Effortless audio encoding in the browser with WebAssembly
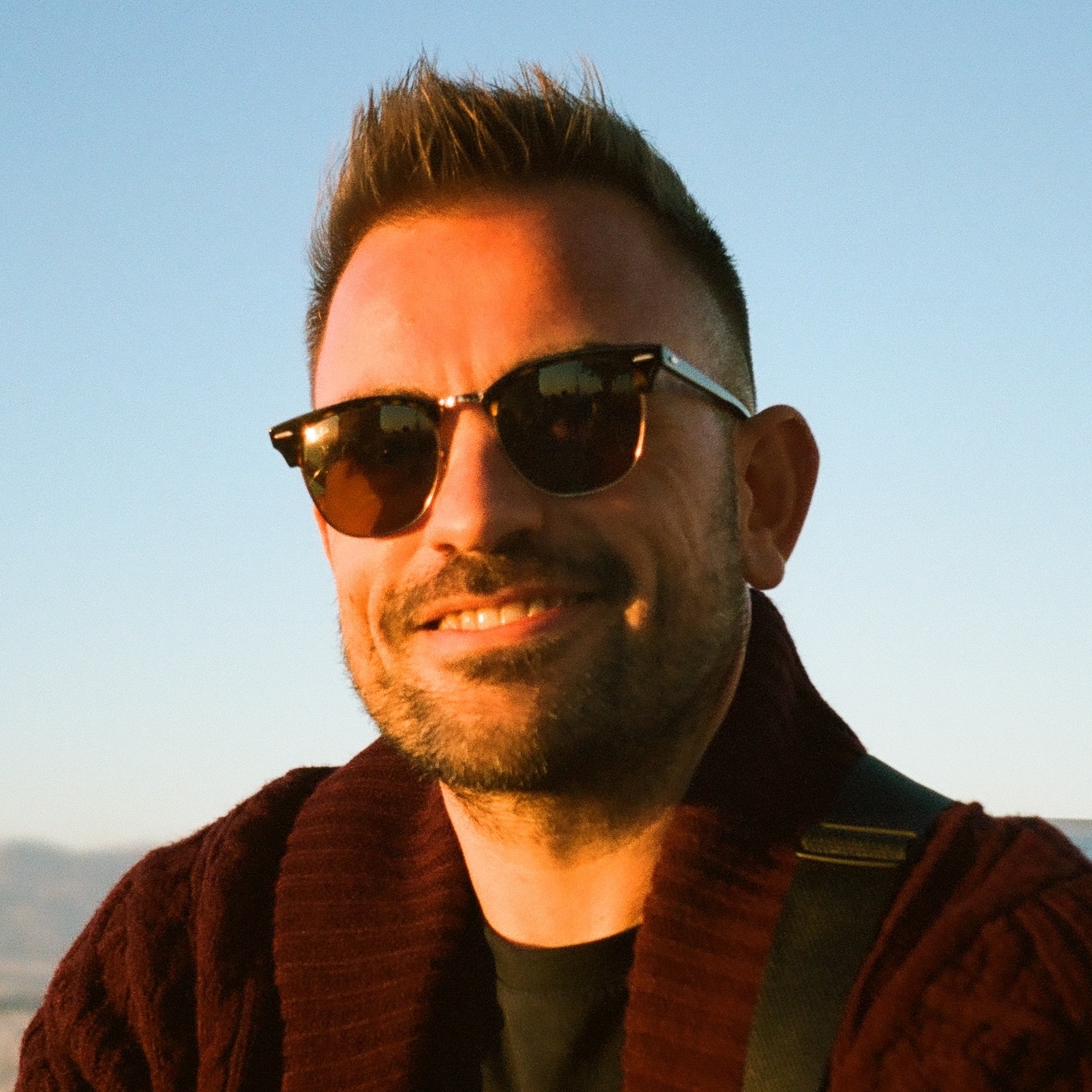
Encoding audio in browsers has evolved significantly over the years. With the introduction of WebAssembly, developers can now perform complex audio processing tasks directly in web applications. In this post, we'll explore how you can harness WebAssembly to encode audio efficiently in browsers.
Introduction to browser-based audio encoding
Traditionally, audio encoding was performed on the server side due to the limitations of JavaScript in handling compute‐intensive tasks. However, with the increasing need for responsive and interactive web applications, offloading some of these tasks to the client side has become advantageous.
The role of WebAssembly in audio processing
WebAssembly (Wasm) is a low-level bytecode format that runs in the browser at near-native speed. It allows developers to compile code written in languages like C, C++, or Rust into a format that can be executed efficiently on the client. This is particularly useful for encoding tasks that require high performance.
Benefits of using WebAssembly for audio encoding
- Performance: Runs at speeds comparable to native applications, outperforming traditional JavaScript in compute‐intensive operations.
