Creating a file upload form in HTML: a developer's tutorial
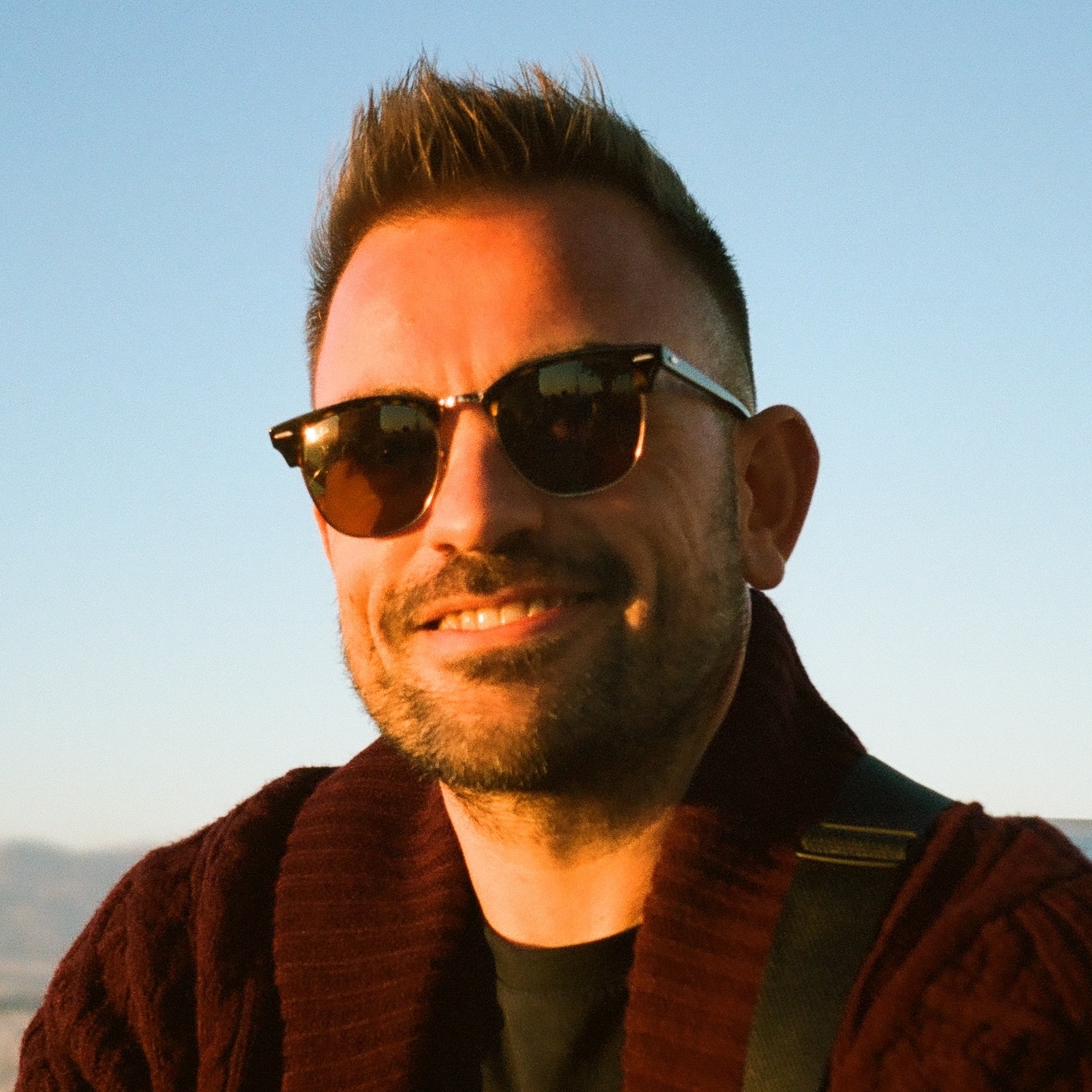
Creating a file upload form in HTML is a fundamental skill for web developers. Whether you're building a simple website or a complex application, allowing users to upload files is a common requirement. This tutorial will guide you through creating an HTML file upload form, handling multiple file uploads, customizing the file input, adding client-side validation, and implementing drag-and-drop functionality.
Introduction to HTML file upload forms
HTML file upload forms enable users to select files from their local computer and send them to a server. This functionality is essential for features like profile picture uploads, document submissions, and more. Understanding how to create and manage HTML file upload forms is crucial for developing interactive web applications.
