Exporting files to Dropbox in Ruby using the Dropbox SDK
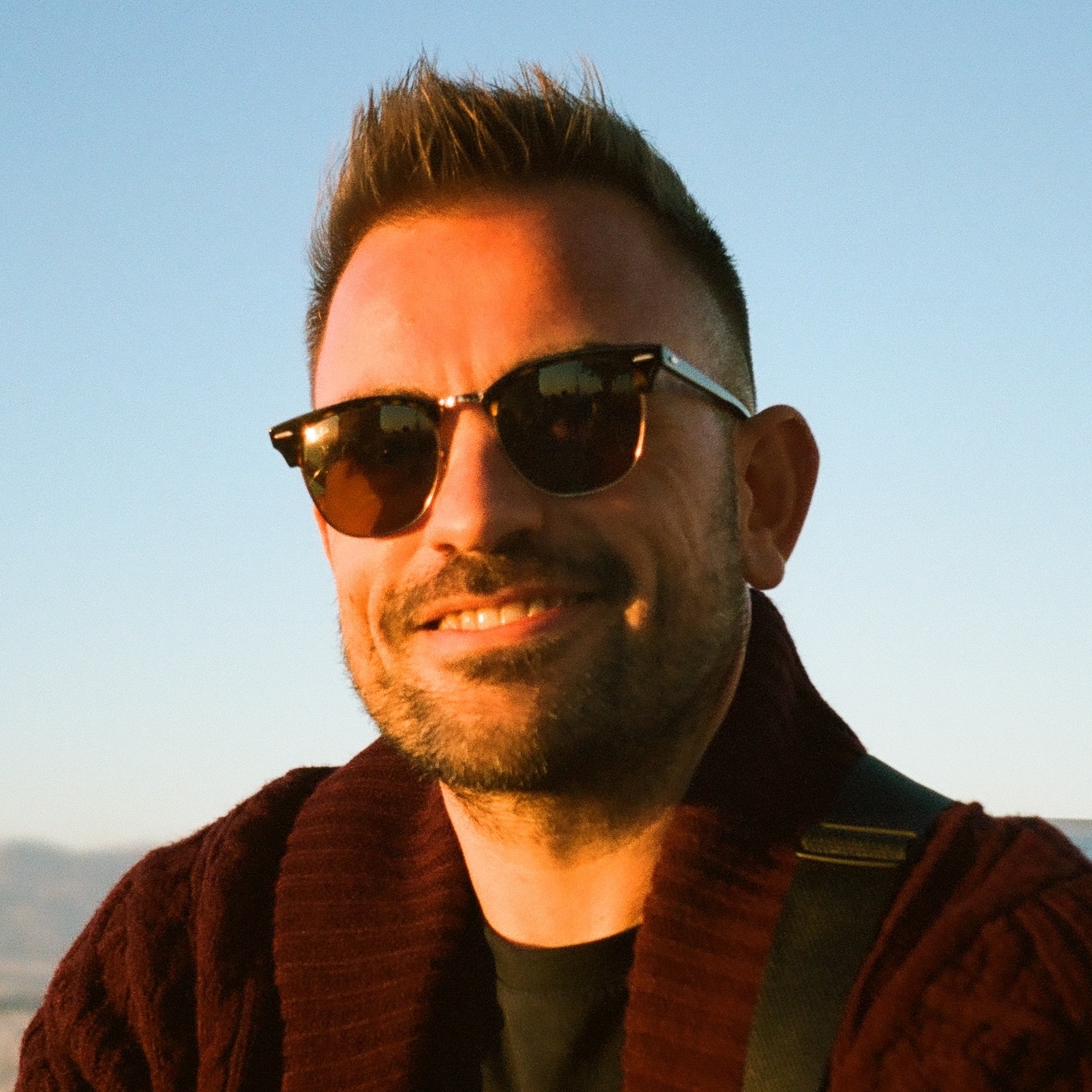
Integrating Dropbox file exports into your Ruby applications can streamline your workflow and enhance functionality. This guide demonstrates how to use the Dropbox SDK for Ruby to automate file uploads with practical examples.
