Optimizing image processing in Rust with parallelism and Rayon
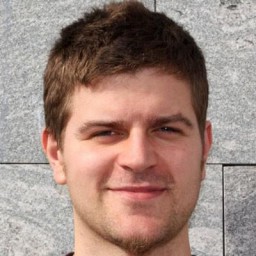
Processing images, such as converting formats, resizing dimensions, or adding watermarks, are common tasks in many applications. Rust, with its performance and safety guarantees, is an excellent choice for building efficient image processing tools. In this guide, we'll explore how to use Rust and open-source libraries to convert, resize, and watermark images.
Introduction to image processing in Rust
Rust's powerful ecosystem and performance make it ideal for handling image manipulation tasks. By leveraging open-source libraries, we can build image processing applications that are both efficient and reliable.
Setting up your Rust environment
Install Rust using rustup:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
Create a new project:
cargo new image_processor
cd image_processor
Utilizing open-source libraries for image manipulation
We'll use the image
crate, a popular open-source library in Rust for image processing tasks. Add
the following dependencies to your Cargo.toml
:
[dependencies]
image = "0.24.6"
anyhow = "1.0.75"
rayon = "1.7"
thiserror = "1.0"
log = "0.4"
env_logger = "0.10"
Converting images: step-by-step guide
To convert images between formats (e.g., from PNG to JPEG), you can use the image
crate as
follows:
use anyhow::{Context, Result};
use image::ImageFormat;
use std::path::Path;
fn convert_image(input_path: &Path, output_path: &Path, output_format: ImageFormat) -> Result<()> {
let img = image::open(input_path)
.with_context(|| format!("Failed to open image: {}", input_path.display()))?;
img.save_with_format(output_path, output_format)
.with_context(|| format!("Failed to save image: {}", output_path.display()))?;
Ok(())
}
Usage:
fn main() -> Result<()> {
let input_path = Path::new("input.png");
let output_path = Path::new("output.jpg");
convert_image(input_path, output_path, ImageFormat::Jpeg)?;
Ok(())
}
Resizing images: practical examples
Resizing images can be done using the resize
function from the image::imageops
module:
use image::imageops::FilterType;
fn resize_image(input_path: &Path, output_path: &Path, new_width: u32, new_height: u32) -> Result<()> {
const CHUNK_SIZE: u32 = 1024;
let img = image::open(input_path)?;
let (width, height) = img.dimensions();
let aspect_ratio = width as f32 / height as f32;
let (final_width, final_height) = if (new_width as f32 / new_height as f32) > aspect_ratio {
(new_height as f32 * aspect_ratio, new_height as f32)
} else {
(new_width as f32, new_width as f32 / aspect_ratio)
};
validate_dimensions(final_width as u32, final_height as u32)?;
let mut output = image::RgbaImage::new(final_width as u32, final_height as u32);
// Process image in chunks
for y in (0..height).step_by(CHUNK_SIZE as usize) {
let chunk_height = CHUNK_SIZE.min(height - y);
let view = img.view(0, y, width, chunk_height);
// Create a buffer for the chunk
let chunk = view.to_image();
// Resize the chunk
let resized_chunk = chunk.resize_exact(
final_width as u32,
(chunk_height as f32 * final_height / height as f32) as u32,
FilterType::CatmullRom
);
// Copy the resized chunk to the output image
image::imageops::replace(&mut output, &resized_chunk, 0, (y as f32 * final_height / height as f32) as i64);
}
output.save(output_path)?;
Ok(())
}
Usage:
fn main() -> Result<()> {
let input_path = Path::new("input.jpg");
let output_path = Path::new("resized.jpg");
resize_image(input_path, output_path, 800, 600)?;
Ok(())
}
Adding watermarks to images
To add a watermark to an image, you can overlay one image onto another:
use image::{DynamicImage, GenericImageView};
use log::{error, info, warn};
fn add_watermark(
base_image_path: &Path,
watermark_image_path: &Path,
output_path: &Path,
position: (u32, u32),
opacity: f32,
) -> Result<()> {
// Validate inputs
validate_opacity(opacity)?;
info!("Processing image: {}", base_image_path.display());
let mut base_img = image::open(base_image_path)
.map_err(|e| {
error!("Failed to open base image: {}", e);
e
})?
.into_rgba8();
let (width, height) = base_img.dimensions();
validate_dimensions(width, height)?;
// Load and validate watermark
let watermark = image::open(watermark_image_path)
.map_err(|e| {
error!("Failed to open watermark: {}", e);
e
})?
