Import files from Cloudflare R2 in Java with Rclone
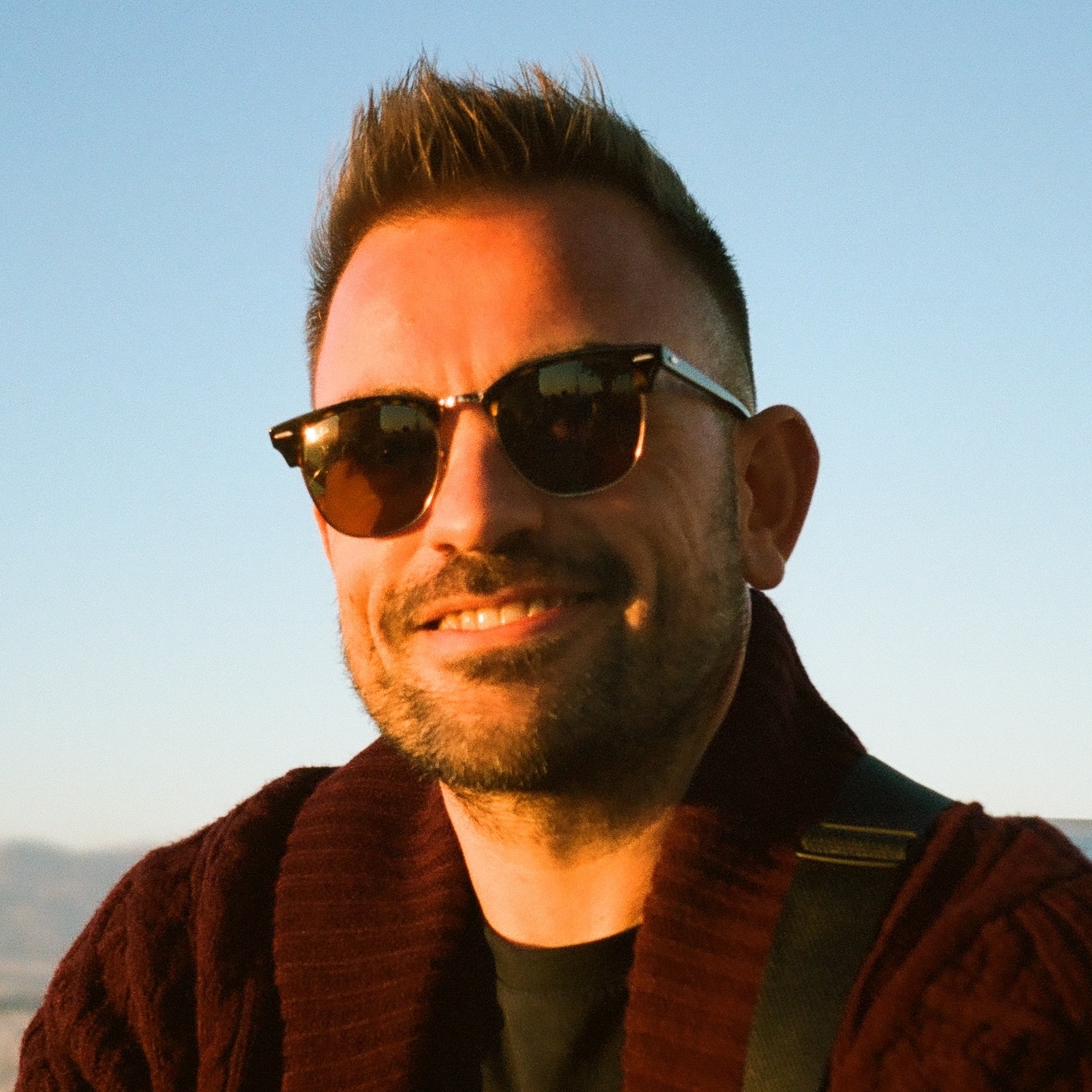
Cloudflare R2 provides developers with a cost-effective, performant, and reliable object storage solution. Integrating it into your Java applications can significantly streamline your file management workflows. In this DevTip, we'll explore how to efficiently import files from Cloudflare R2 using the powerful open-source tool Rclone.