Prerequisites
Before you begin, ensure you have:
- Ruby 2.7 or higher (tested up to Ruby 3.2)
- Bundler 2.0 or higher
- A Dropbox account
- Basic knowledge of Ruby programming
- SSL support in your development environment
Setting up a Dropbox app and obtaining access tokens
To interact with the Dropbox API, you need to create a Dropbox app and generate an access token:
-
Create a Dropbox App:
- Log in to the Dropbox App Console.
- Click on "Create App."
- Choose "Scoped access" and select "Full Dropbox" or "App folder," depending on your needs.
- Give your app a unique name and click "Create App."
-
Generate an Access Token:
- In your app's settings, navigate to the "OAuth 2" section.
- Under "Generated access token," click "Generate."
- Copy the generated access token; you will use it in your application.
- Note: For production, implement a full OAuth2 flow with refresh tokens for enhanced security.
Installing the Dropbox SDK for Ruby
This guide uses the dropbox_api gem version 0.1.21—the latest stable release—to access Dropbox's API. Add the SDK to your Gemfile:
source 'https://rubygems.org'
gem 'dropbox_api', '~> 0.1.21'
Then install it using Bundler:
bundle install
Authenticating with Dropbox in Ruby
Establish secure authentication with proper error handling:
require 'dropbox_api'
def initialize_dropbox_client(access_token)
DropboxApi::Client.new(access_token)
rescue DropboxApi::Errors::BasicError => e
puts "Authentication error: #{e.message}"
raise
rescue StandardError => e
puts "Failed to initialize Dropbox client: #{e.message}"
raise
end
# Initialize the client
dbx = initialize_dropbox_client('YOUR_ACCESS_TOKEN')
Uploading files to Dropbox using Ruby
Define a robust file upload method with proper resource management:
def upload_file(client, file_path, dropbox_path)
File.open(file_path, 'rb') do |file|
client.upload(dropbox_path, file)
puts "File '#{file_path}' uploaded to '#{dropbox_path}'"
end
rescue DropboxApi::Errors::BasicError => e
puts "Authentication error: #{e.message}"
rescue DropboxApi::Errors::HttpError => e
puts "API error: #{e.message}"
rescue StandardError => e
puts "An error occurred: #{e.message}"
end
# Usage example
begin
local_file = 'path/to/your/local/file.txt'
dropbox_destination = '/Apps/YourAppFolder/file.txt'
upload_file(dbx, local_file, dropbox_destination)
rescue => e
puts "Failed to upload file: #{e.message}"
end
Handling errors and exceptions
Implement comprehensive error handling with retry logic for transient issues:
def handle_upload_with_retry(client, file_path, dropbox_path, max_retries = 3)
retries = 0
begin
upload_file(client, file_path, dropbox_path)
rescue DropboxApi::Errors::BasicError => e
puts "Authentication error: #{e.message}"
raise
rescue DropboxApi::Errors::HttpError => e
if retries < max_retries && e.message.include?('rate_limit')
retries += 1
sleep(2 ** retries) # Exponential backoff
retry
end
puts "API error: #{e.message}"
raise
rescue StandardError => e
puts "An error occurred: #{e.message}"
raise
end
end
Automating file exports in your Ruby application
Automate file exports by processing an entire directory:
class DropboxExporter
def initialize(access_token)
@client = initialize_dropbox_client(access_token)
end
def process_directory(local_dir, dropbox_dir)
Dir.glob(File.join(local_dir, '*')).each do |file_path|
next unless File.file?(file_path)
dropbox_path = File.join(dropbox_dir, File.basename(file_path))
handle_upload_with_retry(@client, file_path, dropbox_path)
end
end
end
# Usage example
exporter = DropboxExporter.new('YOUR_ACCESS_TOKEN')
exporter.process_directory('local/files', '/Apps/YourAppFolder')
Rails integration considerations
If you integrate Dropbox exports within a Rails application, be mindful of thread safety and process forking issues. Ensure that any background jobs or scheduled tasks are configured using thread-safe patterns and robust scheduling libraries to prevent concurrency conflicts, especially in production environments.
Conclusion
Integrating the Dropbox SDK into your Ruby application can automate file exports and streamline your workflow. This guide provided a step-by-step approach covering authentication, file uploading, error handling with retry logic, and directory processing. For robust file handling and processing capabilities, you might also consider exploring Transloadit's services.