- Efficiency: Encoding on the client reduces server load and bandwidth usage, resulting in cost and performance benefits.
- Flexibility: Allows you to leverage existing audio encoding libraries written in C or Rust, expanding your toolkit.
Setting up a simple web application for audio encoding
Let's start by setting up a basic web application that handles audio encoding directly in the browser.
Prerequisites
- Basic knowledge of JavaScript and HTML.
- Node.js and npm installed on your machine.
Project setup
Create a new directory for your project and initialize it:
mkdir webassembly-audio-encoder
cd webassembly-audio-encoder
npm init -y
Install the necessary dependencies:
npm install @ffmpeg/ffmpeg@0.12.15 @ffmpeg/util
Development server setup
Since we're using modules and Wasm files, you'll need a proper development server. Create a simple server using Express:
npm install express
Create a file named server.js
:
const express = require('express')
const app = express()
app.use(express.static('.'))
app.use((req, res, next) => {
res.header('Cross-Origin-Opener-Policy', 'same-origin')
res.header('Cross-Origin-Embedder-Policy', 'require-corp')
res.header('Cross-Origin-Resource-Policy', 'cross-origin')
next()
})
app.listen(3000, () => {
console.log('Server running at http://localhost:3000')
})
Update your package.json
scripts section:
{
"scripts": {
"start": "node server.js"
}
}
Run the server with:
npm start
Ffmpeg.wasm setup and usage
After installing the dependencies, create an index.html
file:
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>FFmpeg.wasm Audio Encoder</title>
</head>
<body>
<input type="file" id="uploader" accept="audio/*" />
<button id="encodeButton">Encode Audio</button>
<div id="progress"></div>
<script type="module" src="index.js"></script>
</body>
</html>
Create an index.js
file to handle the FFmpeg.wasm implementation:
import { FFmpeg } from '@ffmpeg/ffmpeg'
import { fetchFile, toBlobURL } from '@ffmpeg/util'
const ffmpeg = new FFmpeg()
async function init() {
try {
const baseURL = 'https://unpkg.com/@ffmpeg/core@0.12.15/dist/umd'
await ffmpeg.load({
coreURL: await toBlobURL(`${baseURL}/ffmpeg-core.js`, 'text/javascript'),
wasmURL: await toBlobURL(`${baseURL}/ffmpeg-core.wasm`, 'application/wasm'),
})
console.log('FFmpeg is ready!')
} catch (error) {
console.error('FFmpeg initialization failed:', error)
throw error
}
}
async function encodeFile() {
const uploader = document.getElementById('uploader')
const progressDiv = document.getElementById('progress')
if (!uploader.files.length) {
throw new Error('Please select an audio file')
}
try {
if (!ffmpeg.loaded) {
await init()
}
const file = uploader.files[0]
const inputFileName = file.name
const outputFileName = 'output.mp3'
const inputData = await fetchFile(file)
await ffmpeg.writeFile(inputFileName, inputData)
ffmpeg.on('progress', ({ progress }) => {
progressDiv.textContent = `Progress: ${(progress * 100).toFixed(2)}%`
})
await ffmpeg.exec(['-i', inputFileName, '-c:a', 'libmp3lame', '-b:a', '192k', outputFileName])
const outputData = await ffmpeg.readFile(outputFileName)
const blob = new Blob([outputData], { type: 'audio/mp3' })
const url = URL.createObjectURL(blob)
const a = document.createElement('a')
a.href = url
a.download = outputFileName
a.click()
URL.revokeObjectURL(url)
} catch (error) {
console.error('Error during encoding:', error)
progressDiv.textContent = `Error: ${error.message}`
}
}
document.getElementById('encodeButton').addEventListener('click', async () => {
try {
await encodeFile()
} catch (error) {
console.error('Encoding failed:', error)
}
})
Direct WebAssembly implementation
Below is an updated example of using WebAssembly directly to process audio data. This example uses Emscripten's embind to expose a simple gain adjustment function to JavaScript.
