Effortless file importing from OpenStack Swift in Java
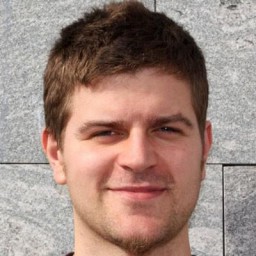
OpenStack Swift is a highly scalable object storage system that empowers you to store and retrieve files efficiently in the cloud. In this guide, we explore how to import files from OpenStack Swift into your Java applications using open-source libraries such as OpenStack4j, and we cover best practices for seamless cloud storage integration.
Setting up your Java environment
Before you begin, ensure you have the following prerequisites:
- Java Development Kit (JDK) 11 or later
- Maven or Gradle for dependency management
- OpenStack Swift credentials (authentication URL, username, password, and domain/project name)
Add the OpenStack4j dependency to your project. For Maven, include the following in your pom.xml
:
<dependency>
<groupId>com.github.openstack4j.core</groupId>
<artifactId>openstack4j</artifactId>
<version>3.12</version>
</dependency>
For Gradle, add to your build.gradle
:
implementation 'com.github.openstack4j.core:openstack4j:3.12'
Connecting to OpenStack Swift
Establish a connection to your OpenStack Swift instance using OpenStack4j. The example below demonstrates project-scoped authentication. Make sure you provide both a domain and a project name to avoid authorization issues:
import org.openstack4j.api.OSClient.OSClientV3;
import org.openstack4j.model.common.Identifier;
import org.openstack4j.openstack.OSFactory;
public class SwiftConnector {
private final OSClientV3 os;
public SwiftConnector(String authUrl, String username, String password,
String domainName, String projectName) {
this.os = OSFactory.builderV3()
.endpoint(authUrl)
.credentials(username, password, Identifier.byName(domainName))
.scopeToProject(Identifier.byName(projectName), Identifier.byName(domainName))
.authenticate();
}
public OSClientV3 getClient() {
return os;
}
}
Importing files from Swift
The following example shows how to import a file from a Swift container into your Java application. This code retrieves the object, downloads its content, and saves it locally.
import org.openstack4j.model.storage.object.SwiftObject;
import java.io.FileOutputStream;
import java.io.InputStream;
public class SwiftFileImporter {
private final OSClientV3 os;
public SwiftFileImporter(OSClientV3 os) {
this.os = os;
}
public void importFile(String containerName, String objectName, String localPath) {
try {
SwiftObject obj = os.objectStorage()
.objects()
.get(containerName, objectName);
if (obj == null) {
throw new RuntimeException("Object not found: " + objectName);
}
try (InputStream is = obj.download().getInputStream();
FileOutputStream fos = new FileOutputStream(localPath)) {
byte[] buffer = new byte[8192];
int bytesRead;
while ((bytesRead = is.read(buffer)) != -1) {
fos.write(buffer, 0, bytesRead);
}
}
} catch (Exception e) {
throw new RuntimeException("Failed to import file: " + objectName, e);
}
}
}
Handling large files efficiently
For large files, implement efficient streaming with progress tracking to ensure smooth operations without exhausting memory:
public class LargeFileImporter {
private static final int BUFFER_SIZE = 1024 * 1024; // 1MB buffer
private final OSClientV3 os;
public LargeFileImporter(OSClientV3 os) {
this.os = os;
}
public void importLargeFile(String containerName, String objectName, String localPath) {
try {
SwiftObject obj = os.objectStorage()
.objects()
.get(containerName, objectName);
if (obj == null) {
throw new RuntimeException("Object not found: " + objectName);
}
long contentLength = obj.getSizeInBytes();
try (InputStream is = obj.download().getInputStream();
FileOutputStream fos = new FileOutputStream(localPath)) {
byte[] buffer = new byte[BUFFER_SIZE];
long totalBytesRead = 0;
int bytesRead;
while ((bytesRead = is.read(buffer)) != -1) {
fos.write(buffer, 0, bytesRead);
totalBytesRead += bytesRead;
double progress = (double) totalBytesRead / contentLength * 100;
System.out.printf("Download progress: %.2f%%\n", progress);
}
}
} catch (Exception e) {
throw new RuntimeException("Failed to import large file: " + objectName, e);
}
}
}
Implementing error handling and retries
Robust error handling is essential, especially when network issues or transient errors occur. The example below retries the download up to three times before ultimately failing.
public class RetryableSwiftImporter {
private static final int MAX_RETRIES = 3;
private static final long RETRY_DELAY_MS = 2000;
private final OSClientV3 os;
public RetryableSwiftImporter(OSClientV3 os) {
this.os = os;
}
public void importWithRetry(String containerName, String objectName, String localPath) {
int attempts = 0;
Exception lastException = null;
while (attempts < MAX_RETRIES) {
try {
SwiftObject obj = os.objectStorage()
.objects()
.get(containerName, objectName);
if (obj == null) {
throw new RuntimeException("Object not found: " + objectName);
}
downloadFile(obj, localPath);
return;
} catch (Exception e) {
lastException = e;
attempts++;
if (attempts < MAX_RETRIES) {
try {
Thread.sleep(RETRY_DELAY_MS * attempts);
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
throw new RuntimeException("Import interrupted", ie);
}
}
}
}
throw new RuntimeException("Failed to import after " + MAX_RETRIES + " attempts", lastException);
}
private void downloadFile(SwiftObject obj, String localPath) throws Exception {
try (InputStream is = obj.download().getInputStream();
FileOutputStream fos = new FileOutputStream(localPath)) {
is.transferTo(fos);
}
}
}
Error handling considerations
When working with OpenStack Swift, consider the following common error scenarios and tailor your error handling accordingly:
- Container Not Found: Ensure the specified container exists in your Swift instance.
- Object Not Found: Verify the object name and its path.
- Authentication Failures: Double-check your credentials, domain name, and project name for proper scoping.
- Quota Exceeded: Monitor your storage limits and usage to avoid surpassing allocated quotas.
Providing detailed error messages and handling these cases explicitly can significantly improve troubleshooting and system reliability.
Performance optimization tips
To optimize your Swift file importing workflow, consider the following practices:
-
Connection Management:
- Reuse OSClientV3 instances when possible
- Implement connection pooling for multiple concurrent operations
- Set appropriate timeouts based on your use case
-
Buffer Size Optimization:
- Use larger buffers (1MB or more) for transferring large files
- Adjust the buffer size based on available memory and file sizes
- Monitor memory usage during transfers
-
Resource Management:
- Always use try-with-resources for proper cleanup of streams and connections
- Explicitly close resources in finally blocks if necessary
- Log exceptions and clean up resources to avoid memory leaks
-
Security Best Practices:
- Store credentials securely using environment variables or secure vaults
- Use HTTPS endpoints for all connections to protect data in transit
- Implement proper access controls and audit logging
-
Bulk File Operations:
- When importing numerous files, consider using Swift's native bulk operations if available, or process files sequentially to manage memory more effectively
Conclusion
By leveraging OpenStack4j, Java developers can efficiently integrate OpenStack Swift into their applications for seamless file importing and cloud storage management. The examples and best practices provided here form a robust starting point for building efficient file importing solutions.
For more advanced file handling capabilities and automated processing workflows, consider exploring Transloadit.