.into_rgba8();
// Check if watermark is larger than base image
if watermark.dimensions().0 > width || watermark.dimensions().1 > height {
warn!("Watermark is larger than base image, consider resizing");
}
// Create a mutable copy of the watermark to adjust opacity
let mut watermark = watermark.clone();
// Adjust opacity
for pixel in watermark.pixels_mut() {
let alpha = (pixel[3] as f32 * opacity).min(255.0) as u8;
pixel[3] = alpha;
}
image::imageops::overlay(&mut base_img, &watermark, position.0, position.1);
// Convert back to DynamicImage for saving
let final_image = DynamicImage::ImageRgba8(base_img);
final_image.save(output_path)
.with_context(|| format!("Failed to save image: {}", output_path.display()))?;
Ok(())
}
Usage:
fn main() -> Result<()> {
let base_image_path = Path::new("input.jpg");
let watermark_image_path = Path::new("watermark.png");
let output_path = Path::new("watermarked.jpg");
add_watermark(base_image_path, watermark_image_path, output_path, (50, 50), 0.5)?;
Ok(())
}
Integrating image processing tasks
You can combine these functions to build a comprehensive image processing pipeline:
fn process_image(input_path: &Path, output_path: &Path, watermark_path: Option<&Path>) -> Result<()> {
// Resize the image
resize_image(input_path, output_path, 800, 600)?;
// Add watermark if provided
if let Some(wm_path) = watermark_path {
add_watermark(output_path, wm_path, output_path, (20, 20), 0.3)?;
}
Ok(())
}
Example usage
fn main() -> Result<()> {
let input_path = Path::new("input.png");
let output_path = Path::new("processed.jpg");
let watermark_path = Some(Path::new("watermark.png"));
process_image(input_path, output_path, watermark_path)?;
Ok(())
}
Benefits of using Rust for image processing
Rust provides several advantages for image processing tasks:
- Performance: Rust offers performance comparable to C/C++, making it ideal for computationally intensive tasks.
- Safety: Rust's ownership model prevents common bugs such as null pointer dereferencing and data races.
- Concurrency: Rust makes it easier to write concurrent code, allowing for efficient multi-threaded processing.
- Ecosystem: The availability of crates like
image
simplifies image manipulation tasks.
Parallel processing with Rayon
For batch processing multiple images, we can use Rayon to parallelize the work:
use rayon::prelude::*;
use std::sync::atomic::{AtomicUsize, Ordering};
struct ProcessingStats {
processed: AtomicUsize,
failed: AtomicUsize,
}
fn process_directory(
input_dir: &Path,
output_dir: &Path,
watermark_path: Option<&Path>
) -> Result<()> {
let stats = ProcessingStats {
processed: AtomicUsize::new(0),
failed: AtomicUsize::new(0),
};
// Create output directory if it doesn't exist
std::fs::create_dir_all(output_dir)?;
// Collect all image files
let entries: Vec<_> = std::fs::read_dir(input_dir)?
.filter_map(Result::ok)
.filter(|entry| {
entry.path().extension()
.map(|ext| ext.eq_ignore_ascii_case("jpg") ||
ext.eq_ignore_ascii_case("png"))
.unwrap_or(false)
})
.collect();
// Process images in parallel
entries.par_iter()
.try_for_each(|entry| {
let input_path = entry.path();
let output_path = output_dir.join(entry.file_name());
match process_image(&input_path, &output_path, watermark_path) {
Ok(_) => {
stats.processed.fetch_add(1, Ordering::Relaxed);
info!("Successfully processed: {}", input_path.display());
Ok(())
}
Err(e) => {
stats.failed.fetch_add(1, Ordering::Relaxed);
error!("Failed to process {}: {}", input_path.display(), e);
Err(e)
}
}
})?;
info!(
"Processing complete: {} succeeded, {} failed",
stats.processed.load(Ordering::Relaxed),
stats.failed.load(Ordering::Relaxed)
);
Ok(())
}
Usage example for batch processing:
fn main() -> Result<()> {
// Initialize logging
env_logger::init();
let input_dir = Path::new("input_images");
let output_dir = Path::new("processed_images");
let watermark_path = Some(Path::new("watermark.png"));
process_directory(input_dir, output_dir, watermark_path)?;
Ok(())
}
This parallel implementation will:
- Process multiple images simultaneously using all available CPU cores
- Track success and failure statistics
- Provide logging for monitoring progress
- Handle errors gracefully without stopping the entire batch
Conclusion
The combination of Rust's safety guarantees and Rayon's parallel processing capabilities creates a robust foundation for high-performance image processing. The examples shown here demonstrate memory-efficient batch operations that can fully utilize modern multi-core systems.
For scalable and cloud-based image processing solutions, consider using Transloadit's Image Manipulation service.