How to resize and watermark images in PHP using Imagick
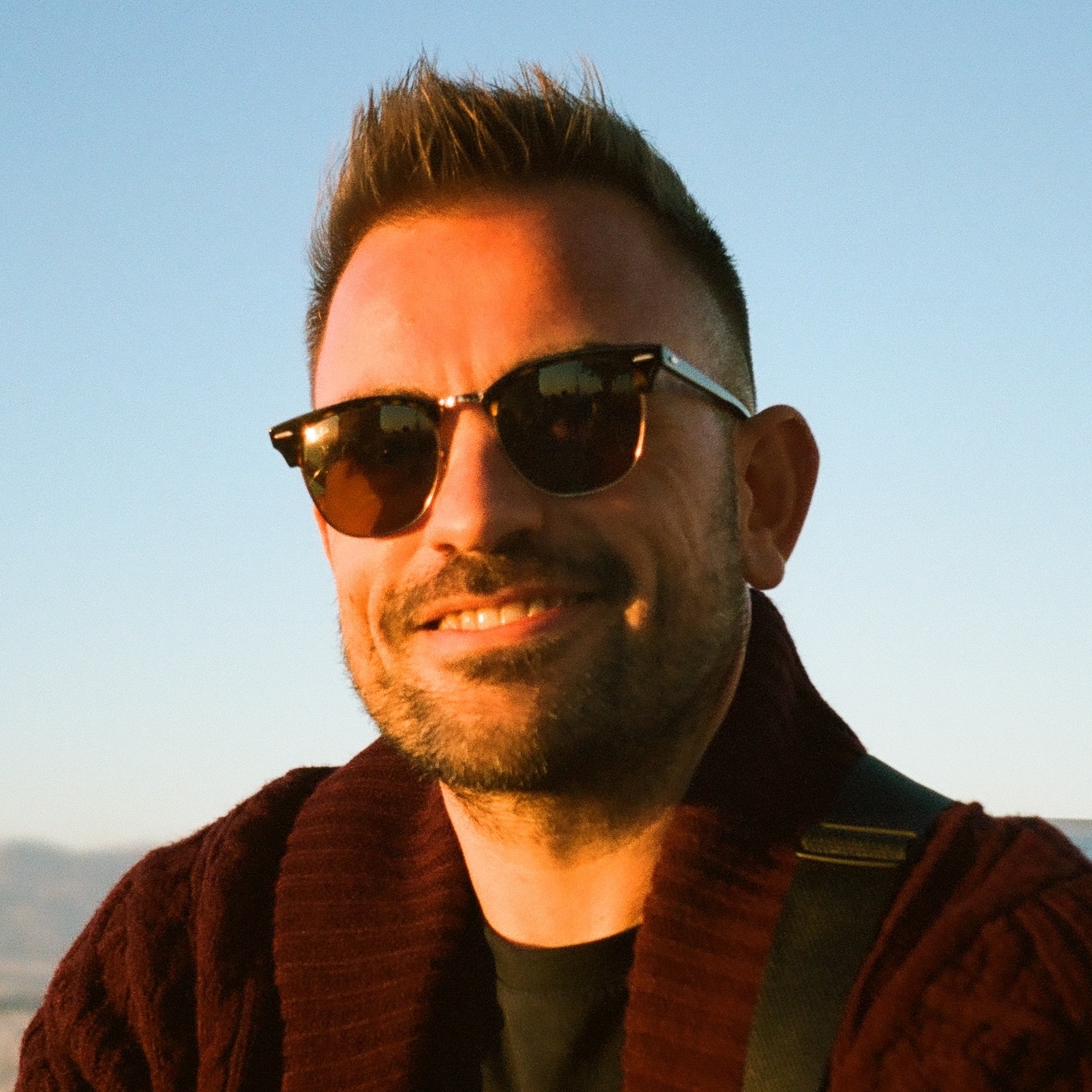
Imagick is a robust PHP extension that wraps the ImageMagick library, enabling developers to efficiently create, modify, and convert images. This tutorial demonstrates how to resize images and apply watermarks using Imagick, helping you enhance your web application's image processing capabilities.
Introduction to Imagick and its benefits
Imagick is an open-source PHP extension providing an object-oriented API for image manipulation. Leveraging the powerful ImageMagick library, it supports a wide range of image formats and advanced image processing features.
PHP version compatibility
Imagick is compatible with:
- PHP 7.x
- PHP 8.0, 8.1, 8.2, 8.3
Advantages of using Imagick
- Wide Format Support: Supports over 200 image formats, including JPEG, PNG, GIF, and more.
- Advanced Processing: Offers robust features for resizing, cropping, rotating, and adding effects.
- Performance: Efficiently handles large images and executes complex operations quickly.
- Active Development: Continuously updated by a large community.
- Open Source: Freely available with community contributions.
Installing and setting up Imagick
Before using Imagick, install both the ImageMagick software and the Imagick PHP extension.
Installation on Ubuntu/Debian (PHP 8.x)
# Ubuntu/Debian (PHP 8.x)
sudo apt-get update
sudo apt-get install php-imagick imagemagick
Installation on macOS
brew install imagemagick
brew install php
pecl install imagick
After installation, ensure the extension is enabled by adding the following line to your php.ini
:
extension=imagick.so
Restart your web server to apply the changes.
