Efficiently read files in Node.js with the fs module
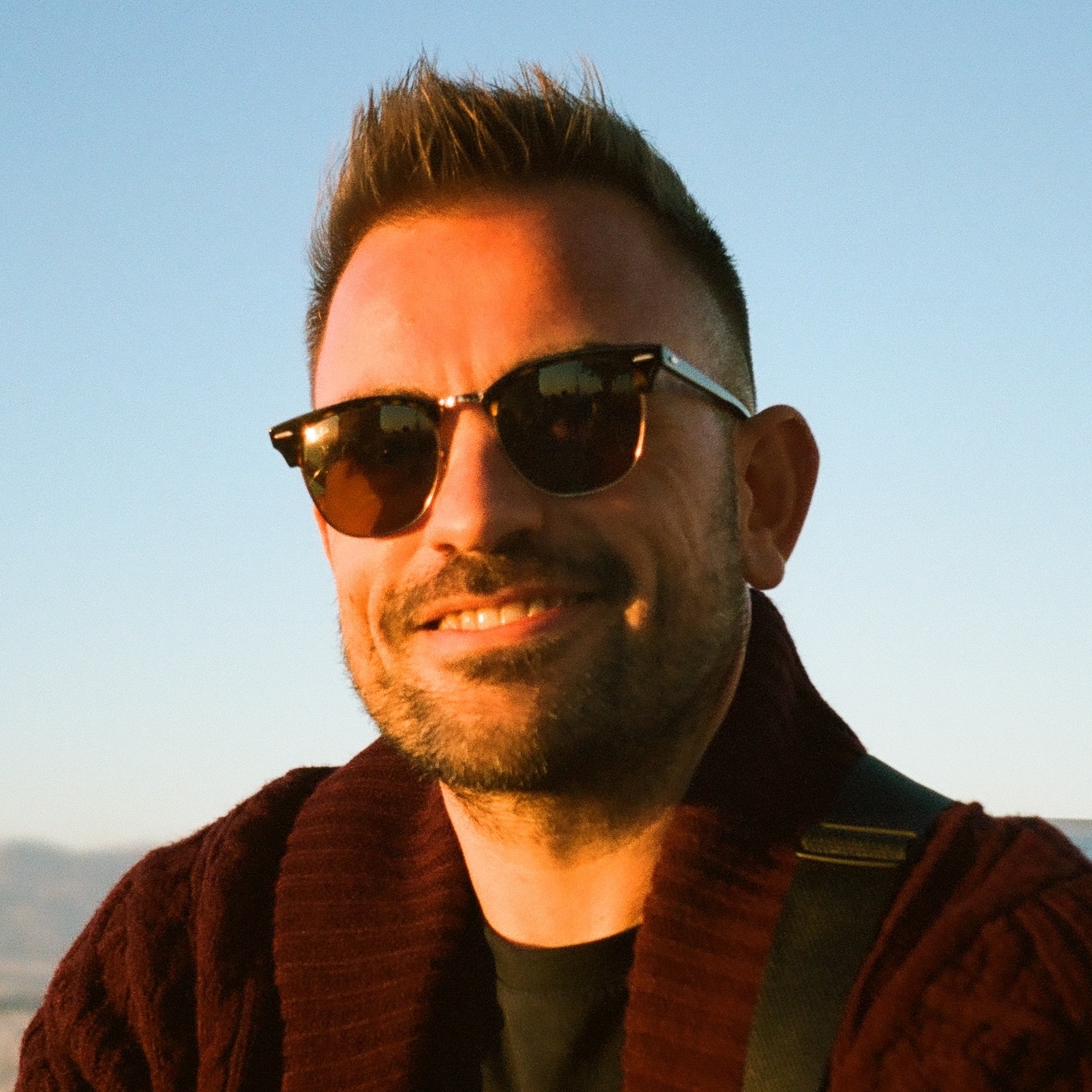
Reading file contents is a fundamental task in many Node.js applications. The built-in fs
module
provides powerful and flexible methods to handle file operations efficiently. Let's explore how to
read files effectively using the modern Promises API, older callback methods, synchronous
approaches, and streams for large files.
