Generate and visualize audio waveforms using Go
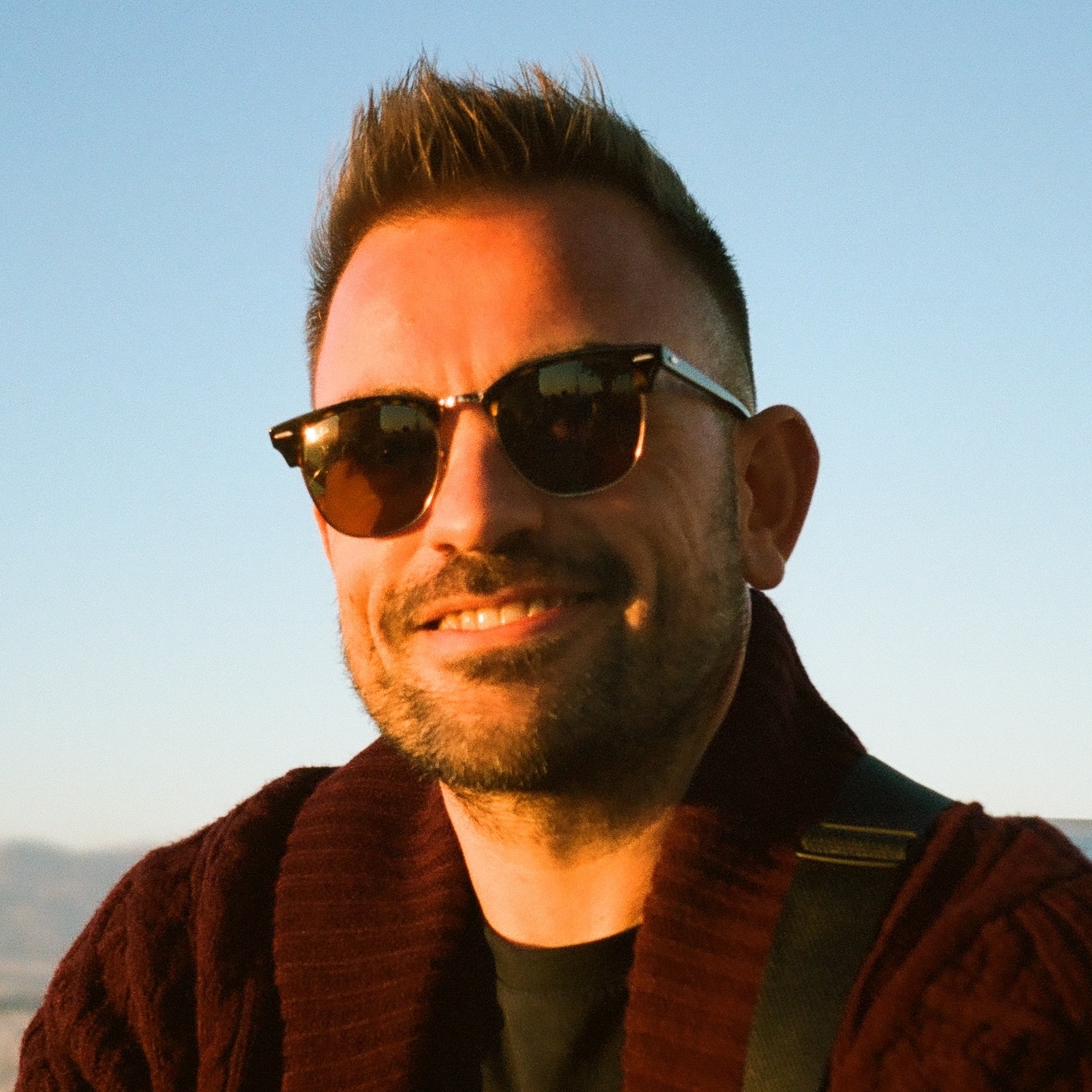
Audio waveform visualization is a powerful tool for developers working with audio data. It provides a visual representation of audio signals, making it easier to analyze, edit, and enhance audio files. In this DevTip, we'll explore how you can generate and visualize audio waveforms using Go, a robust and efficient programming language for audio processing.