Introduction to Cloudflare R2
Cloudflare R2 is an S3-compatible object storage service designed to eliminate egress fees, making
it ideal for applications requiring frequent data retrieval. Its compatibility with the S3 API
simplifies integration with existing tools and workflows used for file importing
.
Overview of Rclone
Rclone is an open-source command-line tool that synchronizes files and directories to and from
various cloud storage providers. It supports numerous storage backends, including Cloudflare R2, and
provides robust features such as syncing, copying, and mounting remote storage. It's one of the most
popular open-source tools
for cloud storage management.
Setting up Rclone for Cloudflare R2
First, install Rclone if you haven't already. You can usually do this with a single command. After installation, verify it's working:
# Install Rclone (linux/macos/bsd)
curl https://rclone.org/install.sh | sudo bash
# Verify installation
rclone version
For other operating systems or methods, refer to the official Rclone installation guide.
Next, configure Rclone to connect to your Cloudflare R2 bucket using the interactive configuration tool:
# Configure Rclone (interactive)
rclone config
Follow the interactive prompts:
- Choose
n
for a new remote. - Enter a name for your remote (e.g.,
cloudflare_r2
). - Select
s3
(or the corresponding number) as the storage type. - For the provider, select
Cloudflare
(or the corresponding number). - Choose
Enter credentials value here
(usually option1
) or let Rclone find credentials if configured elsewhere (e.g., environment variables). - Provide your Cloudflare R2
Access Key ID
. - Provide your Cloudflare R2
Secret Access Key
. - Set the
Endpoint URL
for your R2 bucket:https://<accountid>.r2.cloudflarestorage.com
(replace<accountid>
with your actual Cloudflare account ID). - You can leave the
Location constraint
blank or set it if needed (oftenauto
works). - Set the
ACL
(Access Control List).private
is a common and secure choice. - Review the advanced configuration options (defaults are often fine) and save the configuration.
Your resulting configuration in the Rclone config file (~/.config/rclone/rclone.conf
by default)
should look similar to this:
[cloudflare_r2]
type = s3
provider = Cloudflare
access_key_id = YOUR_ACCESS_KEY_ID
secret_access_key = YOUR_SECRET_ACCESS_KEY
endpoint = https://<accountid>.r2.cloudflarestorage.com
acl = private
Remember to replace the placeholder values with your actual credentials and account ID, and ensure this configuration file is appropriately secured.
Integrating Rclone with Java applications
Java applications can invoke Rclone commands using the ProcessBuilder
class. This allows you to
leverage Rclone's capabilities directly within your Java
code. Here's a practical example
demonstrating how to import files from Cloudflare R2
:
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.TimeUnit; // For timeout handling
public class RcloneImporter {
/**
* Imports files from a specified Cloudflare R2 path to a local path using Rclone.
*
* @param remoteName The name of the configured Rclone remote (e.g., "cloudflare_r2").
* @param remotePath The path within the R2 bucket (e.g., "my-bucket/path/to/files").
* @param localPath The local directory path where files will be downloaded.
* @throws IOException If an I/O error occurs during process execution.
* @throws InterruptedException If the current thread is interrupted while waiting for the process.
* @throws RuntimeException If the Rclone command fails (non-zero exit code) or times out.