Introduction to file reading in Node.js
Node.js provides the fs
(file system) module, allowing you to interact with the file system
directly from your code. Whether you're reading configuration files, processing user uploads,
parsing logs, or handling large datasets, understanding how to efficiently read files is crucial for
building robust and performant applications.
Understanding the fs module
The fs
module is part of the Node.js core API and doesn't require any external installation. You
can access its functionality using either CommonJS or the modern ESM import syntax.
// CommonJS style import (older style)
const fs = require('fs')
const fsPromises = require('fs').promises
// ESM style import (modern style)
import * as fs from 'node:fs' // Access callback-based and sync functions
import { promises as fsPromises } from 'node:fs' // Access the Promises API
// Or import specific promise-based functions directly (recommended)
import { readFile, open, createReadStream } from 'node:fs/promises'
import { createReadStream as createReadStreamSync } from 'node:fs' // For sync stream creation if needed
We recommend using the Promises API (node:fs/promises
) for new development due to its advantages
with async/await
.
Reading files asynchronously with the promises API (recommended)
The fs/promises
API is the modern standard for asynchronous file operations in Node.js. It
integrates seamlessly with async/await
, leading to cleaner, more readable code and simpler error
handling compared to callbacks.
import { readFile } from 'node:fs/promises'
async function readFileExample() {
try {
// Specify 'utf8' encoding to get a string; otherwise, a Buffer is returned.
const data = await readFile('example.txt', 'utf8')
console.log('File contents (promises):', data)
} catch (err) {
// Handles errors like file not found or permission issues
console.error('Error reading file:', err)
}
}
readFileExample()
This approach directly answers: "How do I read files asynchronously in Node.js?"
For reading multiple files concurrently, Promise.all()
is efficient:
import { readFile } from 'node:fs/promises'
async function readMultipleFiles() {
const filesToRead = ['file1.txt', 'file2.txt']
try {
const results = await Promise.all(filesToRead.map((file) => readFile(file, 'utf8')))
console.log('File 1:', results[0])
console.log('File 2:', results[1])
} catch (err) {
console.error('Error reading multiple files:', err)
}
}
readMultipleFiles()
Reading files asynchronously with fs.readfile() (callback api)
Before the Promises API, asynchronous operations used callbacks. While functional, this can lead to nested code ("callback hell") and more complex error handling.
const fs = require('fs') // Using CommonJS for this example
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file (callback):', err)
return
}
console.log('File contents (callback):', data)
})
Synchronous file reading with fs.readfilesync()
Synchronous methods block the Node.js event loop until the operation completes. This can be acceptable for simple scripts or during application initialization (e.g., loading essential configuration) but should be avoided in server applications handling concurrent requests, as it severely impacts performance.
const fsSync = require('fs') // Using CommonJS for clarity
try {
const data = fsSync.readFileSync('example.txt', 'utf8')
console.log('File contents (sync):', data)
} catch (err) {
console.error('Error reading file synchronously:', err)
}
Handling large files efficiently with streams
When dealing with files too large to fit comfortably in memory, streams are the solution. Streams read data in manageable chunks, allowing you to process it piece by piece without high memory usage.
import { createReadStream } from 'node:fs' // Use the callback-based version for streams
// Create a readable stream
const stream = createReadStream('largefile.txt', { encoding: 'utf8' })
// Event handler for incoming data chunks
stream.on('data', (chunk) => {
console.log(`Received ${chunk.length} bytes of data.`)
// Process the chunk here (e.g., parse, transform, write elsewhere)
})
// Event handler for the end of the stream
stream.on('end', () => {
console.log('Finished reading the file.')
})
// Crucial: Event handler for errors during streaming
stream.on('error', (err) => {
console.error('Stream error:', err)
// Clean up the stream resources if an error occurs
stream.destroy()
})
You can also pipe
readable streams to writable streams for efficient data transfer, like reading
from a file and writing to the console or another file:
import { createReadStream } from 'node:fs'
const readable = createReadStream('input.txt')
const writable = process.stdout // Example: writing to console
// Handle errors on the source stream
readable.on('error', (err) => {
console.error('Source stream error:', err)
readable.destroy()
writable.end() // Ensure destination stream is closed if source fails
})
// Handle errors on the destination stream or during the pipe operation
writable.on('error', (err) => {
console.error('Destination stream or pipe error:', err)
readable.destroy() // Stop reading if writing fails
})
// Pipe data from readable to writable
readable.pipe(writable)
Using streams effectively addresses the question: "What are the best practices for handling large files in Node.js?"