Introduction to waveform visualization and its applications
Waveform visualization translates audio signals into graphical representations, typically showing amplitude over time. This technique is widely used in audio editing software, music production, podcasting, and even scientific research. Practical applications include:
- Audio editing and mixing
- Podcast and video production
- Audio analysis and diagnostics
- Interactive audio visualizations for web applications
Setting up your Go environment for audio processing
Dependencies
Before we begin, ensure you have the following dependencies installed:
- Go 1.16 or later
github.com/go-audio/wav
v1.1.0github.com/ajstarks/svgo
v0.0.0-20211024235047-1546f124cd8b
First, ensure you have Go installed. You can verify your installation by running:
go version
Next, initialize a new Go project and install the required dependencies with specific versions for reproducibility:
mkdir waveform-generator
cd waveform-generator
go mod init waveform-generator
go get github.com/go-audio/wav@v1.1.0
go get github.com/ajstarks/svgo@v0.0.0-20211024235047-1546f124cd8b
Generating basic waveforms (sine, square, triangle) with Go
Here's how you can generate a simple sine waveform using Go, including proper error handling and input validation:
package main
import (
"log"
"math"
"os"
"github.com/go-audio/audio"
"github.com/go-audio/wav"
)
func main() {
const sampleRate = 44100
const frequency = 440.0 // A4 note
const duration = 2 // seconds
// Validate input parameters
if sampleRate <= 0 || duration <= 0 || frequency <= 0 {
log.Fatal("Invalid audio parameters: sampleRate, duration, and frequency must be positive.")
}
numSamples := sampleRate * duration
buf := &audio.IntBuffer{
Format: &audio.Format{NumChannels: 1, SampleRate: sampleRate},
Data: make([]int, numSamples),
}
// Generate sine wave
amplitude := 32767.0 // Max amplitude for 16-bit audio
for i := 0; i < numSamples; i++ {
time := float64(i) / float64(sampleRate)
buf.Data[i] = int(amplitude * math.Sin(2*math.Pi*frequency*time))
}
// Create output file with proper error handling
f, err := os.Create("sine.wav")
if err != nil {
log.Fatalf("Failed to create output file 'sine.wav': %v", err)
}
// Use defer with a closure to check for close errors
defer func() {
if err := f.Close(); err != nil {
log.Printf("Warning: Failed to close output file 'sine.wav': %v", err)
}
}()
// Encode audio data to WAV format
// Parameters: output io.Writer, sample rate, bit depth, num channels, audio format (1 = PCM)
encoder := wav.NewEncoder(f, sampleRate, 16, 1, 1)
if err := encoder.Write(buf); err != nil {
log.Fatalf("Failed to write audio data to WAV file: %v", err)
}
// Close the encoder to finalize the WAV file
if err := encoder.Close(); err != nil {
log.Fatalf("Failed to close WAV encoder: %v", err)
}
log.Println("Successfully generated sine.wav")
}
To generate a square wave, modify the waveform generation loop like this:
// Generate square wave
amplitude := 32767.0
for i := 0; i < numSamples; i++ {
time := float64(i) / float64(sampleRate)
if math.Sin(2*math.Pi*frequency*time) >= 0 {
buf.Data[i] = int(amplitude)
} else {
buf.Data[i] = int(-amplitude)
}
}
For a triangle wave, use this loop:
// Generate triangle wave
amplitude := 32767.0
period := float64(sampleRate) / frequency
for i := 0; i < numSamples; i++ {
// Calculate phase within the period (0.0 to 1.0)
phase := math.Mod(float64(i), period) / period
if phase < 0.5 {
// Rising edge: scales from -1 to 1 over the first half
buf.Data[i] = int(amplitude * (phase*4.0 - 1.0))
} else {
// Falling edge: scales from 1 to -1 over the second half
buf.Data[i] = int(amplitude * (3.0 - phase*4.0))
}
}
Visualizing waveforms using SVG and go's image packages
Now, let's visualize the generated waveform (or any WAV file) as an SVG image. This example includes error handling and a note on memory usage for large files.
package main
import (
"fmt"
"log"
"os"
"github.com/ajstarks/svgo"
"github.com/go-audio/wav"
)
func main() {
// Open the WAV file
file, err := os.Open("sine.wav") // Or any other .wav file
if err != nil {
log.Fatalf("Failed to open audio file: %v", err)
}
defer func() {
if err := file.Close(); err != nil {
log.Printf("Warning: Failed to close audio file: %v", err)
}
}()
// Create a new decoder
decoder := wav.NewDecoder(file)
if !decoder.IsValidFile() {
log.Fatal("Invalid WAV file provided.")