Setting up a basic file upload form in HTML
To create a basic HTML file upload form, use the <form>
element along with an <input>
element of
type file
. Here's a simple example:
<form action="/upload" method="post" enctype="multipart/form-data">
<label for="file-upload">Choose a file to upload:</label>
<input type="file" id="file-upload" name="file" />
<button type="submit">Upload File</button>
</form>
In this example:
action="/upload"
specifies the URL where the form data will be sent.method="post"
indicates that the form data will be sent using the POST method.enctype="multipart/form-data"
specifies the encoding type of the form data, which is required for forms that include file uploads.<input type="file">
creates a file upload field.
Handling single and multiple file uploads
By default, the file input allows users to select a single file. To enable multiple file uploads,
add the multiple
attribute to the <input>
element:
<input type="file" id="file-upload" name="files[]" multiple />
The name="files[]"
attribute tells the server to expect an array of files under the files
field,
allowing the server-side script to handle multiple uploaded files.
Customizing the file upload button
To customize the file upload button, you can hide the default file input and style a label element:
<form action="/upload" method="post" enctype="multipart/form-data">
<label for="file-upload" class="custom-file-upload">Select File</label>
<input type="file" id="file-upload" name="file" />
<button type="submit">Upload File</button>
</form>
<style>
.custom-file-upload {
display: inline-block;
padding: 6px 12px;
cursor: pointer;
background-color: #007bff;
color: #fff;
border-radius: 4px;
}
#file-upload {
display: none;
}
</style>
This approach hides the default file input and styles the label to look like a button.
Adding client-side validation with JavaScript
Client-side validation improves user experience by checking file types and sizes before form submission:
<form id="upload-form" action="/upload" method="post" enctype="multipart/form-data">
<label for="file-upload" class="custom-file-upload">Select File</label>
<input type="file" id="file-upload" name="file" />
<button type="submit">Upload File</button>
</form>
<script>
const form = document.getElementById('upload-form')
const fileInput = document.getElementById('file-upload')
form.addEventListener('submit', function (e) {
const file = fileInput.files[0]
if (!file) {
alert('Please select a file to upload.')
e.preventDefault()
return
}
const allowedTypes = ['image/jpeg', 'image/png', 'application/pdf']
if (!allowedTypes.includes(file.type)) {
alert('Only JPEG, PNG, and PDF files are allowed.')
e.preventDefault()
return
}
const maxSize = 2 * 1024 * 1024 // 2MB
if (file.size > maxSize) {
alert('File size must be less than 2MB.')
e.preventDefault()
return
}
})
</script>
This script validates the file type and size, preventing form submission if the validation fails.
Implementing drag-and-drop file upload
Adding drag-and-drop functionality enhances the user experience:
<form id="upload-form" action="/upload" method="post" enctype="multipart/form-data">
<div id="drop-area">
<p>Drag and drop files here or click to select files</p>
<input type="file" id="file-upload" name="files[]" multiple />
</div>
<button type="submit">Upload Files</button>
</form>
<script>
const dropArea = document.getElementById('drop-area')
const fileInput = document.getElementById('file-upload')
;['dragenter', 'dragover', 'dragleave', 'drop'].forEach((eventName) => {
dropArea.addEventListener(eventName, preventDefaults, false)
})
function preventDefaults(e) {
e.preventDefault()
e.stopPropagation()
}
;['dragenter', 'dragover'].forEach((eventName) => {
dropArea.addEventListener(eventName, highlight, false)
})
;['dragleave', 'drop'].forEach((eventName) => {
dropArea.addEventListener(eventName, unhighlight, false)
})
function highlight() {
dropArea.classList.add('highlight')
}
function unhighlight() {
dropArea.classList.remove('highlight')
}
dropArea.addEventListener('drop', handleDrop, false)
function handleDrop(e) {
const dt = e.dataTransfer
const files = dt.files
fileInput.files = files
}
dropArea.addEventListener('click', () => fileInput.click())
</script>
<style>
#drop-area {
border: 2px dashed #ccc;
border-radius: 4px;
padding: 20px;
text-align: center;
cursor: pointer;
}
#drop-area.highlight {
border-color: #007bff;
}
#file-upload {
display: none;
}
</style>
This implementation creates a drag-and-drop zone and handles file drops and highlighting.
Conclusion
Creating a file upload form in HTML offers many opportunities to enhance the user experience. By implementing multiple file uploads, custom styling, client-side validation, and drag-and-drop functionality, you can create a robust and user-friendly HTML file upload form.
Remember to handle file uploads securely on the server side to prevent potential security risks. Always validate and sanitize uploaded files on the server.
For advanced file upload solutions with resumable uploads, progress indicators, and rich user interfaces, consider using Uppy, an open-source file uploader for web browsers.