Effortless file export to OpenStack Swift in .Net C#
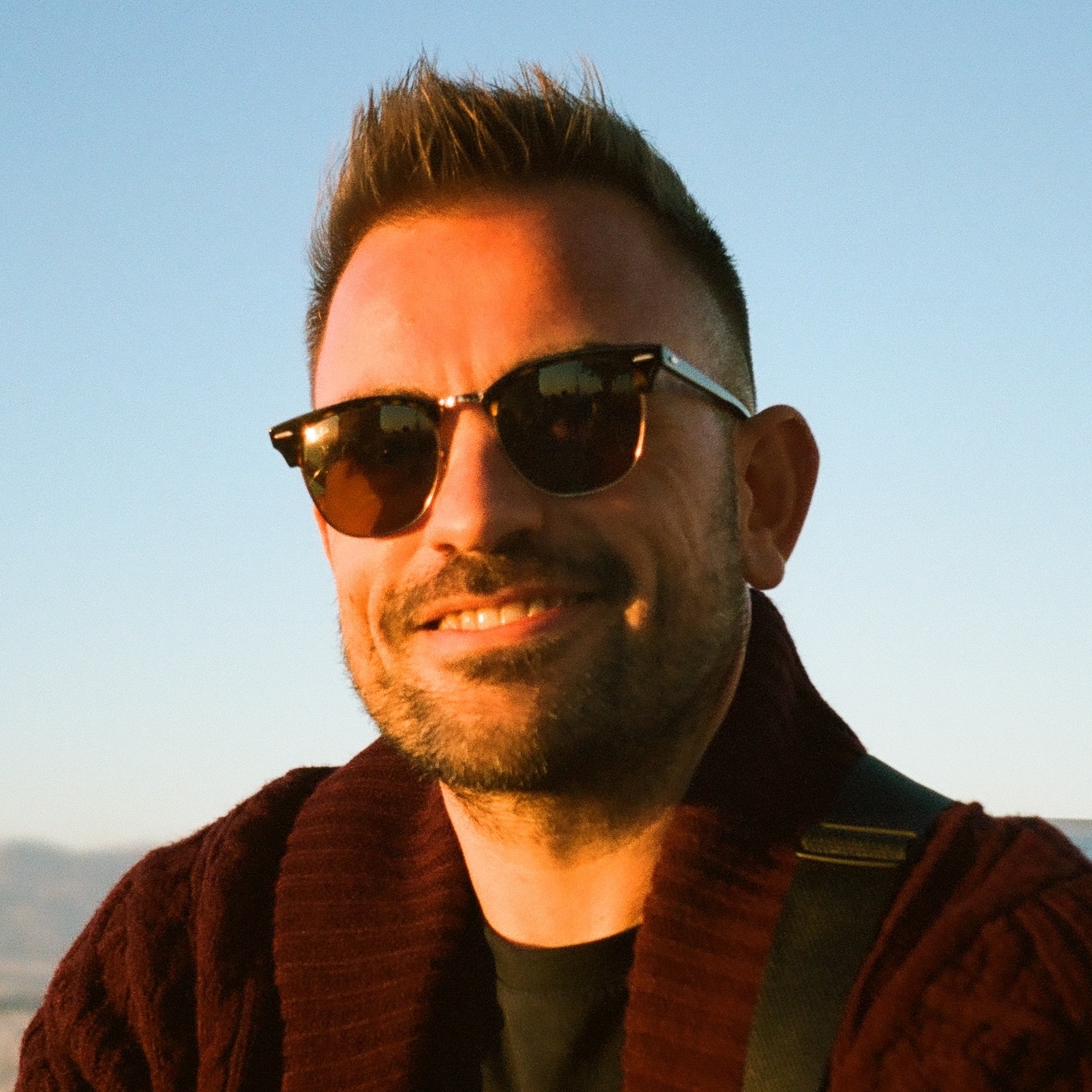
OpenStack Swift provides scalable and reliable object storage, making it an excellent choice for .NET developers looking to integrate cloud storage into their applications. In this guide, we'll explore how to export files to OpenStack Swift using .NET C#, with practical examples and code snippets to help you implement this functionality in your projects.
