Accelerating file hashing in Rust with parallel processing
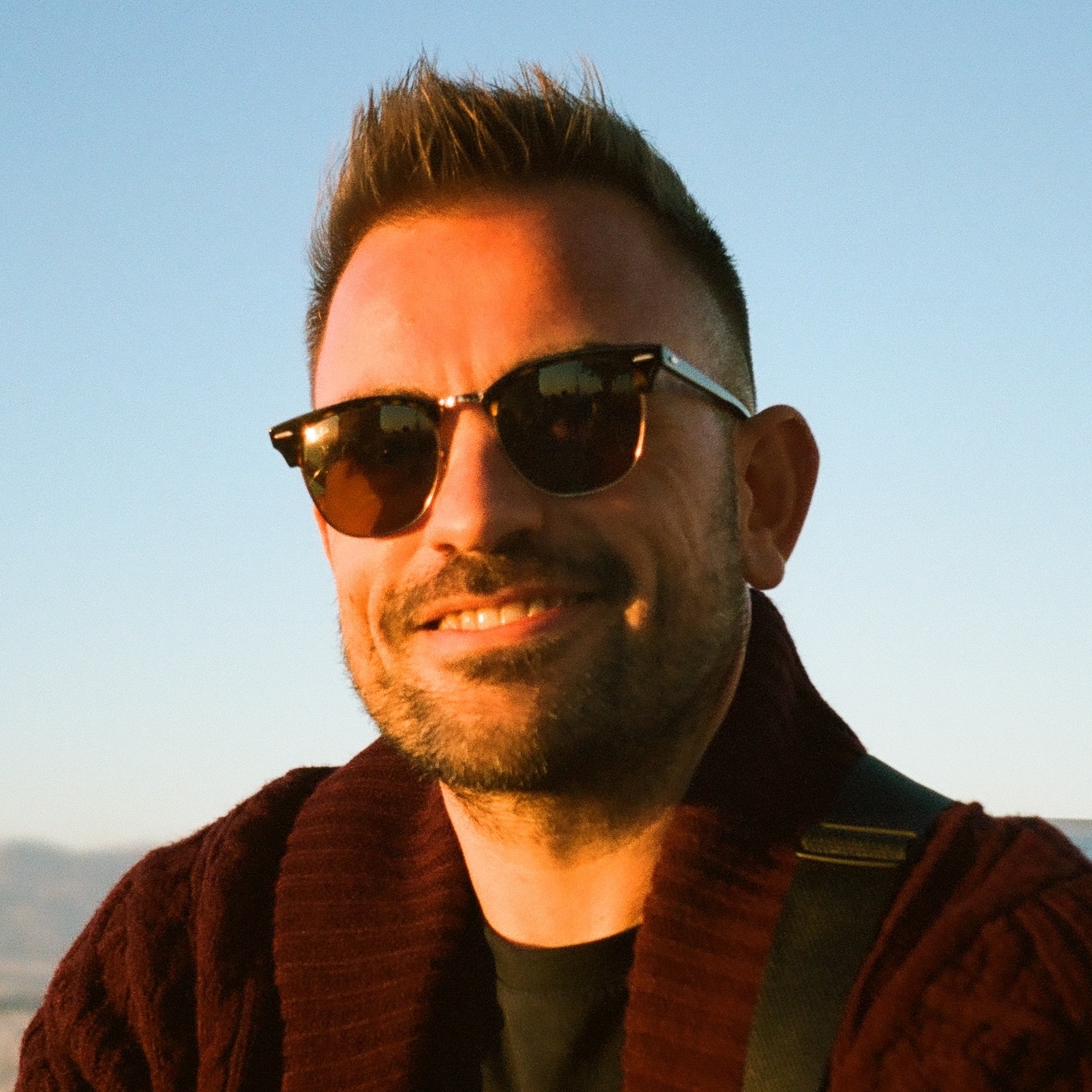
Hashing files is a fundamental task in software development, crucial for data integrity, security,
and efficient data management. In Rust, you can leverage open-source libraries like ring
and
RustCrypto
to implement robust and efficient file hashing. In this DevTip, we explore how to hash
files in Rust using these libraries, compare different hashing algorithms such as SHA-256 and
BLAKE2, and provide practical code examples to get you started.
Introduction to file hashing in Rust
File hashing generates a fixed-size string (hash) from file data that is unique to different content. Hashing verifies file integrity, detects duplicates, supports cryptographic operations, and more. Rust, with its performance and safety guarantees, is an excellent choice for implementing file hashing in your applications.
System requirements
- Rust 1.41 or higher
- A C compiler (gcc, clang, or MSVC on Windows)
- pkg-config (on Unix-like systems)
Setting up your Rust environment
First, ensure you have the latest stable version of Rust installed. You can download it from the official website or update your existing installation with:
rustup update stable
Create a new Rust project:
cargo new file-hashing
cd file-hashing
Choosing the right hashing algorithm
Selecting the appropriate hashing algorithm depends on your application's requirements:
- SHA-256: A cryptographic hash function offering high security and widely used in various applications.
