Pixelate faces: Ruby & ImageMagick
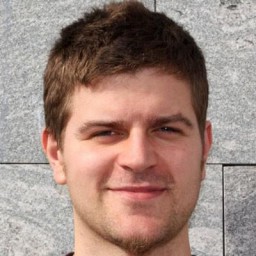
Face pixelation is a powerful technique to enhance privacy by obscuring identifiable features in images. Whether you're building a social media app, a surveillance system, or simply want to anonymize images, automating face pixelation can be incredibly useful.
Why pixelate faces?
Face pixelation helps protect individual privacy by making faces unrecognizable. Practical applications include:
- Social media platforms anonymizing user-uploaded images
- Surveillance footage anonymization
- Compliance with privacy regulations like GDPR
- Protecting minors in public images
- Anonymizing research data containing human subjects
Setting up Ruby and ImageMagick
First, ensure Ruby and ImageMagick are installed on your system:
# For macOS
brew install imagemagick
gem install rmagick google-cloud-vision
# For Ubuntu/Debian
sudo apt-get install imagemagick libmagickwand-dev
gem install rmagick google-cloud-vision
Setting up Google cloud vision API
For face detection, we'll use Google Cloud Vision API, which provides accurate and reliable face detection capabilities.
1. Create a Google cloud project
- Go to the Google Cloud Console.
- Create a new project or select an existing one.
- Make note of your project ID.
2. Enable the vision API
- Navigate to "APIs & Services" > "Library".
- Search for "Vision API" and enable it.
3. Set up authentication
- Go to "APIs & Services" > "Credentials".
- Click "Create credentials" > "Service account".
- Fill in the service account details and grant it the "Cloud Vision API User" role.
- Create a JSON key for this service account and download it.
- Store this key securely on your system.
4. Set the environment variable
export GOOGLE_APPLICATION_CREDENTIALS="/path/to/your-project-credentials.json"
Integrating face detection with Ruby using Google cloud vision API
Now let's create a function to detect faces in images:
require "google/cloud/vision"
def detect_faces(image_path)
# Initialize the Vision client
vision = Google::Cloud::Vision.image_annotator
# Perform face detection
response = vision.face_detection(
image: image_path,
max_results: 10 # You can adjust the maximum number of faces to detect
)
# Extract face coordinates
faces = []
response.responses.each do |res|
res.face_annotations.each do |face|
vertices = face.bounding_poly.vertices
# Calculate face rectangle
x = vertices[0].x
y = vertices[0].y
width = vertices[2].x - x
height = vertices[2].y - y
faces << { x: x, y: y, width: width, height: height }
end
end
faces
end
Applying pixelation effects with ImageMagick
Using RMagick, we can pixelate the detected face regions:
require 'rmagick'
def pixelate_faces(image_path, faces, pixelation_factor = 0.1)
img = Magick::Image.read(image_path).first
faces.each do |face|
# Extract face region
face_region = img.crop(face[:x], face[:y], face[:width], face[:height])
# Pixelate by scaling down and back up
pixelated = face_region.scale(pixelation_factor).scale(face[:width], face[:height])
# Composite the pixelated face back onto the original image
img.composite!(pixelated, face[:x], face[:y], Magick::OverCompositeOp)
end
img.write('pixelated_output.jpg')
puts "Image with pixelated faces saved as 'pixelated_output.jpg'"
return img
end
Step-by-step Ruby code example
Here's a complete example combining detection and pixelation with proper error handling:
require "google/cloud/vision"
require 'rmagick'
def pixelate_image(image_path, output_path = 'pixelated_output.jpg', pixelation_factor = 0.1)
begin
# Detect faces
faces = detect_faces(image_path)
if faces.empty?
puts "No faces detected in the image."
return false
end
# Pixelate faces
img = Magick::Image.read(image_path).first
faces.each do |face|
face_region = img.crop(face[:x], face[:y], face[:width], face[:height])
pixelated = face_region.scale(pixelation_factor).scale(face[:width], face[:height])
img.composite!(pixelated, face[:x], face[:y], Magick::OverCompositeOp)
end
img.write(output_path)
puts "Successfully pixelated #{faces.count} faces in '#{output_path}'"
return true
rescue Google::Cloud::Error => e
puts "Vision API error: #{e.message}"
rescue Magick::ImageMagickError => e
puts "ImageMagick error: #{e.message}"
rescue StandardError => e
puts "Unexpected error: #{e.message}"
end
return false
end
# Usage
pixelate_image('input.jpg', 'output.jpg')
Optimizing performance and handling edge cases
API considerations
- Rate limits: Google Cloud Vision API has quotas (1,000 requests per minute by default).
- Image size: Maximum file size is 20MB per image.
- Batch processing: For multiple images, use batch requests to reduce API calls.
- Cost management: Monitor usage to stay within budget constraints.
Handling edge cases
- No faces detected: Add fallback logic when no faces are found.
- Low confidence detections: Filter results by confidence score.
- Partial faces: Adjust bounding box handling for faces at image edges.
# Filter faces by confidence score
faces = response.responses.first.face_annotations.select do |face|
face.detection_confidence > 0.7 # Only keep faces with >70% confidence
end
Alternative face detection APIs
If Google Cloud Vision doesn't meet your needs, consider these alternatives:
- Amazon Rekognition: Robust face detection with additional features like age estimation.
- Microsoft Azure Face API: Comprehensive facial analysis capabilities.
- Luxand Cloud: Specialized in facial recognition technology.
How does ImageMagick integrate with Ruby for image processing?
ImageMagick integrates with Ruby through the RMagick gem, providing a comprehensive set of image manipulation capabilities. This integration allows developers to:
- Resize, crop, and transform images
- Apply filters and effects
- Composite multiple images
- Convert between image formats
- Extract image metadata
The RMagick library wraps ImageMagick's functionality in a Ruby-friendly API, making complex image operations accessible through simple method calls.
Privacy and GDPR considerations
When implementing face pixelation, consider these privacy best practices:
- Obtain proper consent when processing identifiable images.
- Implement data minimization by only storing necessary information.
- Document your processing activities for compliance purposes.
- Consider whether you need to perform a Data Protection Impact Assessment (DPIA).
- Ensure secure handling of both original and processed images.
Conclusion
Automating face pixelation with Ruby, Google Cloud Vision API, and ImageMagick provides a powerful solution for privacy-focused applications. By combining cloud-based face detection with local image manipulation, you can create efficient and accurate face anonymization workflows.
For more advanced image manipulation needs, consider exploring Transloadit's Image Manipulation Service, which offers powerful tools for processing and transforming images at scale.