Import files from MinIO in Java
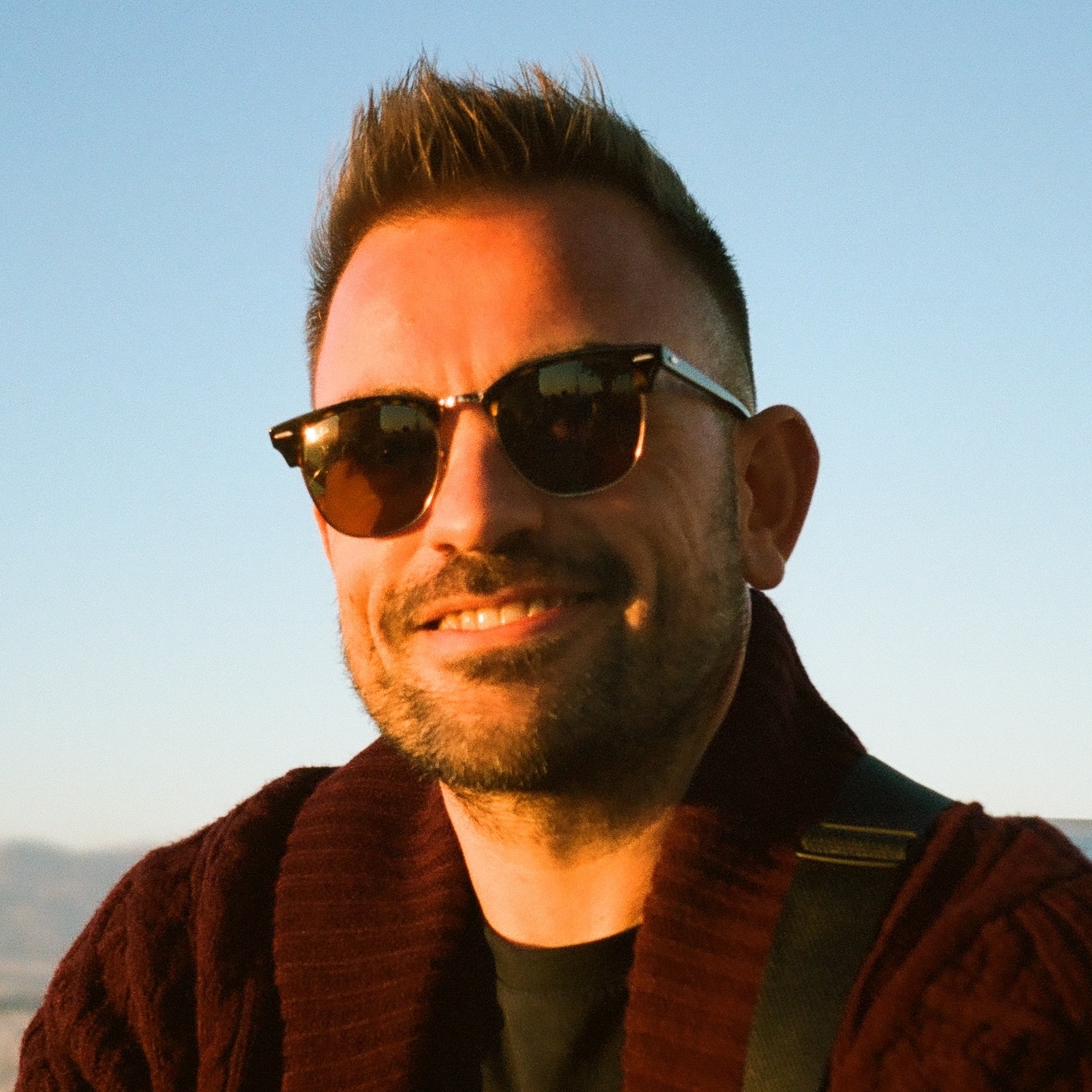
MinIO is a high-performance, distributed object storage system compatible with Amazon S3 APIs. Its simplicity, scalability, and compatibility make it a popular choice for developers looking to integrate robust storage solutions into their Java applications.
In this guide, we'll explore how to efficiently import files from MinIO using Java, leveraging the MinIO Java SDK. We'll cover setup, integration, practical examples, security best practices, and optimization tips.
Introduction to MinIO and S3 compatibility
MinIO provides an open-source, distributed object storage solution designed for high performance and scalability. Its compatibility with Amazon S3 APIs allows developers to easily integrate it into existing workflows and applications that already use S3.
Setting up your Java environment
Before you begin, ensure you have:
- Java 8 or later installed.
- A running MinIO server instance.
- Access credentials (
accessKey
andsecretKey
) for your MinIO server.
Integrating the MinIO Java SDK
To integrate the MinIO Java SDK, add the following dependency to your project:
Maven:
<dependency>
<groupId>io.minio</groupId>
<artifactId>minio</artifactId>
<version>8.5.17</version>
</dependency>
Gradle:
implementation 'io.minio:minio:8.5.17'
Importing files from MinIO: a step-by-step guide
Here's a practical example demonstrating how to import a file from MinIO:
import io.minio.MinioClient;
import io.minio.GetObjectArgs;
import io.minio.errors.MinioException;
import java.io.IOException;
import java.io.InputStream;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
public class MinIOFileImporter {
public static void main(String[] args) {
try {
// Initialize MinIO client
MinioClient minioClient = MinioClient.builder()
.endpoint("play.min.io", 9000, true)
.credentials("Q3AM3UQ867SPQQA43P2F", "zuf+tfteSlswRu7BJ86wekitnifILbZam1KYY3TG")
.build();
// Download file from MinIO
try (InputStream stream = minioClient.getObject(
GetObjectArgs.builder()
.bucket("my-bucket")
.object("path/to/file.txt")
.build())) {
Files.copy(stream, Paths.get("downloaded-file.txt"));
System.out.println("File imported successfully.");
}
} catch (MinioException e) {
System.err.println("Error occurred: " + e);
System.err.println("HTTP trace: " + e.httpTrace());
} catch (IOException e) {
System.err.println("File operation error: " + e);
} catch (NoSuchAlgorithmException | InvalidKeyException e) {
System.err.println("Authentication error: " + e);
} }
}
Replace the endpoint, credentials, bucket name, and object path with your actual MinIO details. Remember to use your own MinIO server and credentials in a production environment.
