Smart CDN enhanced with AI-powered face detection
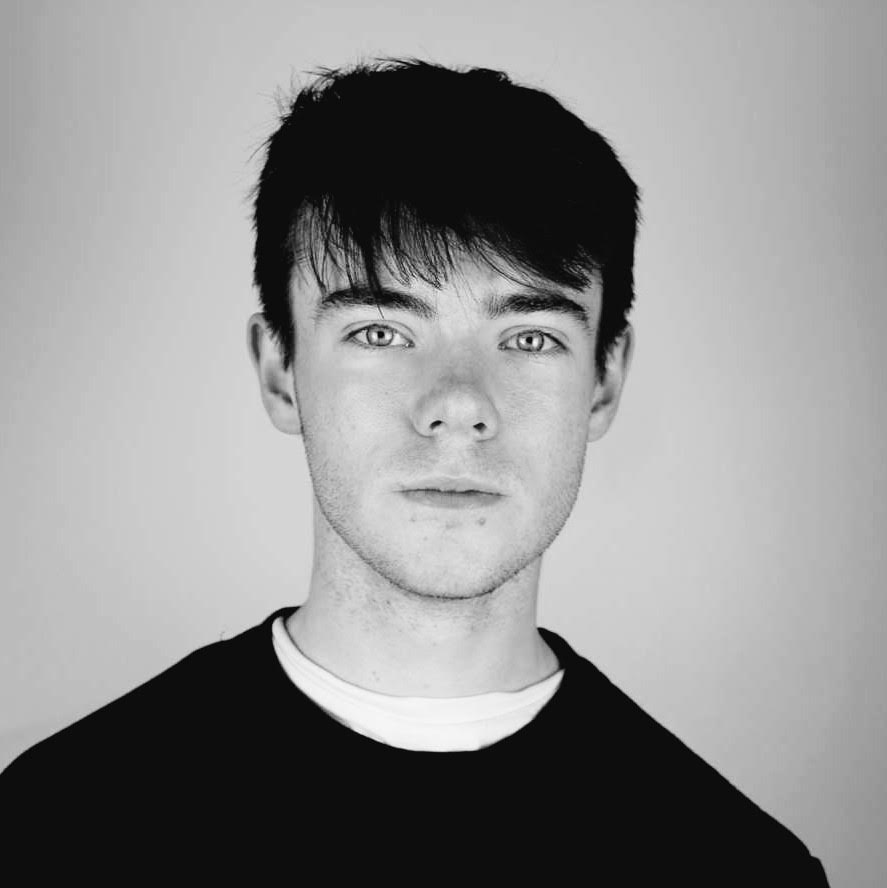
Our Smart CDN is one of the most exciting features we have released in the past few years. It helps our users receive the fastest request times we can offer, and despite it being in beta, it's making great strides every day. Today, we are excited to announce that we have updated the list of compatible Robots to now include our AI-powered /image/facedetect Robot!
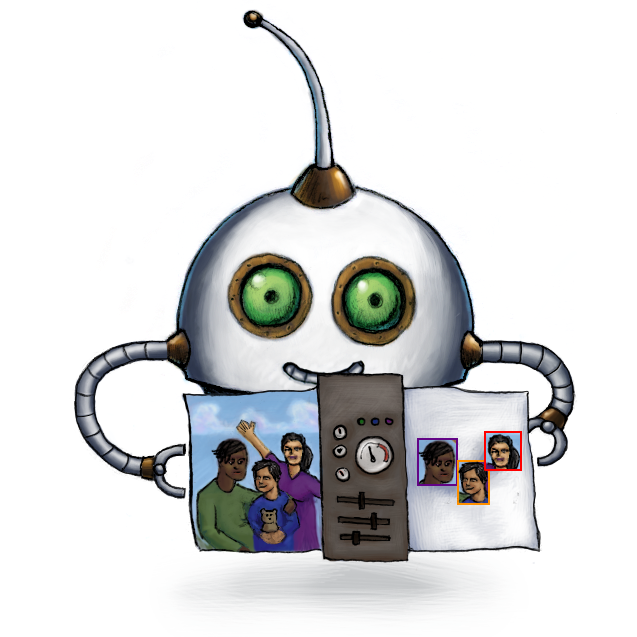
Recently, we swapped our previously used API to AWS's AI service, improving our face detection Robot, and helping to speed up transcoding when importing files from an S3 bucket. This means our /image/facedetect Robot is now right at home with our Smart CDN.
