Using signed URLs to secure your CDN content
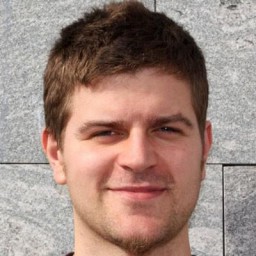
Content Delivery Networks (CDNs) are essential for efficient content delivery across the globe, but they can expose your content to unauthorized access if not properly secured. This DevTip explores how to enhance CDN security by implementing signed URLs, ensuring that only authorized users can access your content.
Understanding CDN security and signed URLs
CDNs improve content delivery speed and user experience by caching content at edge locations. However, without proper access controls, your content could be vulnerable to unauthorized users, leading to potential misuse or piracy. Implementing signed URLs enhances CDN security by adding a layer of token authentication, granting temporary and secure access to your protected resources.
Benefits of using signed URLs:
- Restrict access to premium or sensitive content
- Prevent unauthorized hotlinking and reduce bandwidth theft
- Comply with licensing agreements and security policies
- Control content access duration and revoke access when needed
- Enhance content security without sacrificing performance
Implementing signed URLs with your CDN
Most major CDN providers support signed URLs or token-based authentication mechanisms. Here's a general process for implementing signed URLs:
- Choose a CDN provider supporting signed URL functionality: Options include AWS CloudFront, Google Cloud CDN, and others that offer robust content security features.
- Configure your origin server: Ensure your origin server is set up to serve content through the CDN, with appropriate permissions and policies.
- Set up SSL/TLS encryption: Secure data transmission by enabling HTTPS on your CDN and origin server to protect against eavesdropping.
- Enable and configure signed URL functionality: In your CDN's settings, enable signed URLs or token authentication. Configure necessary parameters, such as expiration times and access policies.
- Generate and manage signing keys: Create key pairs or secrets used to sign URLs. Store them securely and implement key rotation policies to maintain security.
Generating signed urls: AWS cloudfront example
Let's walk through generating a signed URL using AWS CloudFront and Node.js with the AWS SDK for JavaScript v3:
- Install the AWS SDK packages:
npm install @aws-sdk/client-cloudfront @aws-sdk/cloudfront-signer
- Generate a signed URL:
import fs from 'fs'
import { getSignedUrl } from '@aws-sdk/cloudfront-signer'
function generateSignedUrl(resourceUrl, expiresIn = 3600) {
const privateKey = fs.readFileSync(process.env.CLOUDFRONT_PRIVATE_KEY_PATH, 'utf8')
const signedUrl = getSignedUrl({
url: resourceUrl,
keyPairId: process.env.CLOUDFRONT_KEY_PAIR_ID,
privateKey: privateKey,
dateLessThan: new Date(Date.now() + expiresIn * 1000).toISOString(),
})
return signedUrl
}
const resourceUrl = 'https://your-distribution.cloudfront.net/path/to/your/content'
const signedUrl = generateSignedUrl(resourceUrl)
console.log(`Signed URL: ${signedUrl}`)
Ensure you securely store and access your CloudFront key pair ID and private key. Use environment variables or secure configuration management to prevent exposing sensitive information.
Testing signed URL access
To verify your signed URL implementation:
- Generate a signed URL for a protected resource using your application.
- Access the signed URL in a browser within the expiry time; the content should load.
- Attempt to access the original, unsigned URL; access should be denied.
- Wait for the signed URL to expire, then try accessing it again; access should be denied.
Best practices for CDN security
Implement these practices to enhance your CDN security:
- Use HTTPS exclusively to encrypt data in transit.
- Set short expiration times for signed URLs to minimize unauthorized access risks.
- Regularly rotate signing keys to maintain security.
- Implement the principle of least privilege for signing credentials.
- Monitor access logs to detect and respond to suspicious activity promptly.
- Use geo-restriction features if your content has regional limitations.
- Implement token authentication for more granular access control.
- Keep your CDN and origin servers updated to protect against known vulnerabilities.
Conclusion
Implementing signed URLs significantly enhances CDN security, ensuring that only authorized users can access your content. By following the steps and best practices outlined in this guide, you can protect your valuable assets while maintaining the performance benefits of using a Content Delivery Network.
While securing your CDN content, consider how Transloadit can enhance your file processing workflow.