- BLAKE2: A modern, faster alternative to SHA algorithms, providing comparable security with improved performance.
Note: Although MD5 appears in some libraries, it is cryptographically broken and prone to collisions. It should not be used.
Implementing file hashing with ring
The ring
crate provides safe and fast cryptographic operations,
including hashing.
Add ring
to your Cargo.toml
:
[dependencies]
ring = "0.17.8"
Hashing a file using ring
Below is an example of hashing a file using SHA-256 with ring
:
use ring::digest::{Context, SHA256};
use std::fs::File;
use std::io::{BufReader, Read};
fn sha256_digest<R: Read>(mut reader: R) -> Result<String, std::io::Error> {
let mut context = Context::new(&SHA256);
let mut buffer = [0u8; 8192];
loop {
let count = reader.read(&mut buffer)?;
if count == 0 {
break;
}
context.update(&buffer[..count]);
}
Ok(format!("{:x}", context.finish()))
}
Explanation
- Context and Digest:
ring::digest::Context
manages incremental hash computation, and the final hash is produced viacontext.finish()
. - Reading the File: The file is read in chunks to efficiently handle large files.
- Error Handling: The function returns a
Result
, allowing you to handle I/O errors, such as file access issues.
Additional features of ring
Beyond hashing, ring
offers various cryptographic functions including encryption, digital
signatures, and key agreement protocols. It is designed to be secure and performant, making it
suitable for applications with high security requirements.
Exploring advanced hashing with RustCrypto
For a wider range of algorithms and added flexibility, the RustCrypto
project provides several
hashing crates.
Add the desired hash function crate to your Cargo.toml
. For example, to use BLAKE2:
[dependencies]
blake2 = "0.10.6"
Implementing Blake2 hashing
This example demonstrates how to hash a file using BLAKE2 with RustCrypto
:
use blake2::{Blake2b512, Digest};
use std::fs::File;
use std::io::{BufReader, Read};
fn blake2b_digest<R: Read>(mut reader: R) -> Result<String, std::io::Error> {
let mut hasher = Blake2b512::new();
let mut buffer = [0u8; 8192];
loop {
let count = reader.read(&mut buffer)?;
if count == 0 {
break;
}
hasher.update(&buffer[..count]);
}
let result = hasher.finalize();
Ok(format!("{:x}", result))
}
Explanation
- Blake2b512: Implements the BLAKE2b hash function with a 512-bit output.
- Reading and Hashing: Similar to the
ring
example, the file is processed in chunks to efficiently compute the hash.
Comparative analysis of hashing methods
When choosing a hashing algorithm, consider the following factors:
- Security: SHA-256 and BLAKE2 provide robust security for cryptographic purposes.
- Performance: BLAKE2 often outperforms SHA-256, offering faster hashing with similar security.
- Compatibility: For interoperability, choose an algorithm that is widely supported across platforms.
Best practices for file hashing in Rust projects
- Use Buffered Reading: Employ a
BufReader
to optimize file I/O performance. - Handle Errors Gracefully: Utilize Rust's error handling (using the
Result
type) to manage I/O and other errors. - Avoid Blocking I/O: For applications processing multiple files, consider asynchronous I/O or parallel processing to improve throughput.
Accelerating file hashing with parallel processing
Hashing files sequentially can be inefficient when dealing with multiple files. With the rayon
crate, you can process files concurrently.
Add rayon
to your Cargo.toml
:
[dependencies]
rayon = "1.10.0"
sha2 = "0.10"
Parallel file hashing example
use rayon::prelude::*;
use std::fs::File;
use std::path::PathBuf;
use std::io::{self, BufReader};
fn main() -> Result<(), io::Error> {
let files: Vec<PathBuf> = std::env::args_os()
.skip(1)
.map(PathBuf::from)
.collect();
if files.is_empty() {
eprintln!("Usage: {} <file1> <file2> ...", env!("CARGO_PKG_NAME"));
return Ok(());
}
files.par_iter()
.try_for_each(|file| -> Result<(), io::Error> {
let input = File::open(file)?;
let reader = BufReader::new(input);
let digest = sha256_digest(reader)?;
println!("{} {}", digest, file.display());
Ok(())
})
}
Performance considerations
When using Rayon for parallel processing, be aware that parallelization overhead may only be justified for processing larger files (typically over 1MB) or multiple files. For single, small files, sequential processing may actually perform better.
How file hashing contributes to data integrity and security
- Data Integrity: Hashes verify that files have not been altered during transmission or storage.
- Security: Cryptographic hashes play a key role in authentication, password storage, and digital signatures.
- Deduplication: Hashing enables the identification of duplicate files, optimizing storage use.
Conclusion
File hashing in Rust is both straightforward and efficient with the help of open-source libraries
such as ring
and RustCrypto
. By choosing the right hashing algorithm and leveraging parallel
processing via Rayon, you can build robust applications for ensuring data integrity, security, and
efficient data management. For additional file processing solutions, consider exploring
Transloadit's Media Cataloging service.