Merging PDF documents in PHP using FPDI and FPDF
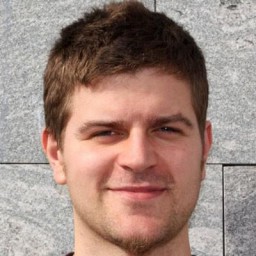
Need to merge multiple PDFs into one file in your PHP application? In this DevTip, we'll explore how to combine PDF documents programmatically using PHP, leveraging the FPDI and FPDF libraries.
Prerequisites
Before we begin, ensure you have the following:
- PHP 7.4 or higher
- Composer installed
- Basic knowledge of PHP
Installing FPDI and FPDF libraries
First, let's install the required libraries using Composer. Open your terminal and run:
composer require setasign/fpdf setasign/fpdi
This command installs both FPDF and FPDI libraries in your project.
Basic usage of FPDF
FPDF is a PHP class that allows you to generate PDF files. Here's a simple example of creating a PDF with FPDF:
<?php
require 'vendor/autoload.php';
$pdf = new FPDF();
$pdf->AddPage();
$pdf->SetFont('Arial', 'B', 16);
$pdf->Cell(40, 10, 'Hello World!');
$pdf->Output('F', 'hello_world.pdf');
This script creates a PDF file named hello_world.pdf
with the text "Hello World!".
Importing existing PDFs with FPDI
FPDI extends FPDF to allow importing pages from existing PDF documents. Here's how to import a page from an existing PDF:
<?php
require 'vendor/autoload.php';
use setasign\Fpdi\Fpdi;
$pdf = new Fpdi();
$pdf->AddPage();
$pdf->setSourceFile('existing.pdf');
$tplId = $pdf->importPage(1);
$pdf->useTemplate($tplId);
$pdf->Output('F', 'imported.pdf');
This script imports the first page of existing.pdf
and creates a new PDF named imported.pdf
.
Merging two PDFs
Now, let's combine the knowledge to merge two PDF documents:
<?php
require 'vendor/autoload.php';
use setasign\Fpdi\Fpdi;
function mergePDFs($file1, $file2, $outputFile) {
$pdf = new Fpdi();
// Import pages from the first PDF
$pageCount = $pdf->setSourceFile($file1);
for ($i = 1; $i <= $pageCount; $i++) {
$tplId = $pdf->importPage($i);
$pdf->AddPage();
$pdf->useTemplate($tplId);
}
// Import pages from the second PDF
$pageCount = $pdf->setSourceFile($file2);
for ($i = 1; $i <= $pageCount; $i++) {
$tplId = $pdf->importPage($i);
$pdf->AddPage();
$pdf->useTemplate($tplId);
}
$pdf->Output('F', $outputFile);
}
mergePDFs('document1.pdf', 'document2.pdf', 'merged.pdf');
This function takes two input PDF files, imports all pages from both, and creates a new merged PDF.
Handling multiple PDFs dynamically
To merge an arbitrary number of PDFs, we can modify our function to accept an array of file paths:
<?php
require 'vendor/autoload.php';
use setasign\Fpdi\Fpdi;
function mergePDFs(array $files, $outputFile) {
$pdf = new Fpdi();
foreach ($files as $file) {
$pageCount = $pdf->setSourceFile($file);
for ($i = 1; $i <= $pageCount; $i++) {
$tplId = $pdf->importPage($i);
$pdf->AddPage();
$pdf->useTemplate($tplId);
}
}
$pdf->Output('F', $outputFile);
}
$filesToMerge = ['document1.pdf', 'document2.pdf', 'document3.pdf'];
mergePDFs($filesToMerge, 'merged_multiple.pdf');
This version of the function can handle any number of input PDFs, making it more flexible for various use cases.
Error handling and best practices
When working with file operations, it's crucial to implement proper error handling:
<?php
require 'vendor/autoload.php';
use setasign\Fpdi\Fpdi;
use setasign\Fpdi\PdfParser\PdfParserException;
function mergePDFs(array $files, $outputFile) {
try {
$pdf = new Fpdi();
foreach ($files as $file) {
if (!file_exists($file)) {
throw new Exception("File not found: $file");
}
$pageCount = $pdf->setSourceFile($file);
for ($i = 1; $i <= $pageCount; $i++) {
$tplId = $pdf->importPage($i);
$pdf->AddPage();
$pdf->useTemplate($tplId);
}
}
$pdf->Output('F', $outputFile);
return true;
} catch (PdfParserException $e) {
error_log("PDF Parser Error: " . $e->getMessage());
return false;
} catch (Exception $e) {
error_log("Error: " . $e->getMessage());
return false;
}
}
$filesToMerge = ['document1.pdf', 'document2.pdf', 'document3.pdf'];
if (mergePDFs($filesToMerge, 'merged_multiple.pdf')) {
echo "PDFs merged successfully.";
} else {
echo "Failed to merge PDFs. Check error log for details.";
}
This version includes error handling for file existence and PDF parsing issues, providing more robust operation.
Conclusion
Merging PDF documents in PHP using the FPDI and FPDF libraries is a powerful technique for PDF manipulation. This approach allows for flexible and programmatic PDF merging, which can be especially useful in web applications that handle document processing.
While this method works well for basic PDF merging, complex document processing might require more advanced solutions. For scalable and feature-rich document processing, consider using a dedicated service like Transloadit's Document Processing service, which offers robust capabilities for handling various document formats and operations.