Exporting files to Backblaze B2 in .Net using open-source tools
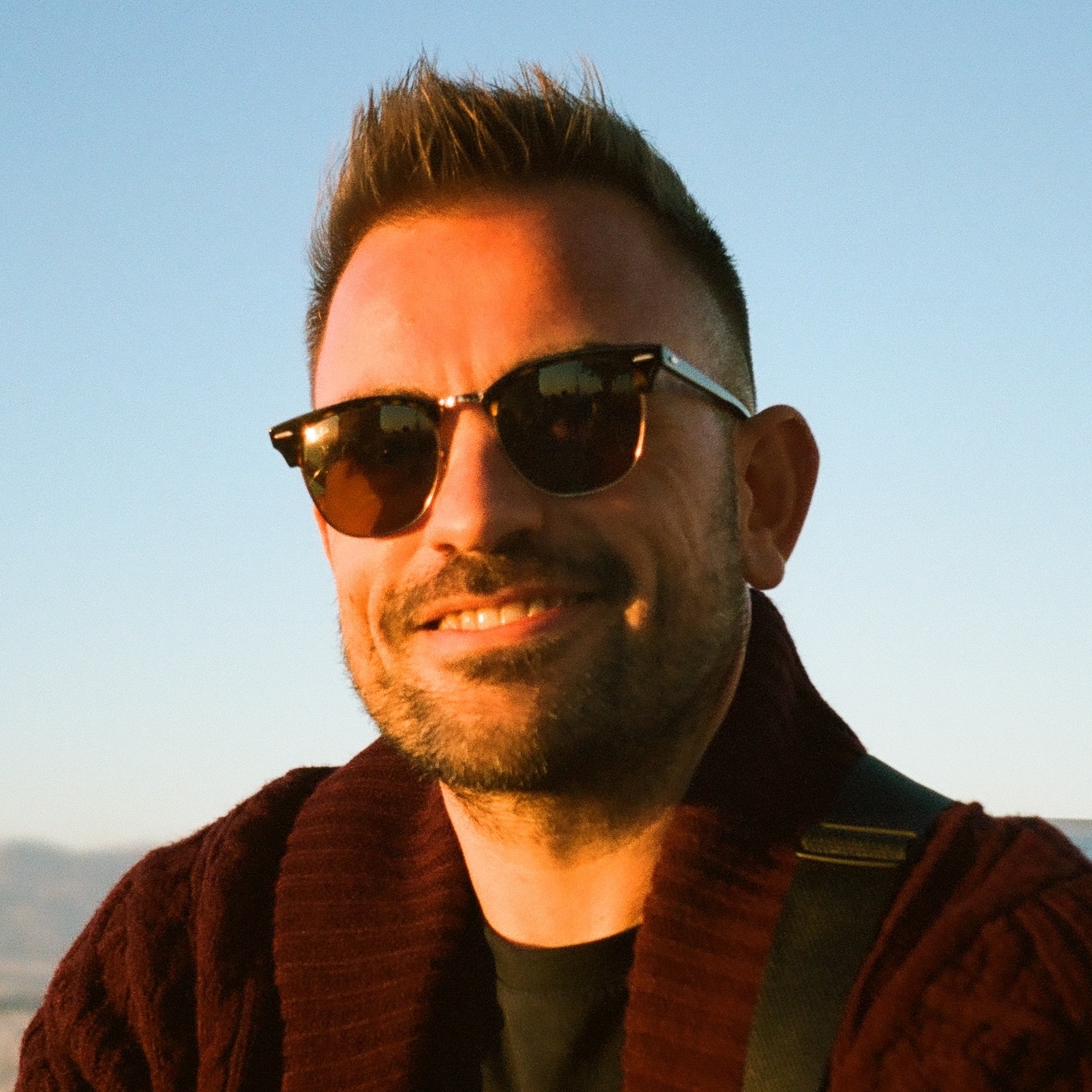
Integrating cloud storage into your .NET applications can significantly enhance your data handling capabilities. This tutorial will guide you through exporting files to Backblaze B2 Cloud Storage using open-source tools in C#, providing practical examples along the way.
Introduction to Backblaze B2 cloud storage
Backblaze B2 is an affordable and scalable cloud storage solution that offers an API for integrating with various applications. It provides unlimited free uploads, making it an excellent choice for backing up and storing files.