*/
public static void importFiles(String remoteName, String remotePath, String localPath)
throws IOException, InterruptedException {
List<String> command = new ArrayList<>();
command.add("rclone");
command.add("copy"); // Use "sync" for synchronization instead of just copying
command.add(remoteName + ":" + remotePath); // Format: remote:path/to/dir
command.add(localPath); // Destination local directory
// Example: Add flags for parallel transfers and progress
// command.add("--transfers");
// command.add("8");
// command.add("--progress");
System.out.println("Executing Rclone command: " + String.join(" ", command));
ProcessBuilder builder = new ProcessBuilder(command);
builder.redirectErrorStream(true); // Merge error stream with standard output
Process process = builder.start();
// Capture and print process output
try (BufferedReader reader = new BufferedReader(
new InputStreamReader(process.getInputStream()))) {
String line;
while ((line = reader.readLine()) != null) {
// Replace with proper logging in a real application
System.out.println("Rclone Output: " + line);
}
}
// Wait for the process to complete with a timeout
// Adjust timeout value as needed
boolean finished = process.waitFor(10, TimeUnit.MINUTES);
if (!finished) {
process.destroyForcibly();
throw new RuntimeException("Rclone command timed out after 10 minutes.");
}
int exitCode = process.exitValue(); // Use exitValue() after waitFor()
if (exitCode != 0) {
// Consider more specific exception handling based on Rclone exit codes if needed
throw new RuntimeException("Rclone command failed with exit code: " + exitCode);
} else {
System.out.println("Rclone command executed successfully.");
}
}
public static void main(String[] args) {
// Example usage:
String rcloneRemoteName = "cloudflare_r2"; // Matches the name used in `rclone config`
String bucketPath = "my-data-bucket/source-files"; // Path inside your R2 bucket
String localDirectory = "./downloaded-files"; // Local destination directory
try {
// Optional: Ensure the local directory exists
// java.nio.file.Files.createDirectories(java.nio.file.Paths.get(localDirectory));
System.out.println("Starting file import from Cloudflare R2...");
importFiles(rcloneRemoteName, bucketPath, localDirectory);
System.out.println("File import completed successfully.");
} catch (IOException | InterruptedException e) {
System.err.println("Error during Rclone execution: " + e.getMessage());
// Log the exception stack trace for debugging
e.printStackTrace();
// Handle the error appropriately in your application
Thread.currentThread().interrupt(); // Restore interrupted status
} catch (RuntimeException e) {
System.err.println("Rclone command failed or timed out: " + e.getMessage());
// Log the exception stack trace for debugging
e.printStackTrace();
// Handle the error appropriately in your application
}
}
// --- Advanced Usage Examples ---
/**
* Lists object names (files and directories) in a given remote path.
*/
public static List<String> listObjects(String remoteName, String remotePath)
throws IOException, InterruptedException {
List<String> command = List.of("rclone", "lsf", remoteName + ":" + remotePath);
ProcessBuilder builder = new ProcessBuilder(command);
builder.redirectErrorStream(true);
Process process = builder.start();
List<String> objectNames = new ArrayList<>();
try (BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()))) {
String line;
while ((line = reader.readLine()) != null) {
objectNames.add(line.trim());
}
}
boolean finished = process.waitFor(1, TimeUnit.MINUTES); // Shorter timeout for listing
if (!finished) {
process.destroyForcibly();
throw new RuntimeException("Rclone list command timed out.");
}
int exitCode = process.exitValue();
if (exitCode != 0) {
// Rclone might return non-zero if path doesn't exist, handle appropriately
System.err.println("Rclone list command finished with non-zero exit code: " + exitCode);
// Depending on the use case, you might return an empty list or throw
// throw new RuntimeException("Rclone list command failed with exit code: " + exitCode);
}
return objectNames;
}
/**
* Checks if a specific file exists at the given remote path.
*/
public static boolean fileExists(String remoteName, String remoteFilePath)
throws IOException, InterruptedException {
// Using `lsf` on a specific file path is a common way to check existence
List<String> command = List.of("rclone", "lsf", remoteName + ":" + remoteFilePath);
ProcessBuilder builder = new ProcessBuilder(command);
builder.redirectErrorStream(true);
Process process = builder.start();
StringBuilder output = new StringBuilder();
try (BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()))) {
String line;
// Read the first line only, if it exists, the file is there
if ((line = reader.readLine()) != null) {
output.append(line);
}
// Consume rest of output if any
while (reader.readLine() != null) {}
}
boolean finished = process.waitFor(30, TimeUnit.SECONDS); // Timeout for check
if (!finished) {
process.destroyForcibly();
throw new RuntimeException("Rclone file check command timed out.");
}
int exitCode = process.exitValue();
// Rclone lsf returns exit code 0 and the filename if found.