#include <emscripten/bind.h>
#include <emscripten/val.h>
void apply_gain(float* samples, int length, float gain) {
for (int i = 0; i < length; i++) {
samples[i] *= gain;
}
}
EMSCRIPTEN_BINDINGS(audio_module) {
emscripten::function("applyGain", &apply_gain);
}
Compile this C++ code with modern Emscripten settings:
emcc audio_processor.cpp -o audio_processor.js \
-s WASM=1 \
-s EXPORTED_RUNTIME_METHODS='["ccall","cwrap"]' \
-s EXPORTED_FUNCTIONS='["_malloc","_free"]' \
-s NO_EXIT_RUNTIME=1 \
-s ALLOW_MEMORY_GROWTH=1 \
-O3 \
--bind
Use the resulting module in JavaScript as follows:
class AudioProcessorWrapper {
constructor() {
this.module = null
this.processor = null
}
async initialize() {
try {
this.module = await createModule()
// The module now exposes the applyGain function via embind
this.processor = this.module
} catch (error) {
console.error('Failed to initialize AudioProcessor:', error)
throw error
}
}
async processAudioBuffer(audioBuffer, gain) {
if (!this.processor) {
throw new Error('AudioProcessor not initialized')
}
const channel = audioBuffer.getChannelData(0)
const bytesPerSample = Float32Array.BYTES_PER_ELEMENT
const numSamples = channel.length
const heapPtr = this.module._malloc(numSamples * bytesPerSample)
const heapFloat32 = new Float32Array(this.module.HEAPF32.buffer, heapPtr, numSamples)
heapFloat32.set(channel)
// Call the exposed applyGain function
this.module.ccall(
'applyGain',
null,
['number', 'number', 'number'],
[heapPtr, numSamples, gain],
)
channel.set(heapFloat32)
this.module._free(heapPtr)
return audioBuffer
}
}
Integrating WebAssembly with JavaScript for enhanced audio features
Modern audio processing requires tight integration between WebAssembly and the Web Audio API. The following example shows how you can use an AudioWorkletProcessor to offload audio processing to Wasm code:
class AudioWorkletProcessorWrapper extends AudioWorkletProcessor {
constructor() {
super()
this.port.onmessage = this.handleMessage.bind(this)
}
async handleMessage(event) {
if (event.data.type === 'loadWasm') {
try {
const { wasmModule } = event.data
this.wasmInstance = await WebAssembly.instantiate(wasmModule)
} catch (error) {
this.port.postMessage({ type: 'error', error: error.message })
}
}
}
process(inputs, outputs, parameters) {
if (!this.wasmInstance) return true
const input = inputs[0]
const output = outputs[0]
for (let channel = 0; channel < input.length; channel++) {
const inputChannel = input[channel]
const outputChannel = output[channel]
// Process the audio samples using the Wasm export
this.wasmInstance.exports.processAudio(inputChannel, outputChannel)
}
return true
}
}
registerProcessor('audio-processor', AudioWorkletProcessorWrapper)
Testing and optimizing audio encoding performance
Modern browser audio processing tasks benefit from rigorous performance optimization. Consider the following compatibility check which ensures that critical features are available:
async function checkBrowserSupport() {
const features = {
webAssembly: typeof WebAssembly === 'object',
audioContext: !!(window.AudioContext || window.webkitAudioContext),
audioWorklet: !!window.AudioWorklet,
sharedArrayBuffer: typeof SharedArrayBuffer === 'function',
}
const missingFeatures = Object.entries(features)
.filter(([, supported]) => !supported)
.map(([feature]) => feature)
if (missingFeatures.length > 0) {
throw new Error(`Missing required features: ${missingFeatures.join(', ')}`)
}
return true
}
Note: This compatibility check covers modern browsers such as Chrome 93+, Firefox 90+, Safari 16.4+, and Edge 93+.
Initialize your AudioWorklet-enabled context as follows:
async function initializeAudioProcessor() {
try {
await checkBrowserSupport()
const AudioContext = window.AudioContext || window.webkitAudioContext
const audioContext = new AudioContext()
await audioContext.audioWorklet.addModule('audio-processor.js')
return audioContext
} catch (error) {
console.error('Audio initialization failed:', error)
throw error
}
}
Deploying and future-proofing your web audio application
Modern deployment of web audio applications requires careful balancing between performance, security, and future updates. The following class outlines a basic architecture for an audio application that integrates the previous components:
class AudioApplication {
constructor() {
this.audioContext = null
this.processor = null
this.initialized = false
}
async initialize() {
try {
await checkBrowserSupport()
this.audioContext = await initializeAudioProcessor()
this.processor = new AudioProcessorWrapper()
await this.processor.initialize()
this.initialized = true
} catch (error) {
console.error('Application initialization failed:', error)
throw error
}
}
async processAudio(audioBuffer) {
if (!this.initialized) {
throw new Error('Audio application not initialized')
}
try {
return await this.processor.processAudioBuffer(audioBuffer, 1.0)
} catch (error) {
console.error('Error processing audio:', error)
throw error
}
}
}
Conclusion
In this post, we explored how to leverage WebAssembly to perform efficient audio encoding directly in the browser. From using FFmpeg.wasm for audio file conversion to integrating a contemporary WebAssembly module and the Web Audio API, you now have a roadmap for building high-performance audio applications.
For more robust audio processing solutions and advanced upload handling, consider exploring Transloadit's offerings—integrated with tools like Uppy and Tus—to further streamline your development workflow. Visit Transloadit to learn more.