Export files to Amazon S3 using PHP: a comprehensive guide
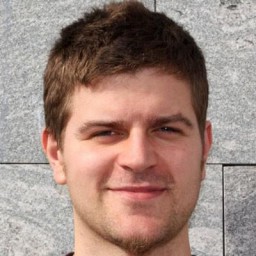
Amazon S3 is a highly scalable and durable cloud storage service that can significantly enhance your PHP applications by providing efficient file exporting capabilities. Whether you're handling user uploads, backups, or serving media content, integrating Amazon S3 into your PHP projects ensures reliable and secure storage. In this comprehensive guide, we walk you through the process of exporting files to Amazon S3 using PHP—covering everything from setup to advanced techniques for large file exports.
Prerequisites and setup
Before we get started, make sure you have the following prerequisites in place:
- PHP version 8.1 or higher (required for AWS SDK compatibility)
- Composer installed
- An AWS account with access to Amazon S3
- AWS SDK for PHP
Installing AWS SDK for PHP
First, create a composer.json
file with the following configuration:
{
"require": {
"aws/aws-sdk-php": "^3.0"
}
}
Then install the AWS SDK using Composer:
composer install
Configuring AWS credentials
For development environments, use environment variables (recommended):
export AWS_ACCESS_KEY_ID=YOUR_AWS_ACCESS_KEY_ID
export AWS_SECRET_ACCESS_KEY=YOUR_AWS_SECRET_ACCESS_KEY
export AWS_DEFAULT_REGION=your-region
For production environments, use IAM roles where possible, especially on AWS services like EC2, ECS, or Lambda.
Creating a simple S3 bucket
When creating an S3 bucket, follow these important guidelines:
- Bucket names must be globally unique across all AWS accounts.
- Names should be between 3 and 63 characters long.
- Use only lowercase letters, numbers, dots, and hyphens.
- Names must start and end with a letter or number.
Create a bucket using the AWS CLI:
aws s3api create-bucket \
--bucket your-bucket-name \
--region your-region \
--create-bucket-configuration LocationConstraint=your-region
Enable recommended security settings:
# Disable acls by enforcing bucket ownership controls
aws s3api put-bucket-ownership-controls \
--bucket your-bucket-name \
--ownership-controls Rules=[{ObjectOwnership=BucketOwnerEnforced}]
# Block public access
aws s3api put-public-access-block \
--bucket your-bucket-name \
--public-access-block-configuration \
BlockPublicAcls=true,IgnorePublicAcls=true,BlockPublicPolicy=true,RestrictPublicBuckets=true
Developing a PHP script to export files
Below is a basic example of uploading a file to S3:
<?php
require 'vendor/autoload.php';
use Aws\S3\S3Client;
use Aws\Exception\AwsException;
try {
$s3Client = new S3Client([
'version' => 'latest',
'region' => getenv('AWS_DEFAULT_REGION'),
]);
$bucket = 'your-bucket-name';
$key = 'path/to/destination/file.txt';
$sourceFile = '/path/to/local/file.txt';
$result = $s3Client->putObject([
'Bucket' => $bucket,
'Key' => $key,
'SourceFile' => $sourceFile,
'ContentType' => mime_content_type($sourceFile),
]);
echo "File uploaded successfully. Location: {$result['ObjectURL']}\n";
} catch (AwsException $e) {
echo "Error: {$e->getMessage()}\n";
echo "AWS Error Code: {$e->getAwsErrorCode()}\n";
echo "Request ID: {$e->getAwsRequestId()}\n";
}
Handling file metadata efficiently
When uploading files, you can include metadata and apply server-side encryption:
$result = $s3Client->putObject([
'Bucket' => $bucket,
'Key' => $key,
'SourceFile' => $sourceFile,
'ContentType' => mime_content_type($sourceFile),
'Metadata' => [
'original-filename' => basename($sourceFile),
'upload-date' => date('c'),
],
'ServerSideEncryption' => 'AES256',
'CacheControl' => 'max-age=86400',
]);
Testing and debugging your integration
Implement comprehensive logging and error handling using a tool like Monolog:
use Monolog\Logger;
use Monolog\Handler\StreamHandler;
use Aws\S3\Exception\S3Exception;
$logger = new Logger('s3-uploads');
$logger->pushHandler(new StreamHandler(__DIR__ . '/logs/s3-uploads.log', Logger::DEBUG));
try {
$result = $s3Client->putObject([
'Bucket' => $bucket,
'Key' => $key,
'SourceFile' => $sourceFile,
]);
$logger->info('File uploaded successfully', [
'bucket' => $bucket,
'key' => $key,
'location' => $result['ObjectURL'],
]);
} catch (S3Exception $e) {
$logger->error('S3 upload failed', [
'error' => $e->getMessage(),
'code' => $e->getAwsErrorCode(),
'request_id' => $e->getAwsRequestId(),
]);
throw $e;
}
Advanced use cases: multipart uploads
For files larger than 100 MB, use the MultipartUploader class:
use Aws\S3\MultipartUploader;
use Aws\Exception\MultipartUploadException;
try {
$uploader = new MultipartUploader($s3Client, $sourceFile, [
'bucket' => $bucket,
'key' => $key,
'params' => [
'ContentType' => mime_content_type($sourceFile),
],
]);
$result = $uploader->upload();
echo "Upload complete: {$result['ObjectURL']}\n";
} catch (MultipartUploadException $e) {
echo "Multipart upload error: {$e->getMessage()}\n";
}
Security best practices
Follow these best practices to secure your S3 file exporting integration:
- Use IAM roles and policies: Grant the minimum required permissions using IAM roles, and prefer temporary credentials where possible.
- Enable server-side encryption: Use AWS-managed keys (SSE-S3) or customer-managed keys (SSE-KMS), and consider enabling default encryption on your bucket.
- Implement proper access controls: Restrict access with bucket policies, disable legacy ACLs by enforcing bucket ownership controls, and block public access at both the bucket and account levels.
- Monitor and audit activity: Enable S3 access logging, use AWS CloudTrail to track API calls, and configure CloudWatch alerts for suspicious behavior.
- Review your bucket policies, storage classes, and lifecycle settings to optimize costs and manage data effectively.
Troubleshooting common issues
If you encounter issues during the file exporting process, consider the following troubleshooting steps:
- Verify that your AWS credentials and region settings are correct.
- Ensure the bucket name complies with AWS naming rules.
- Confirm that your S3 bucket is configured with proper access policies and ownership settings.
- Check that local file paths and permissions are correct.
- Review AWS SDK logs for detailed error messages.
Conclusion and next steps
By following this guide, you have integrated Amazon S3 file exporting capabilities into your PHP applications using the latest AWS SDK best practices. Continue exploring AWS documentation and SDK features to further enhance your integration and secure your file transfers.
For additional perspectives on advanced file uploading, you may also explore other available solutions.
Happy coding!