Secure ajax file uploads: best practices and techniques
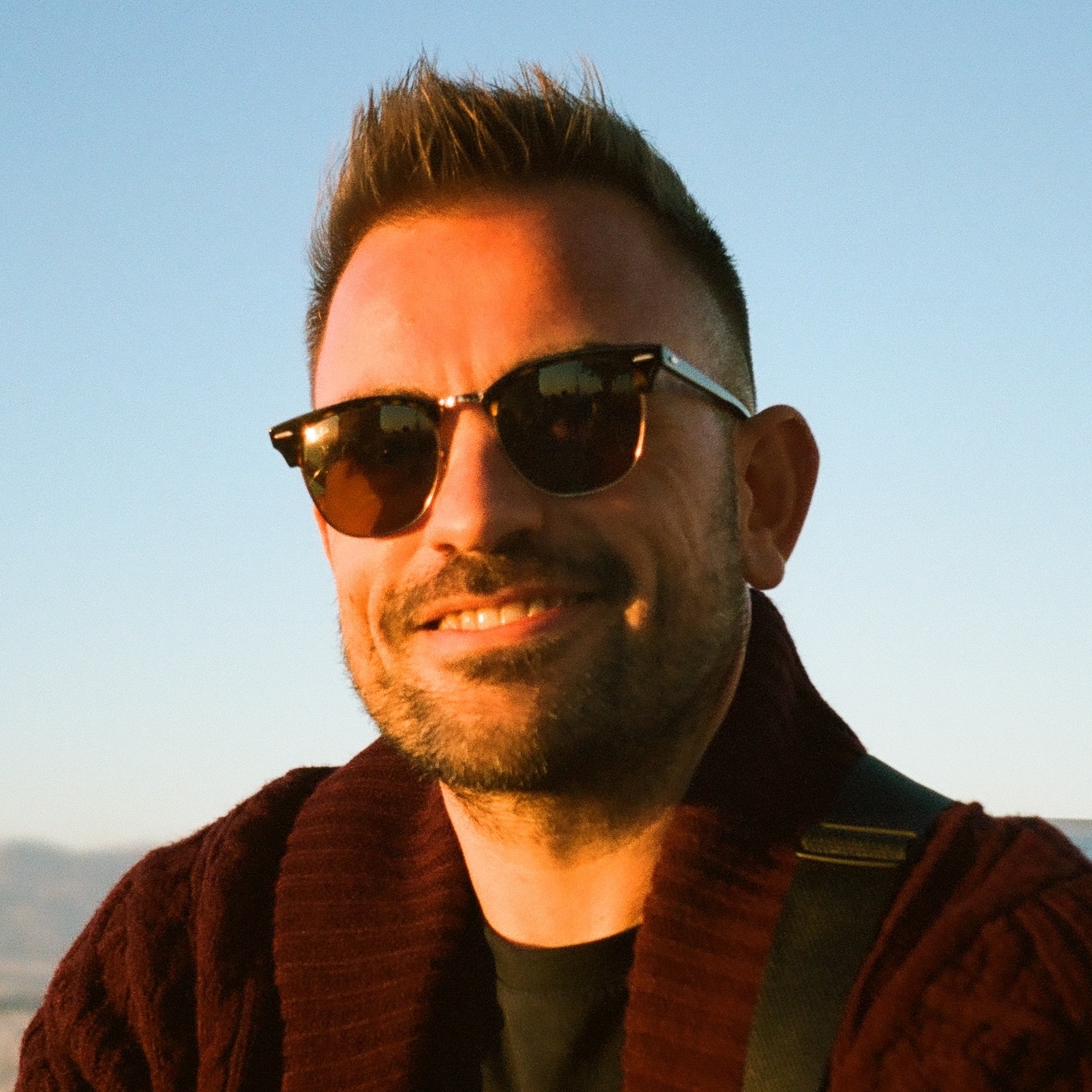
Securely handling file uploads is critical in modern web development. As AJAX file uploads become increasingly prevalent, protecting your application from potential vulnerabilities is essential. This article explores best practices and techniques to enhance the security of AJAX file uploads in your web applications.
Understanding ajax file uploads
AJAX (Asynchronous JavaScript and XML) allows web applications to send and receive data from the server asynchronously without refreshing the page, enhancing user experience with seamless interactions. When implementing file uploads with AJAX, it's important to address both client-side and server-side security measures.
Techniques for ajax file upload
Uploading files via AJAX can be achieved through various methods. Two common techniques are:
-
Using the FormData API with Fetch or XMLHttpRequest: Modern browsers support the
FormData
interface, allowing developers to construct a set of key/value pairs representing form fields and their values. Combined with thefetch
API orXMLHttpRequest
, you can submit forms asynchronously, including file uploads. -
Using iframes for Legacy Support: In older browsers that do not support the
FormData
API, developers used hidden iframes to emulate asynchronous file uploads. While this method is largely obsolete due to modern browser support, it's important to be aware of it when maintaining legacy systems.
In this article, we'll focus on using the FormData
API with modern JavaScript techniques.