Setting up a Backblaze B2 account
To get started with Backblaze B2, follow these steps:
- Visit the Backblaze B2 sign-up page.
- Complete the registration process.
- Navigate to the Buckets section in your account dashboard.
- Create a new bucket to store your files.
Installing required .Net libraries
To interact with the Backblaze B2 API in .NET, we'll use the open-source B2.NET library. It simplifies API calls and handles authentication.
Install B2.NET using the Package Manager Console:
Install-Package B2Net
Or, if you're using the .NET CLI:
dotnet add package B2Net
Authenticating with Backblaze B2 API in .Net
To authenticate with the Backblaze B2 API, you'll need your Account ID and Application Key:
- In your Backblaze account dashboard, go to App Keys.
- Create a new Application Key.
- Note down the Key ID (Account ID) and Application Key (Secret Key).
Set up authentication in your .NET application:
using B2Net;
public class BackblazeService
{
private readonly B2Client _client;
public BackblazeService(string keyId, string applicationKey, string bucketId)
{
var options = new B2Options
{
KeyId = keyId,
ApplicationKey = applicationKey,
BucketId = bucketId
};
_client = new B2Client(options);
}
}
Uploading files to Backblaze B2 using C#
With authentication set up, you can now upload files:
using System;
using System.IO;
using System.Threading.Tasks;
using B2Net;
public class BackblazeService
{
private readonly B2Client _client;
public BackblazeService(string keyId, string applicationKey, string bucketId)
{
var options = new B2Options
{
KeyId = keyId,
ApplicationKey = applicationKey,
BucketId = bucketId
};
_client = new B2Client(options);
}
public async Task<string> UploadFileAsync(string filePath)
{
await _client.AuthorizeAsync();
using var fileStream = File.OpenRead(filePath);
var fileName = Path.GetFileName(filePath);
var fileInfo = await _client.Files.UploadAsync(fileStream, fileName);
return fileInfo.FileName;
}
}
Handling errors and exceptions
Implement robust error handling to manage potential issues during file uploads:
using System;
using System.IO;
using System.Threading.Tasks;
using B2Net;
public class BackblazeService
{
private readonly B2Client _client;
public BackblazeService(string keyId, string applicationKey, string bucketId)
{
var options = new B2Options
{
KeyId = keyId,
ApplicationKey = applicationKey,
BucketId = bucketId
};
_client = new B2Client(options);
}
public async Task<string> UploadFileAsync(string filePath)
{
try
{
await _client.AuthorizeAsync();
using var fileStream = File.OpenRead(filePath);
var fileName = Path.GetFileName(filePath);
var fileInfo = await _client.Files.UploadAsync(fileStream, fileName);
return fileInfo.FileName;
}
catch (B2Exception ex)
{
Console.WriteLine($"Backblaze B2 error: {ex.Message}");
throw;
}
catch (Exception ex)
{
Console.WriteLine($"An error occurred: {ex.Message}");
throw;
}
}
}
Integrating Backblaze uploads into your application
Here's an example of how to integrate the BackblazeService
into an ASP.NET Core application:
using Microsoft.AspNetCore.Mvc;
using System.IO;
using System.Threading.Tasks;
[ApiController]
[Route("[controller]")]
public class FileUploadController : ControllerBase
{
private readonly BackblazeService _backblazeService;
public FileUploadController(BackblazeService backblazeService)
{
_backblazeService = backblazeService;
}
[HttpPost("upload")]
public async Task<IActionResult> Upload(IFormFile file)
{
if (file == null || file.Length == 0)
return BadRequest("No file selected.");
var tempPath = Path.GetTempFileName();
try
{
using (var stream = new FileStream(tempPath, FileMode.Create))
{
await file.CopyToAsync(stream);
}
var uploadedFileName = await _backblazeService.UploadFileAsync(tempPath);
return Ok($"File '{uploadedFileName}' uploaded successfully.");
}
finally
{
System.IO.File.Delete(tempPath);
}
}
}
Conclusion
Integrating Backblaze B2 into your .NET applications using open-source tools like B2.NET is straightforward and enhances your application's storage capabilities. By following these steps, you can efficiently upload files, handle errors gracefully, and integrate cloud storage seamlessly.
While this guide focuses on Backblaze B2, at Transloadit, we specialize in file uploading and processing. Our encoding REST API can be easily integrated into your applications for advanced file processing needs, complementing your cloud storage solution.