Harnessing Python for versatile audio encoding
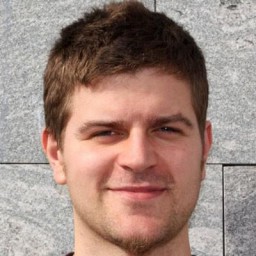
Audio encoding is essential in many Python applications, from web development to data analysis. In this guide, we explore how to effectively encode audio in Python, leveraging powerful libraries like Pydub (version 0.25.1) and tools like FFmpeg for versatile audio processing tasks.
Introduction to audio encoding in Python
Python offers robust capabilities for audio processing, making tasks like converting between audio formats, optimizing audio quality, and batch processing straightforward. Whether you are working on a media application, a podcast platform, or processing audio data, Python's libraries have you covered.
Setting up your environment
Before you start encoding audio, set up your development environment with the necessary tools.
Installing Pydub
Pydub is a high-level audio manipulation library that simplifies working with audio files. This guide uses pydub version 0.25.1.
# Create a virtual environment (recommended)
python -m venv venv
# On macos/linux:
source venv/bin/activate
# On Windows:
venv\Scripts\activate
# Install Pydub (version 0.25.1 for compatibility)
pip install pydub==0.25.1
Installing FFmpeg
FFmpeg is required for Pydub to handle audio file conversions. Install FFmpeg as follows:
For Ubuntu/Debian:
sudo apt update
sudo apt install ffmpeg
For macOS:
brew install ffmpeg
For Windows:
- Download the latest FFmpeg build from the official FFmpeg website.
- Extract the ZIP file.
- Add the
bin
folder to your system's PATH environment variable. - Verify installation:
ffmpeg -version
Dependencies
Required
- Python 3.6+.
- FFmpeg or Libav.
- pydub 0.25.1.
Optional (for playback)
- simpleaudio (recommended).
- pyaudio (alternative).
Understanding popular audio formats
Python can handle various audio formats through FFmpeg:
- WAV: Uncompressed, high-quality audio.
- MP3: Compressed format, offering a good quality-to-size ratio.
- AAC: Advanced Audio Coding, used widely in streaming.
- OGG: Open-source alternative to MP3.
- FLAC: Lossless compression format.
Encoding audio with Pydub and FFmpeg
Converting wav to mp3
Below is a practical example of converting a WAV file to MP3 using Pydub with comprehensive error handling:
import logging
from pydub import AudioSegment
from pydub.exceptions import CouldntDecodeError
# Configure logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def convert_to_mp3(input_path, output_path):
try:
# Load the audio file (WAV format)
audio = AudioSegment.from_wav(input_path)
# Export as MP3 with specified settings
audio.export(
output_path,
format='mp3',
bitrate='192k',
parameters=[
'-codec:a', 'libmp3lame',
'-qscale:a', '2'
]
)
logger.info(f'Successfully converted {input_path} to MP3')
return True
except CouldntDecodeError:
logger.error(f'Failed to decode {input_path}. Ensure it is a valid WAV file.')
return False
except Exception as e:
logger.error(f'Error converting file: {str(e)}')
return False
# Usage example
if __name__ == "__main__":
result = convert_to_mp3('input.wav', 'output.mp3')
if result:
print('Conversion successful.')
else:
print('Conversion failed.')
Advanced audio processing
Below is an example of a comprehensive audio processing function that includes normalization, volume adjustment, and fade effects:
import os
import logging
from pydub import AudioSegment
from pydub.effects import normalize
# Configure logging
logger = logging.getLogger(__name__)
def process_audio(input_path, output_path, **kwargs):
try:
# Verify that the input file exists
if not os.path.exists(input_path):
raise FileNotFoundError(f"Input file not found: {input_path}")
# Load the audio file
audio = AudioSegment.from_file(input_path)
# Apply audio processing based on parameters
if kwargs.get('normalize', False):
audio = normalize(audio)
if kwargs.get('volume_boost'):
# Increase volume by a specified number of decibels
audio = audio.apply_gain(kwargs['volume_boost'])
if kwargs.get('fade_in'):
audio = audio.fade_in(kwargs['fade_in'])
if kwargs.get('fade_out'):
audio = audio.fade_out(kwargs['fade_out'])
# Export the processed audio with specified format and quality
audio.export(
output_path,
format=kwargs.get('format', 'mp3'),
bitrate=kwargs.get('bitrate', '192k')
)
return True
except Exception as e:
logger.error(f'Error processing audio: {str(e)}')
return False
# Example usage with various effects
if __name__ == "__main__":
success = process_audio(
'input.wav',
'output.mp3',
normalize=True,
volume_boost=5, # Increase volume by 5 dB
fade_in=2000, # 2 seconds fade-in
fade_out=3000, # 3 seconds fade-out
format='mp3',
bitrate='320k'
)
if success:
print('Audio processing completed successfully.')
else:
print('Audio processing failed.')
