id="upload-form" action="/upload" method="post" enctype="multipart/form-data">
<input type="file" id="file-input" name="file" />
<label for="file-input" id="file-input-label">Choose a file</label>
<button type="submit">Upload</button>
</form>
In this code:
- The
input
element is hidden using CSS. - A
label
is associated with theinput
via thefor
attribute. - Clicking the label activates the hidden file input.
Now, let's style the custom button to improve its appearance.
Styling the custom file upload button with CSS
Add the following CSS to style the label as a button:
#file-input {
display: none;
}
#file-input-label {
display: inline-block;
padding: 10px 20px;
background-color: #007bff;
color: #fff;
cursor: pointer;
border-radius: 4px;
font-size: 16px;
}
#file-input-label:hover {
background-color: #0056b3;
}
This CSS:
- Hides the default file input.
- Styles the label to look like a button.
- Adds hover effects for better user interaction.
Adding drag-and-drop file upload functionality in HTML
Enhancing the file upload form with drag-and-drop functionality makes it even more user-friendly. Here's how to implement it:
Updating the HTML
Add a drop area to your HTML:
<div id="drop-area">
<p>Drag & drop files here</p>
<p>or</p>
<label for="file-input" id="file-input-label">Choose a file</label>
</div>
<form id="upload-form" action="/upload" method="post" enctype="multipart/form-data">
<input type="file" id="file-input" name="file" />
<button type="submit">Upload</button>
</form>
Styling the drop area with CSS
#drop-area {
border: 2px dashed #007bff;
border-radius: 4px;
padding: 20px;
text-align: center;
}
#drop-area.highlight {
background-color: #e9ecef;
}
This CSS:
- Defines the appearance of the drop area.
- Provides visual feedback when files are dragged over the drop area.
Implementing drag-and-drop with JavaScript
Add the following JavaScript to handle drag-and-drop events:
const dropArea = document.getElementById('drop-area')
const fileInput = document.getElementById('file-input')
// Prevent default behaviors
;['dragenter', 'dragover', 'dragleave', 'drop'].forEach((eventName) => {
dropArea.addEventListener(eventName, preventDefaults, false)
})
function preventDefaults(e) {
e.preventDefault()
e.stopPropagation()
}
// Highlight drop area when file is dragged over it
;['dragenter', 'dragover'].forEach((eventName) => {
dropArea.addEventListener(eventName, highlight, false)
})
;['dragleave', 'drop'].forEach((eventName) => {
dropArea.addEventListener(eventName, unhighlight, false)
})
function highlight() {
dropArea.classList.add('highlight')
}
function unhighlight() {
dropArea.classList.remove('highlight')
}
// Handle dropped files
dropArea.addEventListener('drop', handleDrop, false)
function handleDrop(e) {
const dt = e.dataTransfer
const files = dt.files
handleFiles(files)
}
function handleFiles(files) {
// Append files to FormData and send via AJAX
const formData = new FormData()
for (let i = 0; i < files.length; i++) {
formData.append('file', files[i])
}
uploadFiles(formData)
}
function uploadFiles(formData) {
fetch('/upload', {
method: 'POST',
body: formData,
})
.then((response) => response.text())
.then((data) => {
console.log('Success:', data)
})
.catch((error) => {
console.error('Error:', error)
})
}
This JavaScript:
- Prevents default browser behaviors for drag-and-drop events.
- Highlights the drop area when files are dragged over it.
- Handles files dropped into the drop area.
- Sends the files to the server using the Fetch API.
Handling file uploads on the server side
To process the uploaded files on the server, you need to handle the POST request made by the Fetch API. Here's an example using Node.js and Express:
const express = require('express')
const multer = require('multer')
const cors = require('cors')
const app = express()
const upload = multer({ dest: 'uploads/' })
app.use(cors())
app.use(express.static('public'))
app.post('/upload', upload.array('file'), (req, res) => {
if (!req.files || req.files.length === 0) {
return res.status(400).send('No files uploaded.')
}
res.send('Files uploaded successfully!')
})
app.listen(3000, () => {
console.log('Server started on http://localhost:3000')
})
In this code:
- We include
cors
to handle Cross-Origin Resource Sharing if the front-end and back-end are on different domains. - We use
multer
middleware to handlemultipart/form-data
. upload.array('file')
allows multiple files to be uploaded.- The server responds after successfully receiving the files.
Best practices for file upload security
When handling file uploads, it's important to implement security measures to protect your application and users:
- Validate Files on the Server Side: Check the file type, size, and content. Accept only expected file types and sizes.
- Store Uploaded Files Securely: Save files in a directory not accessible from the web to prevent direct access.
- Set a Maximum File Size: Limit the size of uploaded files to prevent denial-of-service (DoS) attacks.
- Sanitize File Names: Rename uploaded files and remove any hazardous characters to prevent directory traversal attacks.
- Use HTTPS: Ensure your application uses HTTPS to encrypt data during transmission.
Conclusion
By creating a custom HTML file upload button with drag-and-drop functionality, you enhance the user experience and make file uploads more intuitive. Implementing these features can significantly improve the usability of your web application.
For a more advanced, cross-browser compatible solution with additional features like progress bars, file previews, and resumable uploads, consider using Uppy, an open-source file uploader for web browsers.
Now you know how to add a file upload button in HTML and upgrade it with drag-and-drop capabilities. Happy coding!