Security considerations
When working with Imagick, implement these security measures:
<?php
// Validate image MIME types
$allowedMimeTypes = ['image/jpeg', 'image/png', 'image/gif'];
if (!in_array($image->getImageMimeType(), $allowedMimeTypes)) {
throw new Exception('Invalid image type');
}
// Set resource limits to prevent DOS attacks
$image->setResourceLimit(Imagick::RESOURCE_MEMORY, 256);
$image->setResourceLimit(Imagick::RESOURCE_DISK, 1024);
Resizing images using Imagick: a step-by-step tutorial
The following example demonstrates how to securely resize images using Imagick:
<?php
try {
$image = new Imagick($imagePath);
// Ensure the file is a valid image
if (!$image->valid()) {
throw new Exception('Invalid image');
}
// Validate MIME type
$allowedMimeTypes = ['image/jpeg', 'image/png', 'image/gif'];
if (!in_array($image->getImageMimeType(), $allowedMimeTypes)) {
throw new Exception('Invalid image type');
}
// Resize the image to a width of 800px while maintaining the aspect ratio
$image->resizeImage(800, 0, Imagick::FILTER_LANCZOS, 1, true);
// Strip metadata for enhanced security and performance
$image->stripImage();
$image->writeImage($outputPath);
} catch (ImagickException $e) {
error_log('Imagick error: ' . $e->getMessage());
throw new Exception('Image processing failed');
} finally {
if (isset($image)) {
$image->clear();
$image->destroy();
}
}
Adding watermarks to images with Imagick
This secure implementation demonstrates how to add watermarks to images:
<?php
try {
$image = new Imagick($imagePath);
// Ensure the file is a valid image
if (!$image->valid()) {
throw new Exception('Invalid image');
}
// Create watermark text
$draw = new ImagickDraw();
$draw->setFillColor('gray');
$draw->setFont('Arial');
$draw->setFontSize(30);
$draw->setGravity(Imagick::GRAVITY_SOUTHEAST);
// Add watermark text with a slight opacity effect
$image->annotateImage($draw, 10, 12, 0, '© Your Company');
$image->evaluateImage(Imagick::EVALUATE_MULTIPLY, 0.5, Imagick::CHANNEL_ALPHA);
$image->writeImage($outputPath);
} catch (ImagickException $e) {
error_log('Imagick error: ' . $e->getMessage());
throw new Exception('Watermark application failed');
} finally {
if (isset($image)) {
$image->clear();
$image->destroy();
}
}
Performance optimization
Optimize your processed images for web performance using these adjustments:
<?php
try {
$image = new Imagick($imagePath);
// Set compression quality for larger images
if ($image->getImageLength() > 1024 * 1024) {
$image->setImageCompressionQuality(85);
}
// Enable progressive JPEG for enhanced web loading
$image->setInterlaceScheme(Imagick::INTERLACE_PLANE);
// Convert image format to WebP for improved compression
$image->setImageFormat('webp');
// Remove metadata to reduce file size
$image->stripImage();
$image->writeImage($outputPath);
} catch (ImagickException $e) {
error_log('Imagick error: ' . $e->getMessage());
throw new Exception('Image optimization failed');
} finally {
if (isset($image)) {
$image->clear();
$image->destroy();
}
}
Combining operations for efficient workflows
Combine resizing, watermarking, and optimization into a single, efficient process:
<?php
try {
$image = new Imagick($imagePath);
// Validate the image
if (!$image->valid()) {
throw new Exception('Invalid image');
}
// Resize the image
$image->resizeImage(800, 0, Imagick::FILTER_LANCZOS, 1, true);
// Apply watermark text
$draw = new ImagickDraw();
$draw->setFillColor('gray');
$draw->setFont('Arial');
$draw->setFontSize(20);
$draw->setGravity(Imagick::GRAVITY_SOUTHEAST);
$image->annotateImage($draw, 10, 12, 0, '© Your Company');
// Optimize the image for the web
$image->stripImage();
$image->setImageCompressionQuality(85);
$image->setInterlaceScheme(Imagick::INTERLACE_PLANE);
$image->writeImage($outputPath);
} catch (ImagickException $e) {
error_log('Imagick error: ' . $e->getMessage());
throw new Exception('Image processing failed');
} finally {
if (isset($image)) {
$image->clear();
$image->destroy();
}
}
Best practices
When working with Imagick, adhere to these best practices:
- Use comprehensive error handling with try-catch blocks.
- Validate input images and MIME types rigorously.
- Set resource limits to prevent denial-of-service attacks.
- Favor progressive image loading for web performance.
- Strip metadata to reduce file sizes and enhance security.
- Free resources by calling
clear()
anddestroy()
on Imagick objects. - Consider converting images to the WebP format for improved compression.
- Configure appropriate file permissions and access controls.
- Log errors effectively to aid in troubleshooting.
By following these strategies and leveraging Imagick's robust features, you can create efficient image processing workflows in your PHP applications.
At Transloadit, we use robust image processing tools to manage complex image manipulations efficiently. For scalable solutions in handling images and media files, explore our Image Manipulation service.