}
// Read the full PCM buffer
// Note: For large audio files, consider processing the audio in chunks
// rather than loading the entire file into memory with FullPCMBuffer().
buf, err := decoder.FullPCMBuffer()
if err != nil {
log.Fatalf("Failed to read audio data from WAV file: %v", err)
}
// Define SVG dimensions
width := 800
height := 200
centerY := height / 2
// Create SVG file
svgFile, err := os.Create("waveform.svg")
if err != nil {
log.Fatalf("Failed to create SVG file 'waveform.svg': %v", err)
}
defer func() {
if err := svgFile.Close(); err != nil {
log.Printf("Warning: Failed to close SVG file 'waveform.svg': %v", err)
}
}()
// Initialize SVG canvas
canvas := svg.New(svgFile)
canvas.Start(width, height)
canvas.Rect(0, 0, width, height, "fill:white") // Background
// Draw center line
canvas.Line(0, centerY, width, centerY, "stroke:#cccccc;stroke-width:1")
// Calculate step to fit waveform data into SVG width
numSamples := len(buf.Data)
if numSamples == 0 {
log.Println("Audio buffer is empty, cannot generate waveform.")
canvas.End()
return // Exit if no data
}
// Determine how many samples correspond to one pixel width
step := numSamples / width
if step <= 0 {
step = 1 // Ensure step is at least 1, especially for short audio clips
}
// Draw waveform lines from center to amplitude
maxAmplitude := 32767.0 // For 16-bit audio
scale := float64(centerY) / maxAmplitude // Scale factor for amplitude to fit height
for x := 0; x < width; x++ {
idx := x * step
if idx >= numSamples {
break // Stop if we've processed all samples
}
sampleValue := float64(buf.Data[idx])
// Calculate y coordinate, inverting for SVG (0 is top)
y := centerY - int(sampleValue*scale)
canvas.Line(x, centerY, x, y, "stroke:#0066cc;stroke-width:1")
}
canvas.End()
log.Println("Successfully generated waveform.svg")
}
Advanced waveform customization techniques
You can enhance your SVG visualizations with these techniques:
Adjusting colors and line thickness
Modify the SVG drawing part to use custom styles:
// Define custom colors and styles
backgroundColor := "#f5f5f5"
waveformColor := "#2a9d8f"
centerLineColor := "#e76f51"
waveformStrokeWidth := 2
// Apply to SVG
canvas.Rect(0, 0, width, height, "fill:"+backgroundColor)
canvas.Line(0, centerY, width, centerY, "stroke:"+centerLineColor+";stroke-width:1")
// Draw waveform with custom style
for x := 0; x < width; x++ {
idx := x * step
if idx >= numSamples { break }
sampleValue := float64(buf.Data[idx])
y := centerY - int(sampleValue*scale)
// Use fmt.Sprintf for cleaner style string construction
style := fmt.Sprintf("stroke:%s;stroke-width:%d", waveformColor, waveformStrokeWidth)
canvas.Line(x, centerY, x, y, style)
}
Creating a filled waveform
Instead of drawing individual lines, draw a polygon for an area chart style visualization:
// Draw a filled waveform (area chart style)
fillColor := "#2a9d8f"
fillOpacity := 0.5
// Collect points for the polygon (top edge)
// Allocate slice capacity for efficiency
polyPoints := make([]int, 0, width*2+4) // Top points + start/end points on center line
polyPoints = append(polyPoints, 0, centerY) // Start at (0, center Y)
lastX := 0
for x := 0; x < width; x++ {
idx := x * step
if idx >= numSamples {
break // Stop if we run out of samples
}
sampleValue := float64(buf.Data[idx])
y := centerY - int(sampleValue*scale)
polyPoints = append(polyPoints, x, y) // Add point along the waveform top edge
lastX = x
}
// Add the final point on the center line to close the shape
polyPoints = append(polyPoints, lastX, centerY)
// Draw the polygon
style := fmt.Sprintf("fill:%s;fill-opacity:%.2f;stroke:none", fillColor, fillOpacity)
canvas.Polygon(polyPoints, style)
Practical use cases and project ideas
Here are some ideas to integrate waveform visualization into your Go projects:
- Audio editing tools: Display waveforms to help users identify and edit specific parts of audio files.
- Podcast hosting platforms: Show episode waveforms to help listeners navigate content visually.
- Interactive music players: Create responsive visualizations that react to the music being played.
- Educational tools: Visualize different waveforms (sine, square, triangle, sawtooth) to teach audio engineering concepts.
- Speech analysis applications: Identify patterns like pauses or volume changes in speech recordings through waveform analysis.
Conclusion and further resources
Waveform generation and visualization in Go are straightforward and powerful, enabling you to create detailed audio visualizations quickly. The combination of Go's performance with SVG's flexibility makes it an excellent choice for various audio processing applications.
For further exploration, consider these resources:
If you need to generate waveforms from various audio formats at scale, consider using a dedicated service. Transloadit's 🤖 /audio/waveform Robot supports multiple output formats (image or JSON), customizable dimensions (width/height), background and waveform colors, and anti-aliasing options as part of our Audio Encoding service. This Robot can streamline waveform generation in your production workflows.