Implementing ajax file upload with vanilla JavaScript
Using formdata and the fetch API
Modern browsers support the FormData
interface and the fetch
API for AJAX requests. This
combination allows for efficient and secure file uploads.
const fileInput = document.querySelector('#fileInput')
const formData = new FormData()
formData.append('file', fileInput.files[0])
fetch('/upload', {
method: 'POST',
body: formData,
})
.then((response) => response.json())
.then((data) => console.log(data))
.catch((error) => console.error('Error:', error))
Handling file upload with xmlhttprequest
Alternatively, you can use the XMLHttpRequest
object, which provides more control over the AJAX
request and allows monitoring upload progress.
const fileInput = document.querySelector('#fileInput')
const formData = new FormData()
formData.append('file', fileInput.files[0])
const xhr = new XMLHttpRequest()
xhr.open('POST', '/upload', true)
xhr.upload.addEventListener('progress', (event) => {
if (event.lengthComputable) {
const percentComplete = (event.loaded / event.total) * 100
console.log(`Upload progress: ${percentComplete}%`)
}
})
xhr.onload = () => {
if (xhr.status === 200) {
console.log('File uploaded successfully')
} else {
console.error('Upload failed')
}
}
xhr.onerror = () => {
console.error('Error uploading file')
}
xhr.send(formData)
Uploading files with jQuery ajax
While modern JavaScript allows for straightforward AJAX file uploads, many projects still utilize jQuery for its simplicity and widespread adoption. Here's how to perform an AJAX file upload using jQuery.
<input type="file" id="fileInput" /> <button id="uploadButton">Upload</button>
$('#uploadButton').click(function () {
const fileData = new FormData()
const file = $('#fileInput')[0].files[0]
fileData.append('file', file)
$.ajax({
url: '/upload',
type: 'POST',
data: fileData,
processData: false,
contentType: false,
success: function (data) {
console.log('File uploaded successfully')
},
error: function (jqXHR, textStatus, errorThrown) {
console.error('Upload failed:', textStatus, errorThrown)
},
})
})
Validating file types and sizes on the client side
Client-side validation enhances user experience by providing immediate feedback.
const allowedTypes = ['image/png', 'image/jpeg', 'application/pdf']
const maxSize = 5 * 1024 * 1024 // 5 MB
fileInput.addEventListener('change', (event) => {
const file = event.target.files[0]
if (!allowedTypes.includes(file.type)) {
alert('Invalid file type.')
return
}
if (file.size > maxSize) {
alert('File is too large.')
return
}
// Proceed with upload
})
Server-side validation and sanitization
Never rely solely on client-side validation. Implement server-side checks to ensure security.
Example in Node.js with Express
const express = require('express')
const multer = require('multer')
const path = require('path')
const app = express()
const storage = multer.diskStorage({
destination: (req, file, cb) => {
cb(null, 'uploads/')
},
filename: (req, file, cb) => {
cb(null, Date.now() + path.extname(file.originalname))
},
})
const fileFilter = (req, file, cb) => {
const allowedTypes = ['image/png', 'image/jpeg', 'application/pdf']
if (allowedTypes.includes(file.mimetype)) {
cb(null, true)
} else {
cb(new Error('Invalid file type'), false)
}
}
const upload = multer({
storage: storage,
limits: { fileSize: 5 * 1024 * 1024 }, // 5 MB
fileFilter: fileFilter,
})
app.post('/upload', upload.single('file'), (req, res) => {
res.json({ message: 'File uploaded successfully' })
})
app.use((err, req, res, next) => {
res.status(400).json({ error: err.message })
})
app.listen(3000, () => console.log('Server started on port 3000'))
Handling ajax file upload errors
Proper error handling improves user experience and application reliability.
fetch('/upload', {
method: 'POST',
body: formData,
})
.then((response) => {
if (!response.ok) {
throw new Error('Network response was not ok')
}
return response.json()
})
.then((data) => console.log(data))
.catch((error) => {
console.error('Error:', error)
alert('File upload failed. Please try again.')
})
On the server side, ensure that errors are properly sent with appropriate HTTP status codes and messages.
app.use((err, req, res, next) => {
res.status(400).json({ error: err.message })
})
Best practices to enhance security
Implementing secure AJAX file uploads requires careful attention to both client-side and server-side practices. Here are some best practices to follow:
- Limit File Types: Accept only necessary file types.
- Set File Size Limits: Prevent large files from overloading the server.
- Use a Secure Upload Directory: Store uploads outside the web root or in a directory with restricted access.
- Rename Files: Avoid using user-supplied filenames to prevent overwriting and path traversal attacks.
- Scan Uploaded Files: Use antivirus or malware scanning tools on the server.
- Implement HTTPS: Encrypt data in transit to protect against man-in-the-middle attacks.
Conclusion
By following these best practices and thoroughly validating uploads, you can protect your application from common vulnerabilities and provide a safe experience for your users.
For advanced file upload solutions with robust security features, consider using Uppy by Transloadit—a sleek, open-source file uploader that simplifies file uploading and enhances security.