Harnessing PDFtk: a developer's guide to efficient PDF manipulation
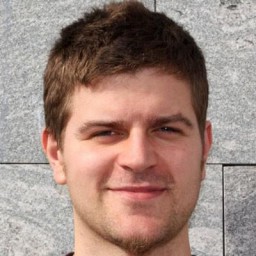
PDFtk (PDF Toolkit) is a versatile command-line tool for manipulating PDFs. In version 3.3.3 (released in 2024), it offers developers powerful capabilities for merging, splitting, compressing, and securing documents—making it an essential asset for automating document workflows.
System requirements
Before installation, ensure your system meets these prerequisites:
- Java Runtime Environment (JRE) 8 or higher (required by pdftk-java)
- Minimum 512MB RAM for basic operations
- 1GB or more RAM recommended for processing large or complex PDFs
- At least 100MB of disk space for installation
Installing PDFtk
PDFtk is available on all major operating systems. Follow the instructions below for your platform:
On Ubuntu/Debian
Update your package list and install pdftk-java:
sudo apt-get update
sudo apt-get install pdftk-java
On macOS
Install using Homebrew:
brew install pdftk-java
On Windows
Two options are available:
- PDFtk Free (GUI version): Download from the official PDFtk website
- PDFtk Server (command-line version): Download from the PDFtk Server page
Basic PDF operations
Merging PDFs
Combine multiple PDFs and handle errors gracefully. For example:
if pdftk file1.pdf file2.pdf cat output combined.pdf; then
echo "PDFs merged successfully"
else
echo "Error: Unable to merge PDFs"
exit 1
fi
You can also specify page ranges when merging:
if pdftk A=file1.pdf B=file2.pdf cat A1-5 B1-end output combined.pdf; then
echo "PDFs merged successfully"
else
echo "Error: Unable to merge PDFs"
exit 1
fi
Splitting PDFs
Extract specific pages from a document using:
# Extract pages 1 to 5
if pdftk input.pdf cat 1-5 output pages1-5.pdf; then
echo "Pages extracted successfully"
else
echo "Error: Unable to extract pages"
exit 1
fi
Optimizing PDF file size
While PDFtk does not compress PDFs directly, you can chain it with Ghostscript to optimize file size. This two-step process first standardizes the PDF and then compresses it using settings ideal for ebooks.
# Standardize the PDF with PDFtk
pdftk input.pdf output intermediate.pdf dont_ask
# Compress the standardized PDF with Ghostscript
gs -sDEVICE=pdfwrite -dCompatibilityLevel=1.4 -dPDFSETTINGS=/ebook \
-dNOPAUSE -dBATCH -sOutputFile=compressed.pdf intermediate.pdf
Security considerations
Handling sensitive PDFs requires extra care. Ensure that you protect your documents by applying strong encryption and managing file access. For example:
Secure PDF handling
Encrypt a PDF by setting an owner password with 128-bit encryption:
pdftk input.pdf output encrypted.pdf owner_pw YOUR_OWNER_PASSWORD encrypt_128bit
Set both owner and user passwords with defined permissions:
pdftk input.pdf output secure.pdf owner_pw YOUR_OWNER_PASSWORD user_pw YOUR_USER_PASSWORD \
allow printing allow ScreenReaders encrypt_128bit
Manage file permissions
Control access by restricting permissions appropriately:
pdftk input.pdf output restricted.pdf owner_pw YOUR_OWNER_PASSWORD \
allow printing allow DegradedPrinting allow ModifyAnnotations allow ScreenReaders encrypt_128bit
Always remember to secure your scripts and do not hard-code sensitive passwords directly.
Integration example
Below is a Python snippet demonstrating how to integrate PDFtk using the subprocess module with proper error handling and file path management:
import subprocess
from pathlib import Path
def run_pdftk(input_file: Path, output_file: Path, *args) -> bool:
command = ['pdftk', str(input_file)]
command.extend(args)
command.extend(['output', str(output_file)])
try:
subprocess.run(command, check=True, capture_output=True, text=True)
return True
except subprocess.CalledProcessError as e:
print(f"Error processing PDF: {e.stderr}")
return False
# Example usage
input_path = Path('input.pdf')
output_path = Path('output.pdf')
if input_path.exists():
if run_pdftk(input_path, output_path, 'dont_ask'):
print("PDF processed successfully.")
else:
print("Failed to process PDF.")
else:
print(f"Input file {input_path} not found.")
Troubleshooting common issues
Memory-related errors
When processing large PDFs, you may encounter memory constraints. To mitigate this:
-
Increase the Java heap size by setting:
export _JAVA_OPTIONS="-Xmx1024m"
-
Break the document into smaller batches to reduce memory load:
# Process 10 pages at a time pdftk input.pdf cat 1-10 output batch1.pdf pdftk input.pdf cat 11-20 output batch2.pdf
File access errors
Resolve permission issues by ensuring proper access rights:
# Display file permissions
ls -l input.pdf
# Ensure the file is readable
chmod 644 input.pdf
# Verify the output directory has the correct permissions
chmod 755 output_directory
Performance optimization
For optimal performance in large-scale operations, consider these strategies:
- Process files in batches, particularly keeping each batch below 100MB to minimize memory overhead.
- If handling multiple files, implement a queuing system to manage processing sequentially or in parallel as resources allow.
- Adjust Java's memory allocation for heavy workloads using the _JAVA_OPTIONS variable.
- Use temporary files for intermediate processing steps to reduce load on your primary files.
Here is an example Python script to create file batches based on a maximum batch size (in megabytes):
import os
from pathlib import Path
from typing import List
def get_file_size_mb(file_path: str) -> float:
return os.path.getsize(file_path) / (1024 * 1024)
def create_batches(files: List[str], max_batch_size_mb: float = 100) -> List[List[str]]:
batches = []
current_batch = []
current_size = 0
for file in files:
file_size = get_file_size_mb(file)
if current_size + file_size > max_batch_size_mb:
batches.append(current_batch)
current_batch = [file]
current_size = file_size
else:
current_batch.append(file)
current_size += file_size
if current_batch:
batches.append(current_batch)
return batches
This script can be integrated into larger automation workflows to efficiently manage high-volume PDF processing.
At Transloadit, we recognize the challenges of handling large document workflows. For enterprise-scale operations, consider integrating with Transloadit's document processing service to complement these techniques with scalable, cloud-based solutions.
Conclusion
PDFtk is a powerful tool that empowers developers to manipulate and automate PDF workflows effectively. By following the best practices outlined above—ranging from installation and basic operations to security and integration—you can build robust document processing pipelines. Explore PDFtk further, and for advanced needs, consider leveraging Transloadit's comprehensive document processing service for enhanced scalability and reliability.