// Non-zero exit code (e.g., 3 for "Directory not found") indicates not found or error.
return exitCode == 0 && !output.toString().trim().isEmpty();
}
}
This improved Java
implementation executes the Rclone command to copy files from your
Cloudflare R2
bucket to a local directory. It includes better process output handling, timeout
management, and more robust error checking.
Common issues and troubleshooting tips
When integrating Rclone with Java
for Cloudflare R2
operations, you might encounter these
issues:
1. Authentication errors
- Incorrect Credentials: Double-check the
access_key_id
andsecret_access_key
in your Rclone configuration or environment variables. - Wrong Endpoint: Ensure the
endpoint
URL is correct and includes your specific Cloudflare account ID (https://<accountid>.r2.cloudflarestorage.com
). - Permissions: Verify that the R2 API token associated with your credentials has the necessary
permissions (e.g.,
Object Read
) for the target bucket and objects. - ACL Settings: Ensure the
acl
setting in your Rclone config (private
,public-read
, etc.) aligns with your bucket policy and access needs.
2. Network issues
- Firewall Restrictions: Ensure your server's firewall allows outbound HTTPS connections
(port 443) to the Cloudflare R2 endpoint (
*.r2.cloudflarestorage.com
). - Connectivity: Verify general network connectivity from the machine running the Java
application to Cloudflare's services (e.g., using
ping
orcurl
).
3. Performance optimization
- Parallel Transfers: Use the
--transfers N
flag (e.g.,--transfers 8
) in your Rclone command to perform multiple file transfers concurrently, significantly speeding up operations with many small files. Add this to thecommand
list in the Java code. - Chunk Size: For very large files, experiment with
--s3-chunk-size SIZE
(e.g.,--s3-chunk-size 64M
) to optimize multipart uploads/downloads. - Bandwidth Limit: If needed, use
--bwlimit RATE
(e.g.,--bwlimit 10M
for 10 MBytes/sec) to control bandwidth usage.
4. Java-specific issues
- Rclone Not Found: Ensure the
rclone
executable is in the system'sPATH
environment variable accessible by the Java process, or provide the full path to the executable in theProcessBuilder
command list. - Process Handling: Implement proper handling for the process input/output streams (as shown in
the example) to avoid blocking. Use
redirectErrorStream(true)
to capture errors. - Timeouts: Implement process timeouts using
process.waitFor(timeout, unit)
as shown in the example code to prevent indefinite hangs. Adjust the timeout duration based on expected operation time. - Resource Cleanup: Ensure
Process
resources are handled correctly, especially in long-running applications. Thetry-with-resources
for theBufferedReader
helps, and ensuring the process terminates (viawaitFor
ordestroyForcibly
) is crucial.
Advanced usage examples
Beyond simple copying, you can use Rclone via Java
for other tasks like listing objects or
checking file existence. See the static methods listObjects
and fileExists
within the
RcloneImporter
class example above.
Security best practices
When integrating external tools like Rclone and handling cloud credentials in Java
applications,
prioritize security:
- Avoid Hardcoding Credentials: Never embed your Cloudflare R2
Access Key ID
orSecret Access Key
directly in your source code. - Use Secure Credential Storage:
- Rclone Config File: Let Rclone use its standard configuration file (
rclone.conf
), but ensure the file itself has restricted read permissions (e.g.,chmod 600 ~/.config/rclone/rclone.conf
). This is often the simplest approach. - Environment Variables: Configure Rclone to read credentials from environment variables
(e.g.,
RCLONE_CONFIG_CLOUDFLARE_R2_ACCESS_KEY_ID
,RCLONE_CONFIG_CLOUDFLARE_R2_SECRET_ACCESS_KEY
). Set these variables securely in your deployment environment. - Secrets Management System: Integrate with a dedicated secrets management tool (like HashiCorp Vault, AWS Secrets Manager, etc.) to fetch credentials at runtime.