Introduction to OpenStack Swift
OpenStack Swift is an open-source object storage system that's highly available, distributed, and scalable. It allows you to store and retrieve large amounts of unstructured data with a RESTful API interface.
For .NET developers, integrating OpenStack Swift can enhance your applications by providing cloud storage capabilities for files such as images, videos, documents, and more.
Understanding the restful API for OpenStack Swift
OpenStack Swift operates through a RESTful API, allowing clients to interact with the storage system using standard HTTP methods. The key operations you'll use include:
- PUT: Uploads or updates objects (files) in the storage.
- GET: Retrieves objects or lists containers and objects.
- DELETE: Deletes objects or containers.
- HEAD: Retrieves metadata about containers or objects.
To interact with these APIs in .NET C#, we'll use HTTP client libraries.
Setting up your environment
Prerequisites
Before starting, ensure you have:
- Visual Studio or Visual Studio Code installed.
- .NET Framework 4.7.2 or later, or .NET Core 3.1 or later.
- An OpenStack Swift account with access credentials (username, password, project name, and authentication endpoint).
Handling authentication: secure access to your Swift account
Authentication is required to interact with OpenStack Swift. You need to obtain an authentication token by sending your credentials to the OpenStack Identity Service (Keystone).
Here's how to authenticate and obtain an auth token in C#:
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json;
using System.Linq;
public class SwiftAuthenticator
{
private readonly string _authUrl;
private readonly string _username;
private readonly string _password;
private readonly string _projectName;
public SwiftAuthenticator(string authUrl, string username, string password, string projectName)
{
_authUrl = authUrl;
_username = username;
_password = password;
_projectName = projectName;
}
public async Task<(string token, string storageUrl)> AuthenticateAsync()
{
var authRequest = new
{
auth = new
{
identity = new
{
methods = new[] { "password" },
password = new
{
user = new
{
name = _username,
domain = new { name = "Default" },
password = _password
}
}
},
scope = new
{
project = new
{
name = _projectName,
domain = new { name = "Default" }
}
}
}
};
using var client = new HttpClient();
var content = new StringContent(JsonConvert.SerializeObject(authRequest), Encoding.UTF8, "application/json");
var response = await client.PostAsync($"{_authUrl}/auth/tokens", content);
response.EnsureSuccessStatusCode();
var token = response.Headers.GetValues("X-Subject-Token").FirstOrDefault();
var responseBody = await response.Content.ReadAsStringAsync();
dynamic jsonResponse = JsonConvert.DeserializeObject(responseBody);
string storageUrl = null;
foreach (var catalog in jsonResponse.token.catalog)
{
if (catalog.type == "object-store")
{
foreach (var endpoint in catalog.endpoints)
{
if (endpoint.@interface == "public" && endpoint.region == "RegionOne")
{
storageUrl = endpoint.url;
break;
}
}
}
}
return (token, storageUrl);
}
}
Exporting files to OpenStack Swift
Now that we have authentication set up, let's implement file uploading to a Swift container.
