Recognize text in images (OCR) in Rust
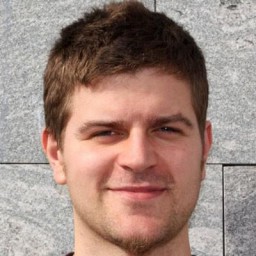
Optical Character Recognition (OCR) is a powerful technology that enables computers to extract text from images. In this DevTip, we will explore how to implement OCR in Rust using the Tesseract library, along with best practices for achieving accurate results.
Introduction
Rust's performance and safety guarantees make it an excellent choice for implementing OCR solutions. Whether you are building a document processing system or adding text extraction capabilities to your application, Rust provides the tools and ecosystem to handle these tasks efficiently.
Prerequisites
- Rust installed on your system
- Tesseract OCR installed (version 4.0 or later)
- Basic knowledge of Rust and Cargo
Setting up the project
First, create a new Rust project and add the required dependencies to your Cargo.toml
:
[dependencies]
tesseract = "0.7"
image = "0.24"
anyhow = "1.0"
Ensure that you are using the latest versions of the crates for compatibility and performance.
Installing tesseract
Before we can use the Rust bindings, we need to install Tesseract on our system.
On Ubuntu/Debian
sudo apt-get install tesseract-ocr libtesseract-dev
On macOS
brew install tesseract
Basic OCR implementation
Let us start with a basic example that demonstrates how to extract text from an image:
use anyhow::Result;
use tesseract::Tesseract;
fn main() -> Result<()> {
let mut ocr = Tesseract::new(None, Some("eng"))?;
// Set the image to process
ocr.set_image("input.png")?;
// Get the text output
let text = ocr.get_text()?;
println!("Extracted text:\n{}", text);
Ok(())
}
This code initializes a new Tesseract
instance for English language recognition, sets the image
input.png
, and prints the extracted text.
Handling different image formats
Sometimes you will need to preprocess images for better OCR results. Preprocessing can enhance image quality and improve recognition accuracy. Here is how to handle image conversion and enhancement:
use anyhow::Result;
use image::DynamicImage;
use tesseract::Tesseract;
fn prepare_image_for_ocr(image_path: &str) -> Result<String> {
// Load the image and convert to grayscale
let img = image::open(image_path)?.grayscale();
// Adjust contrast
let img = img.adjust_contrast(1.5);
// Save preprocessed image to a temporary file
let temp_path = "temp_processed.png";
img.save(temp_path)?;
// Perform OCR on the processed image
let mut ocr = Tesseract::new(None, Some("eng"))?;
ocr.set_image(temp_path)?;
let text = ocr.get_text()?;
std::fs::remove_file(temp_path)?; // Clean up the temporary file
Ok(text)
}
By converting the image to grayscale and adjusting the contrast, we can make text more distinguishable for OCR.
Advanced OCR configuration
Tesseract provides various configuration options to improve recognition accuracy. For example, you can specify a character whitelist or adjust the page segmentation mode:
use anyhow::Result;
use tesseract::Tesseract;
fn configure_ocr() -> Result<String> {
let mut ocr = Tesseract::new(None, Some("eng"))?;
// Configure OCR parameters
ocr.set_variable("tessedit_char_whitelist", "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz")?;
// Set the page segmentation mode (e.g., 1 = Automatic page segmentation with OSD)
ocr.set_variable("tessedit_pageseg_mode", "1")?;
ocr.set_image("input.png")?;
let text = ocr.get_text()?;
Ok(text)
}
tessedit_char_whitelist
allows you to specify which characters Tesseract should recognize, potentially reducing errors by ignoring irrelevant characters.tessedit_pageseg_mode
changes how Tesseract segments the image into text blocks, which can improve recognition on different layouts.
Best practices for OCR in Rust
-
Image Preprocessing
- Convert images to grayscale: Simplifies the image and reduces noise.
- Adjust contrast and brightness: Enhances text visibility.
- Remove noise: Apply filters to clean up the image.
- Ensure sufficient resolution: A resolution of 300 DPI is recommended for clear text.
-
Performance Optimization
- Use parallel processing for multiple images: Utilize Rust's concurrency features to process images in parallel.
- Implement caching: Cache results for frequently processed documents to save time.
- Consider batch processing: For large volumes of images, batch processing can be more efficient.
-
Error Handling
Proper error handling ensures your application can gracefully handle issues during OCR processing:
use anyhow::{Context, Result};
use tesseract::Tesseract;
fn robust_ocr(image_path: &str) -> Result<String> {
let mut ocr = Tesseract::new(None, Some("eng"))
.with_context(|| "Failed to initialize Tesseract")?;
ocr.set_image(image_path)
.with_context(|| format!("Failed to set image '{}'", image_path))?;
let text = ocr.get_text()
.with_context(|| "Failed to extract text")?;
Ok(text)
}
Using the anyhow
crate's Context
trait provides more informative error messages.
Handling multiple languages
Tesseract supports multiple languages. Here is how to work with them:
use anyhow::Result;
use tesseract::Tesseract;
fn multilingual_ocr(image_path: &str) -> Result<String> {
// Specify multiple languages (e.g., English, French, German)
let mut ocr = Tesseract::new(None, Some("eng+fra+deu"))?;
ocr.set_image(image_path)?;
let text = ocr.get_text()?;
Ok(text)
}
By specifying the languages, Tesseract will attempt to recognize text in all listed languages.
Conclusion
Implementing OCR in Rust using Tesseract provides a robust solution for text recognition in images. The combination of Rust's safety and performance with Tesseract's powerful OCR capabilities enables you to build reliable text extraction systems.
Transloadit offers powerful document processing capabilities through our Document Processing Service, which can complement your OCR implementations with additional features like format conversion and metadata extraction.