Handling common exceptions
When working with MinIO, you might encounter several types of exceptions:
MinioException
: Base exception for all MinIO-related errors.ErrorResponseException
: Indicates issues like incorrect bucket names or permissions.InsufficientDataException
: Occurs when the data stream ends prematurely.InternalException
: Represents internal SDK errors.
IOException
: File system related errors.NoSuchAlgorithmException
orInvalidKeyException
: Authentication-related errors.
Always handle exceptions gracefully and log meaningful error messages to simplify debugging.
Security best practices
When working with MinIO in production, follow these security best practices:
Use environment variables
Store credentials in environment variables instead of hardcoding them:
String accessKey = System.getenv("MINIO_ACCESS_KEY");
String secretKey = System.getenv("MINIO_SECRET_KEY");
MinioClient minioClient = MinioClient.builder()
.endpoint("your-minio-server.com")
.credentials(accessKey, secretKey)
.build();
Enable tls
Always use HTTPS in production:
MinioClient minioClient = MinioClient.builder()
.endpoint("your-minio-server.com", 443, true) // true enables HTTPS
.credentials(accessKey, secretKey)
.build();
Implement proper error handling and logging
Log errors appropriately without exposing sensitive information:
try {
// MinIO operations
} catch (MinioException e) {
logger.error("MinIO operation failed: {}", e.getMessage());
logger.debug("Full error details: {}", e); // Only log at debug level
}
Connection management and optimization
To optimize MinIO operations, consider these techniques:
Connection pooling
Reuse the MinioClient
instance across your application:
// Create a singleton MinioClient
public class MinioClientFactory {
private static MinioClient instance;
public static synchronized MinioClient getClient() {
if (instance == null) {
instance = MinioClient.builder()
.endpoint("your-minio-server.com")
.credentials(accessKey, secretKey)
.build();
}
return instance;
}
}
Configure timeouts
Set appropriate connection and read/write timeouts:
OkHttpClient httpClient = new OkHttpClient.Builder()
.connectTimeout(30, TimeUnit.SECONDS)
.writeTimeout(60, TimeUnit.SECONDS)
.readTimeout(60, TimeUnit.SECONDS)
.build();
MinioClient minioClient = MinioClient.builder()
.endpoint("your-minio-server.com")
.credentials(accessKey, secretKey)
.httpClient(httpClient)
.build();
Implement retry mechanisms
Add retry logic for transient failures, using exponential backoff:
public InputStream getObjectWithRetry(String bucket, String object, int maxRetries) {
int attempts = 0;
while (attempts < maxRetries) {
try {
return minioClient.getObject(
GetObjectArgs.builder()
.bucket(bucket)
.object(object)
.build());
} catch (MinioException e) {
attempts++;
if (attempts >= maxRetries) throw new RuntimeException("Failed after " + maxRetries + " attempts", e);
try {
Thread.sleep(1000 * attempts); // Exponential backoff
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
throw new RuntimeException("Interrupted during retry", ie);
}
} catch (Exception e) {
throw new RuntimeException("Unexpected error", e);
} }
throw new RuntimeException("Should not reach here");
}
Real-world use cases
MinIO is widely used for:
- Storing and retrieving large datasets for data analytics.
- Backup and archival solutions.
- Serving static assets for web applications.
- Media storage and streaming.
- AI/ML model storage and retrieval.
Example: listing objects
import io.minio.ListObjectsArgs;
import io.minio.Result;
import io.minio.messages.Item;
try {
Iterable<Result<Item>> results = minioClient.listObjects(
ListObjectsArgs.builder().bucket("my-bucket").prefix("documents/").build());
for (Result<Item> result : results) {
Item item = result.get();
System.out.println(item.objectName());
}
} catch (Exception e) {
System.err.println("Error listing objects: " + e.getMessage());
}
Troubleshooting
Connection refused
If you encounter connection issues:
- Verify that the MinIO server is running.
- Check firewall settings.
- Ensure the endpoint URL and port are correct.
- Verify network connectivity.
Access denied
For permission issues:
- Verify your access and secret keys.
- Check bucket policies and permissions.
- Ensure the bucket exists.
- Verify the object path is correct.
Conclusion
Integrating MinIO with Java provides a powerful and efficient way to manage files in your applications. The MinIO Java SDK offers a robust API for interacting with MinIO servers, including features like connection pooling, retry mechanisms, and comprehensive error handling. By following the best practices outlined in this guide, you can build secure, scalable, and high-performance applications that leverage the power of MinIO.
For further exploration, check out the official MinIO Java SDK documentation.
Transloadit also leverages MinIO. Our 🤖 MinIO Import Robot allows you to import entire directories from your MinIO buckets effortlessly, and our 🔧 Java SDK simplifies integration with Transloadit's file processing capabilities.