Creating a container
Before uploading files, ensure that the container (bucket) exists. If not, you can create it:
public async Task CreateContainerAsync(string containerName, string authToken, string storageUrl)
{
using var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Put, $"{storageUrl}/{containerName}");
request.Headers.Add("X-Auth-Token", authToken);
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
}
Uploading a file
Here's how to upload a file to a container:
public async Task UploadFileAsync(string containerName, string objectName, string filePath, string authToken, string storageUrl)
{
using var client = new HttpClient();
var fileBytes = await File.ReadAllBytesAsync(filePath);
var content = new ByteArrayContent(fileBytes);
var request = new HttpRequestMessage(HttpMethod.Put, $"{storageUrl}/{containerName}/{objectName}");
request.Headers.Add("X-Auth-Token", authToken);
request.Content = content;
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
}
Practical example: exporting image files
Let's put it all together in a simple console application:
using System;
using System.Threading.Tasks;
namespace SwiftFileExporter
{
class Program
{
static async Task Main(string[] args)
{
var authUrl = "https://your-openstack-endpoint:5000/v3";
var username = "your_username";
var password = "your_password";
var projectName = "your_project";
var containerName = "my-container";
var filePath = "path/to/your/image.jpg";
var objectName = "images/image.jpg";
var authenticator = new SwiftAuthenticator(authUrl, username, password, projectName);
var (authToken, storageUrl) = await authenticator.AuthenticateAsync();
await CreateContainerAsync(containerName, authToken, storageUrl);
await UploadFileAsync(containerName, objectName, filePath, authToken, storageUrl);
Console.WriteLine("File uploaded successfully to OpenStack Swift.");
}
public static async Task CreateContainerAsync(string containerName, string authToken, string storageUrl)
{
using var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Put, $"{storageUrl}/{containerName}");
request.Headers.Add("X-Auth-Token", authToken);
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
}
public static async Task UploadFileAsync(string containerName, string objectName, string filePath, string authToken, string storageUrl)
{
using var client = new HttpClient();
var fileBytes = await File.ReadAllBytesAsync(filePath);
var content = new ByteArrayContent(fileBytes);
var request = new HttpRequestMessage(HttpMethod.Put, $"{storageUrl}/{containerName}/{objectName}");
request.Headers.Add("X-Auth-Token", authToken);
request.Content = content;
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
}
}
}
Improving your workflow: automating file exports
To automate exports or handle large files, consider implementing chunked uploads or background services that monitor directories and upload files when changes are detected.
Handling large files with segmented uploads
For large files, OpenStack Swift supports segmented uploads. You can upload file segments and then create a manifest file that tells Swift how to assemble them.
Here's an example of uploading a large file in segments:
public async Task UploadLargeFileAsync(string containerName, string objectName, string filePath, string authToken, string storageUrl)
{
const int segmentSize = 104857600; // 100 MB
var fileInfo = new FileInfo(filePath);
var totalSegments = (int)Math.Ceiling((double)fileInfo.Length / segmentSize);
using var fileStream = File.OpenRead(filePath);
for (int segment = 0; segment < totalSegments; segment++)
{
var segmentName = $"{objectName}/{segment:D8}";
var bufferSize = (int)Math.Min(segmentSize, fileInfo.Length - (segment * segmentSize));
var buffer = new byte[bufferSize];
await fileStream.ReadAsync(buffer, 0, bufferSize);
using var client = new HttpClient();
var content = new ByteArrayContent(buffer);
var request = new HttpRequestMessage(HttpMethod.Put, $"{storageUrl}/{containerName}/{segmentName}");
request.Headers.Add("X-Auth-Token", authToken);
request.Content = content;
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
}
// Create manifest file
using var manifestClient = new HttpClient();
var manifestRequest = new HttpRequestMessage(HttpMethod.Put, $"{storageUrl}/{containerName}/{objectName}");
manifestRequest.Headers.Add("X-Auth-Token", authToken);
manifestRequest.Headers.Add("X-Object-Manifest", $"{containerName}/{objectName}/");
manifestRequest.Content = new StringContent("");
var manifestResponse = await manifestClient.SendAsync(manifestRequest);
manifestResponse.EnsureSuccessStatusCode();
}
Potential challenges and how to overcome them
- Authentication Issues: Ensure your credentials are correct and you're using the correct authentication endpoint.
- Network Errors: Implement retry logic and exception handling to account for network interruptions.
- Large Files: For files larger than a certain size, consider using segmented (chunked) uploads.
Conclusion
Integrating OpenStack Swift into your .NET applications provides robust cloud storage capabilities, allowing you to manage files efficiently. By following this guide, you can set up authentication, create containers, and export files to OpenStack Swift using C#.
For enhanced file upload capabilities, consider using Tus for resumable uploads and Uppy for a comprehensive, open-source file uploader.