Using tus-client in Java for efficient file transfers
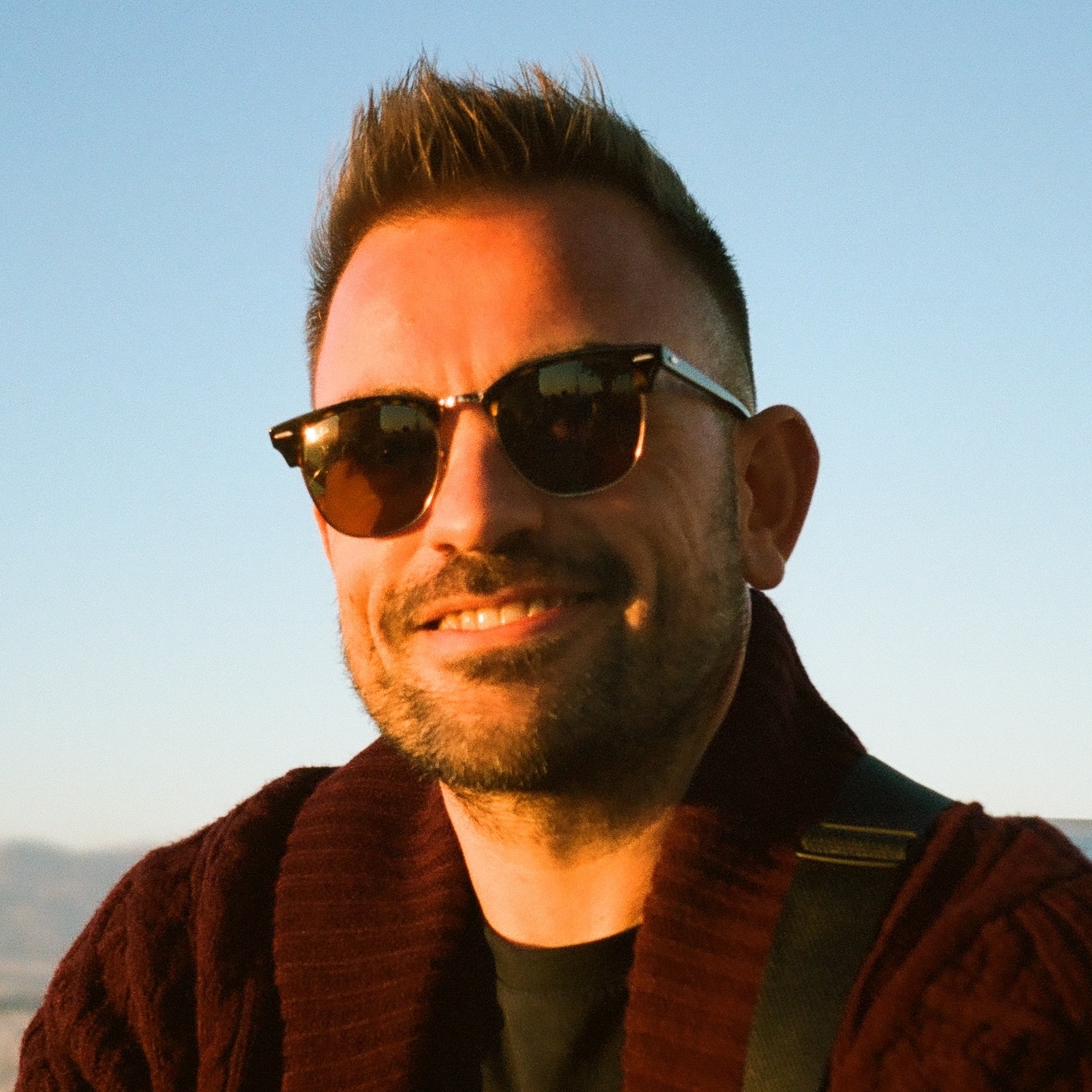
The tus protocol enables resumable file uploads over HTTP, allowing uploads to be paused and resumed without re-uploading previous data. This is particularly useful for large files and unstable networks. Let's explore how to use the tus-java-client library to implement resumable uploads in Java.
Setting up tus-java-client
Add the dependency to your project:
<dependency>
<groupId>io.tus.java.client</groupId>
<artifactId>tus-java-client</artifactId>
<version>0.5.0</version>
</dependency>
Basic usage
Here's a complete example showing how to use tus-java-client with best practices:
import io.tus.java.client.*;
import java.io.File;
import java.io.IOException;
import java.net.URL;
public class TusUploaderExample {
public static void main(String[] args) {
try {
// Create and configure client
TusClient client = new TusClient();
client.setUploadCreationURL(new URL("https://tusd.tusdemo.net/files"));
client.enableResuming(new TusURLMemoryStore());
// Prepare file for upload
File file = new File("path/to/your/file.ext");
final TusUpload upload = new TusUpload(file);
// Use TusExecutor for automatic retries
TusExecutor executor = new TusExecutor() {
@Override
protected void makeAttempt() throws ProtocolException, IOException {
// Resume or create upload
TusUploader uploader = client.resumeOrCreateUpload(upload);
// Set chunk size (1KB in this example)
uploader.setChunkSize(1024);
// Upload with progress tracking
do {
long totalBytes = upload.getSize();
long bytesUploaded = uploader.getOffset();
double progress = (double) bytesUploaded / totalBytes * 100;
System.out.printf("Upload at %06.2f%%.\n", progress);
} while(uploader.uploadChunk() > -1);
uploader.finish();
System.out.println("Upload URL: " + uploader.getUploadURL());
}
};
executor.makeAttempts();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Advanced features
-
Custom Server Support: For servers without Creation extension support:
TusUploader uploader = client.beginOrResumeUploadFromURL(upload, new URL("https://tus.server.net/files/my_file"));
-
Proxy Support: Add a proxy to the client:
client.setProxy(new Proxy(Proxy.Type.HTTP, new InetSocketAddress("proxy.example.com", 8080)));
-
Custom SSL: Extend
TusClient
to use your ownSSLSocketFactory
:@Override public void prepareConnection(@NotNull HttpURLConnection connection) { super.prepareConnection(connection); if(connection instanceof HttpsURLConnection) { ((HttpsURLConnection) connection).setSSLSocketFactory(mySSLSocketFactory); } }
Platform compatibility
The library works on both standard Java and Android platforms. For Android-specific features, check out tus-android-client.
For more details, visit the tus-java-client documentation and GitHub repository.