Using filehandle for advanced operations
For more fine-grained control, such as reading specific parts of a file, writing at specific
positions, or performing multiple operations without repeatedly opening/closing the file, the
FileHandle
API (via fs/promises
) is powerful. It provides an object representing an open file
descriptor.
import { open } from 'node:fs/promises'
async function readWithFileHandle() {
let fileHandle = null // Declare outside try for access in finally
try {
// Open the file for reading ('r')
fileHandle = await open('example.txt', 'r')
// Read the entire file content using the handle
const content = await fileHandle.readFile({ encoding: 'utf8' })
console.log('File content (FileHandle):', content)
// Example: Read the first 10 bytes
const buffer = Buffer.alloc(10)
const { bytesRead } = await fileHandle.read(buffer, 0, 10, 0) // buffer, offset, length, position
console.log(`Read ${bytesRead} bytes: ${buffer.toString()}`)
} catch (err) {
console.error('Error using FileHandle:', err)
} finally {
// Crucial: Always close the file handle to release system resources
if (fileHandle) {
await fileHandle.close()
console.log('File handle closed.')
}
}
}
readWithFileHandle()
Error handling and best practices
Robust error handling is essential when working with the file system.
- Promises (
async/await
): Usetry...catch
blocks for clean error management. - Callbacks: Always check the first
err
argument in the callback. - Streams: Implement
error
event listeners on all streams involved in a pipeline. Ensure resources are cleaned up (destroy()
,close()
) on error. - File Handles: Use
try...catch...finally
to guaranteefileHandle.close()
is called. - Permissions: Ensure your Node.js process has the necessary read permissions for the target files.
- Existence Checks: Consider checking if a file exists (e.g., using
fsPromises.access
) before attempting to read it, although often it's better to handle theENOENT
(file not found) error directly in thecatch
block.
Performance considerations
Choosing the right method significantly impacts performance and resource usage:
- Streams: Best for large files due to minimal memory footprint. Ideal for processing data sequentially.
- Promises API (
readFile
): Suitable for small to medium files where loading the entire content into memory is acceptable. Offers good balance of performance and ease of use withasync/await
. - Synchronous API (
readFileSync
): Highest potential performance for a single read if blocking is acceptable (scripts, initialization), but disastrous for concurrent server workloads. FileHandle
: Can be more efficient than repeatedreadFile
calls if multiple operations (reads, writes, stats) are needed on the same file, as it keeps the file descriptor open. Adds slight overhead for single reads.- Concurrency: Use
Promise.all
withreadFile
for concurrent reads of multiple small files, but be mindful of OS limits on open file descriptors.
Real-world use cases and examples
Efficient file reading is fundamental in:
- Loading application configuration (e.g.,
.env
,config.json
). - Processing user uploads in web applications.
- Parsing large log files or CSV datasets.
- Streaming video or audio data.
- Reading HTML/CSS/JS assets or template files.
At Transloadit, we leverage efficient file handling techniques extensively. For instance, our 🤖 /file/read Robot can efficiently read file contents as part of a larger processing Assembly. Note that this specific Robot currently accepts files under 500KB.
Conclusion and additional resources
Node.js offers a versatile fs
module for reading files. Prioritize the non-blocking, asynchronous
fs/promises
API with async/await
for modern applications. Use streams for large files to manage
memory effectively. Reserve synchronous methods for specific cases like simple scripts or
initialization code. Proper error handling is crucial regardless of the method chosen.
For comprehensive details, refer to the official Node.js File System documentation.
If your application involves complex file processing, encoding, or manipulation workflows, consider exploring tools and services designed for these tasks, such as the Transloadit Node.js SDK.