Stay tuned for a quick overview of how you can make use of this new update.
Setup
Before we get started with an example, we need to have some form of cloud storage in place that contains an image of some faces and a Template. Since our API runs on AWS, we recommend storing your files in an S3 bucket to allow for the lowest latency. If you have a different preference, you can instead opt for one of our other importing Robots.
Let's get started with creating our Template. Please be sure to take a note of your Template ID, as we need to use that later in this demonstration.
{
"steps": {
"imported": {
"robot": "/s3/import",
"result": true,
"credentials": "YOUR_S3_CREDENTIALS",
"path": "upload/${fields.input}"
},
"facedetect": {
"robot": "/image/facedetect",
"use": "imported",
"crop": true,
"faces": "${fields.type}",
"format": "preserve",
"crop_padding": "10%",
"result": true
},
"served": {
"use": "facedetect",
"robot": "/file/serve"
}
}
}
As mentioned above, we make use of S3 in our Template for importing our file. The
critical thing to look at in the first Step, "imported", is the path
parameter. Its
value specifies that we want to target a specific folder. An Assembly Variable is then
set up to determine which file we wish to call in our URL transformation.
The imported image is now passed to the facedetect
Step, where our AI-powered
/image/facedetect Robot handles the face
detection. Again, this Step uses Assembly Variables to interface with the
faces
parameter when the URL we created is called. If you would like a more in-depth explanation
about this parameter, you can find it in our previous
/image/facedetect blog.
Closing off our Template, we use the /file/serve Robot to deliver our Assembly result.
Signature Authentication
I'd also like to take a quick moment here to show just how easy it is to add Signature Authentication to our URL transformation method.
It isn't necessary to set up Signature Authentication, but we do recommend it as a best practice. It automatically protects you against DDoS attacks, makes URLs expire after generating them, and helps avoid abuse. On top of that, it probably only takes a few extra minutes to implement into your back-end.
If you'd like to see how to use our Smart CDN without Signature Authentication, you can do so on the content delivery page on our website.
Code
We've written the Signature Authentication in Node.js for this example. If you prefer to use another language in your back-end, you can see some examples here.
const crypto = require('crypto')
const secret = 'YOUR-AUTH-SECRET'
const expires = +new Date() + 60 * 60 * 1000
const signee = `YOUR-TEMPLATE-NAME/faces.jpg?type=max-confidence&expires=${expires}`
const signature = crypto
.createHmac('sha1', secret)
.update(Buffer.from(signee, 'utf-8'))
.digest('hex')
const url = `https://YOUR-APP-NAME.tlcdn.com/${signee}&signature=${signature}`
console.log(url)
The program above uses the crypto module to generate an encrypted string using your Transloadit Auth Secret and the current date that will be passed into your generated URL.
Next to the signee
variable, you will have to replace YOUR-TEMPLATE-NAME
with the name of your
own Template. Directly after that, you can specify the name of your image. In my case,
the example file is called faces.jpg
. Next, we use a wildcard with the type
field attribute we
created earlier in our Template to specify we wish to use the max-confidence
parameter
value.
Moving on, you need to alter the url
variable to contain your Transloadit App name. With that all
in place, running your Node.js app will generate a result URL!
Result
With our URL generated, all we need to do is visit the new URL for our encoding to take place.
Visiting this URL in a browser will automatically cause the encoded result to pop up once the
process is complete. If you like, you could just as easily save the Assembly result
directly to your machine using the command below. Make sure to replace url
with the value that was
outputted to your console.
curl -fsSLo /your/choosen/path/file_name.jpg ${url}
That concludes a quick look at the recent update to our Smart CDN. We're excited to see the creative ways in which you'll be able to incorporate this new feature into your projects, and we would love to hear about it on Twitter!