- Rclone Config File: Let Rclone use its standard configuration file (
- Principle of Least Privilege: Ensure the R2 API token used by Rclone has only the minimum permissions required for its tasks (e.g., read-only access if only importing files). Create specific tokens for specific applications.
- Input Validation: Sanitize any user-provided paths or parameters used in constructing Rclone
commands if applicable, although using
ProcessBuilder
with a list of arguments (as shown) significantly mitigates command injection risks compared to building a single command string. - Error Handling and Logging: Implement robust error handling that logs failures securely. Use
a proper logging framework (like Log4j2, SLF4j/Logback) instead of
System.out.println
ore.printStackTrace()
in production. Avoid logging sensitive information like full credentials or detailed internal paths in case of errors.
Here's the example showing dynamic configuration via environment variables, presented with strong caveats:
// Caution: Running 'rclone config create' programmatically can be complex
// and might expose secrets in process lists or logs if not handled carefully.
// Prefer configuring Rclone beforehand using its config file or standard env vars.
public static void configureRcloneFromEnv() throws IOException, InterruptedException {
String remoteName = "cloudflare_r2_env"; // Use a distinct name
String accessKey = System.getenv("R2_ACCESS_KEY_ID");
String secretKey = System.getenv("R2_SECRET_KEY"); // Use secure env var names
String endpoint = System.getenv("R2_ENDPOINT");
if (accessKey == null || secretKey == null || endpoint == null) {
throw new IllegalStateException("Required R2 environment variables are not set.");
}
// Note: Passing secrets directly on the command line is generally discouraged.
// Rclone has safer ways (env vars like RCLONE_CONFIG_REMOTE_ACCESS_KEY_ID).
// This example is illustrative; review Rclone docs for secure credential handling.
List<String> command = List.of(
"rclone", "config", "create", remoteName, "s3",
"provider=Cloudflare",
"access_key_id=" + accessKey,
"secret_access_key=" + secretKey, // HIGHLY CAUTIOUS with this approach
"endpoint=" + endpoint,
"acl=private"
// Consider using "config_is_local=true" if needed
);
System.out.println("Attempting to configure Rclone dynamically (use with extreme caution)...");
ProcessBuilder builder = new ProcessBuilder(command);
builder.redirectErrorStream(true);
Process process = builder.start();
// Capture output for debugging (avoid in production if secrets are involved)
try (BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println("Config Output: " + line);
}
}
boolean finished = process.waitFor(1, TimeUnit.MINUTES);
if (!finished) {
process.destroyForcibly();
throw new RuntimeException("Failed to configure Rclone dynamically: Timeout");
}
int exitCode = process.exitValue();
if (exitCode != 0) {
throw new RuntimeException("Failed to configure Rclone dynamically. Exit code: " + exitCode);
}
System.out.println("Rclone remote '" + remoteName + "' configured dynamically (verify security implications).");
}
Conclusion and additional resources
Integrating Cloudflare R2
with Java
using Rclone provides a robust and efficient solution for
file importing
tasks. The combination offers flexibility, performance, and the cost-effectiveness
of R2's zero egress fees for your application's storage needs. By leveraging ProcessBuilder
carefully and understanding Rclone's command-line options, you can build powerful cloud storage
interactions into your Java services.
For further exploration, check out these resources:
- Rclone Official Documentation
- Rclone S3 Backend Documentation (includes Cloudflare R2)
- Cloudflare R2 Documentation
- Java ProcessBuilder Documentation
If you're looking for a fully managed solution that handles the complexities of cloud imports, Transloadit offers a dedicated 🤖 Cloudflare Import Robot as part of our File Importing service. This Robot simplifies the process and supports advanced features like recursive directory imports, pagination control, file stub generation for on-demand processing, and secure authentication using Template Credentials. Transloadit also provides a convenient Java SDK to streamline integration with our platform.