Batch processing audio files
Processing multiple audio files can be done efficiently using multithreading. This example demonstrates how to convert all WAV files in a directory to MP3 concurrently:
import os
import logging
from concurrent.futures import ThreadPoolExecutor
from pydub import AudioSegment
logger = logging.getLogger(__name__)
def batch_convert(input_dir, output_dir, max_workers=4):
if not os.path.exists(output_dir):
os.makedirs(output_dir)
def process_file(filename):
if filename.endswith('.wav'):
input_path = os.path.join(input_dir, filename)
output_filename = f"{os.path.splitext(filename)[0]}.mp3"
output_path = os.path.join(output_dir, output_filename)
try:
audio = AudioSegment.from_wav(input_path)
audio.export(output_path, format='mp3', bitrate='192k')
logger.info(f'Converted {filename} to {output_filename}')
except Exception as e:
logger.error(f'Error processing {filename}: {str(e)}')
with ThreadPoolExecutor(max_workers=max_workers) as executor:
files = os.listdir(input_dir)
executor.map(process_file, files)
# Usage example
if __name__ == "__main__":
batch_convert('wav_files', 'mp3_files', max_workers=4)
Error handling and quality control
Implementing robust error handling ensures your application can gracefully manage unexpected issues. In the example below, we retrieve metadata using FFmpeg and perform basic validation:
from pydub.utils import mediainfo
import logging
logger = logging.getLogger(__name__)
def validate_audio(file_path):
try:
# Get audio file information
info = mediainfo(file_path)
# Check basic audio properties
duration = float(info['duration'])
if duration < 0.1: # Less than 100ms
raise ValueError('Audio file too short')
# Check audio quality
bit_rate = int(info['bit_rate'])
if bit_rate < 128000: # Less than 128kbps
logger.warning('Low bit rate detected')
# Verify channel configuration
channels = int(info['channels'])
if channels not in [1, 2]:
logger.warning('Unusual channel configuration')
return True
except Exception as e:
logger.error(f'Validation failed: {str(e)}')
return False
Optimizing audio quality
You can optimize audio quality using different presets tailored to various use cases. The following example demonstrates how to apply normalization and dynamic range compression:
from pydub import AudioSegment
from pydub.effects import normalize, compress_dynamic_range
import logging
logger = logging.getLogger(__name__)
def optimize_audio(input_path, output_path, quality_preset='high'):
presets = {
'high': {
'bitrate': '320k',
'normalize': True,
'compression': False
},
'streaming': {
'bitrate': '192k',
'normalize': True,
'compression': True
},
'mobile': {
'bitrate': '128k',
'normalize': True,
'compression': True
}
}
try:
audio = AudioSegment.from_file(input_path)
settings = presets.get(quality_preset, presets['high'])
if settings['normalize']:
audio = normalize(audio)
if settings['compression']:
audio = compress_dynamic_range(audio)
audio.export(
output_path,
format='mp3',
bitrate=settings['bitrate']
)
logger.info(f'Audio optimized with {quality_preset} preset.')
return True
except Exception as e:
logger.error(f'Error optimizing audio: {str(e)}')
return False
# Usage example
if __name__ == "__main__":
optimize_audio('input.wav', 'output.mp3', quality_preset='streaming')
Troubleshooting common issues
If you encounter issues during audio processing, consider the following steps:
- Ensure FFmpeg is correctly installed and added to your system's PATH.
- Confirm that the input audio file exists and is not corrupted.
- Check log messages for detailed error information.
- For batch processing, verify file permissions and system resource availability.
Conclusion
Python, combined with FFmpeg and Pydub, provides powerful tools for audio encoding and processing. Whether you are converting formats, adjusting audio properties, or processing files in bulk, Python makes these tasks accessible and efficient. For large-scale or more complex audio processing needs, consider using Transloadit's audio encoding service, which offers advanced features and scalable infrastructure to enhance your audio toolset.