File uploads in Angular: a comprehensive guide
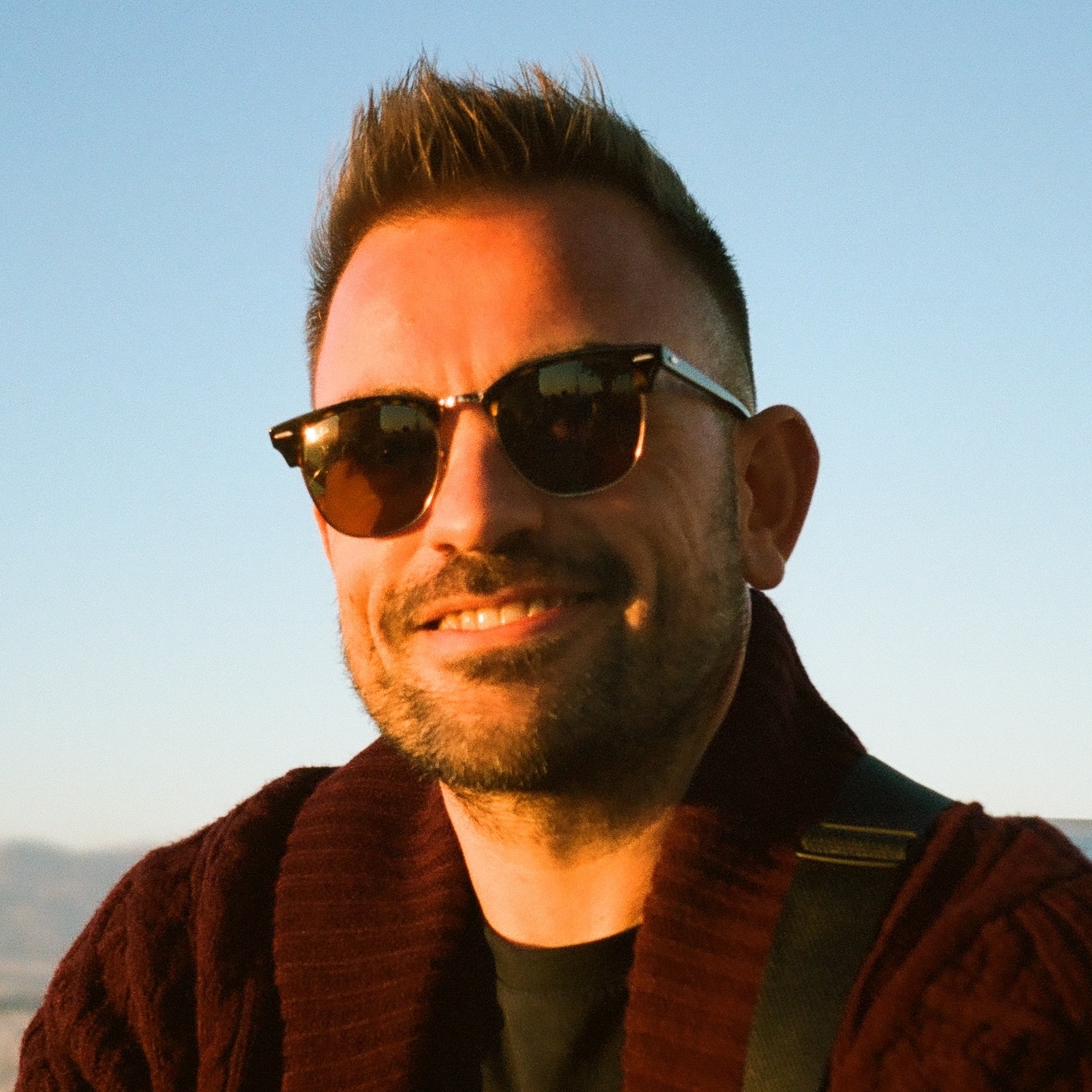
File uploads are a crucial feature in many web applications because they enable users to share documents, images, and other media. In this comprehensive guide, we explore robust file upload functionality in Angular applications—from basic implementations to advanced techniques and best practices. This guide is compatible with Angular 14+ (tested with Angular 17) and uses Angular 17 syntax.
Required setup
Before implementing file uploads, ensure your project has the necessary dependencies. First, import the HttpClientModule in your app.module.ts:
import { NgModule } from '@angular/core'
import { HttpClientModule } from '@angular/common/http'
@NgModule({
imports: [HttpClientModule],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule {}
Understanding the basics of file uploads in Angular
Before diving into implementation details, it is essential to understand the core concepts of file uploads in Angular.
