Efficiently export files to Microsoft Azure using cURL
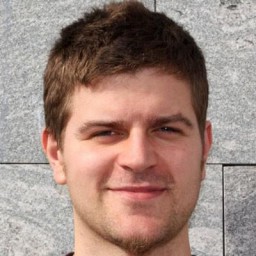
Exporting files to Microsoft Azure Storage using cURL provides a flexible and efficient way to interact with cloud storage directly from the command line. This guide walks you through the process of setting up and using cURL to export files to Azure Blob Storage, leveraging the Azure Storage REST API for seamless cloud integration.
Setting up Azure storage account
Before using cURL with Azure Storage, you need an Azure Storage account and a container. If you haven't created these yet, you can do so through the Azure Portal or Azure CLI.
Generating a sas token
To authenticate your cURL requests, you'll need a Shared Access Signature (SAS) token. This token provides secure, time-limited access to your storage resources, allowing cURL to interact with the Azure Storage REST API securely.
You can generate a SAS token through the Azure Portal:
- Navigate to your storage account.
- Select "Shared access signature".
- Configure the permissions and expiry time.
- Click "Generate SAS and connection string".
Basic file upload using cURL
Here's a basic example of uploading a file to Azure Blob Storage using cURL:
curl -X PUT \
-H "x-ms-blob-type: BlockBlob" \
-H "Content-Type: application/octet-stream" \
--data-binary "@localfile.txt" \
"https://youraccount.blob.core.windows.net/container/remotefile.txt?your_sas_token"
Replace the URL components with your storage account details and SAS token.
Handling large file uploads
For large files, it's better to use the Block Blob API, which supports chunked uploads. This involves uploading the file in smaller blocks and then committing them as a single blob:
# Upload a block
curl -X PUT \
-H "x-ms-blob-type: BlockBlob" \
--data-binary "@chunk1.txt" \
"https://youraccount.blob.core.windows.net/container/largefile.txt?comp=block&blockid=block1&your_sas_token"
# Commit the blocks
curl -X PUT \
-H "Content-Length: 0" \
-H "Content-Type: application/xml" \
--data-binary "<?xml version='1.0' encoding='utf-8'?><BlockList><Latest>block1</Latest></BlockList>" \
"https://youraccount.blob.core.windows.net/container/largefile.txt?comp=blocklist&your_sas_token"
Replace your_sas_token
with the actual SAS token and ensure that blockid
is Base64-encoded if
necessary.
Automating file exports
Create a shell script to automate the export process:
#!/bin/bash
SAS_TOKEN="your_sas_token"
STORAGE_ACCOUNT="youraccount"
CONTAINER="container"
upload_file() {
local source="$1"
local destination="$2"
curl -X PUT \
-H "x-ms-blob-type: BlockBlob" \
-H "Content-Type: application/octet-stream" \
--data-binary "@$source" \
"https://$STORAGE_ACCOUNT.blob.core.windows.net/$CONTAINER/$destination?$SAS_TOKEN"
}
# Usage
upload_file "local/path/file.txt" "remote/path/file.txt"
This script simplifies the upload process, making it easier to integrate into your workflows or automate regular exports.
Error handling and retries
Implement robust error handling and retries in your cURL commands to ensure reliability:
upload_with_retry() {
local source="$1"
local destination="$2"
local max_attempts=3
local attempt=1
while [ $attempt -le $max_attempts ]; do
if curl -X PUT \
-H "x-ms-blob-type: BlockBlob" \
--data-binary "@$source" \
"https://$STORAGE_ACCOUNT.blob.core.windows.net/$CONTAINER/$destination?$SAS_TOKEN"; then
return 0
fi
echo "Attempt $attempt failed. Retrying..."
attempt=$((attempt + 1))
sleep 2
done
echo "File upload failed after $max_attempts attempts."
return 1
}
This function attempts to upload the file up to three times before giving up, helping to handle transient network issues.
Checking file status
Verify your uploads using HEAD requests to check the file's existence and metadata:
curl -I \
"https://youraccount.blob.core.windows.net/container/file.txt?$SAS_TOKEN"
This returns the HTTP headers, allowing you to confirm the upload was successful.
Security best practices
- Always use HTTPS for transfers to encrypt data in transit.
- Generate SAS tokens with minimal required permissions (e.g., only write access if uploading).
- Set appropriate token expiration times to limit exposure.
- Use IP address restrictions in SAS tokens when possible.
- Regularly rotate SAS tokens to maintain security.
Optimizing transfer speed
To improve transfer speeds:
-
Upload Files in Parallel: If uploading multiple files, consider running uploads in parallel to maximize bandwidth usage.
-
Use Appropriate Block Sizes: For large files, adjust the block size to balance between memory usage and speed.
-
Optimize Network Settings: Ensure your network connection is stable and consider uploading from a location closer to the Azure data center.
-
Monitor Transfer Rates: Use cURL's
--verbose
flag to monitor transfer rates and diagnose bottlenecks.
Conclusion
Using cURL with Azure Blob Storage provides a powerful way to automate file exports and integrate with cloud services. By leveraging the Azure Storage REST API, you can build scripts and tools that interact directly with your cloud storage, streamlining your workflows.
If you're looking for more advanced file handling capabilities, check out Transloadit's file processing services, which offer robust solutions for file uploads, processing, and storage integration.