The HTML5 file API
Angular leverages the HTML5 File API, which provides a standardized way to interact with files in web applications. This API allows us to access file information and content directly in the browser.
Angular's forms module
Angular's Forms module plays a crucial role in handling file uploads by providing directives and services that simplify working with form controls, including file inputs.
Implementing basic file uploads
Let's start with a simple file upload implementation in Angular.
Setting up the HTML Template
First, create a basic HTML template with a file input and a button to trigger the upload:
<div class="upload-container">
<input type="file" (change)="onFileSelected($event)" accept=".jpg,.jpeg,.png,.pdf" />
<button [disabled]="!selectedFile" (click)="onUpload()">Upload</button>
<div *ngIf="uploadProgress > 0" class="progress">Upload Progress: %</div>
</div>
Handling file selection in the component
Next, implement the file selection logic with type validation in your component:
import { Component } from '@angular/core'
import { HttpClient, HttpEventType, HttpEvent, HttpErrorResponse } from '@angular/common/http'
import { throwError } from 'rxjs'
import { catchError } from 'rxjs/operators'
@Component({
selector: 'app-file-upload',
templateUrl: './file-upload.component.html',
styleUrls: ['./file-upload.component.css'],
})
export class FileUploadComponent {
selectedFile: File | null = null
uploadProgress = 0
private allowedTypes = ['image/jpeg', 'image/png', 'application/pdf']
constructor(private http: HttpClient) {}
onFileSelected(event: Event): void {
const input = event.target as HTMLInputElement
const file = input.files?.[0]
if (file && this.allowedTypes.includes(file.type)) {
this.selectedFile = file
} else {
alert('Please select a valid file type (JPEG, PNG, or PDF)')
input.value = ''
this.selectedFile = null
}
}
onUpload(): void {
if (this.selectedFile) {
const formData = new FormData()
formData.append('file', this.selectedFile)
this.http
.post('https://api.example.com/upload', formData, {
reportProgress: true,
observe: 'events',
})
.subscribe({
next: (event: HttpEvent<any>) => {
if (event.type === HttpEventType.UploadProgress) {
this.uploadProgress = Math.round(100 * (event.loaded / (event.total || 1)))
} else if (event.type === HttpEventType.Response) {
console.log('Upload complete', event.body)
this.selectedFile = null
setTimeout(() => {
this.uploadProgress = 0
}, 3000)
}
},
error: (err) => {
console.error('Upload failed:', err)
this.uploadProgress = 0
},
})
}
}
private handleError(error: HttpErrorResponse) {
if (error.error instanceof ErrorEvent) {
console.error('Client error:', error.error.message)
} else {
console.error(`Server error: ${error.status}, body was: ${error.error}`)
}
return throwError(() => new Error('Upload failed; please try again later.'))
}
}
Best practices for file uploads in Angular
To ensure a smooth and secure file upload experience, consider the following best practices:
- Validate file types and sizes: Implement client-side checks to prevent uploads of unsupported file types or files that exceed size limits.
- Use chunked uploads: For larger files, implement chunked uploads to enhance performance and reliability.
- Implement retry logic: Add retry mechanisms for failed uploads to handle network issues gracefully.
- Secure your endpoints: Ensure that server-side upload endpoints validate incoming files appropriately.
- Optimize for performance: Use techniques such as compression and lazy loading to improve application performance.
- Provide clear feedback: Keep users informed about the upload status with progress indicators and clear error messages.
- Handle multiple file uploads: Enable functionality to manage multiple file selections and simultaneous uploads.
Using Uppy with Angular
For an advanced file upload solution, you might consider using Uppy—a modern, modular file uploader. To integrate Uppy with Angular, follow these steps:
- Install the required packages: ```bash npm install @uppy/core @uppy/dashboard @uppy/angular
2. Import the Uppy Angular Dashboard module in your component or module:
```typescript
import { UppyAngularDashboardModule } from '@uppy/angular';
```
3. Configure Uppy according to the official documentation and customize it as needed.
This approach provides a scalable alternative for handling complex file uploads.
## Conclusion
Handling file uploads effectively is essential for building robust and user-friendly web applications. By understanding the fundamentals, implementing advanced techniques, and following best practices, you can create a seamless upload experience in your Angular applications. For advanced functionalities, consider exploring dedicated libraries such as Uppy. Happy coding!