Fast and efficient: export files to Vimeo in Java
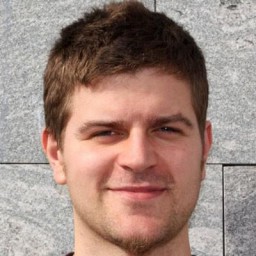
Exporting videos to Vimeo programmatically can significantly enhance your video publishing workflow. This guide demonstrates how to export files to Vimeo using Java, leveraging the Vimeo API and Java SDK.
Prerequisites
Before starting, ensure you have:
- Java Development Kit (JDK) 11 or later
- Maven or Gradle for dependency management
- A Vimeo Pro account or higher (required for API access)
- Vimeo API credentials with upload permissions
- Sufficient storage quota (maximum file size: 250GB)
- Videos under 24 hours in duration
Setting up your development environment
Add the Vimeo Java SDK to your project using your preferred dependency management tool.
For Maven, add to pom.xml
:
<repositories>
<repository>
<id>maven-central</id>
<url>https://repo.maven.apache.org/maven2</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>com.clickntap</groupId>
<artifactId>vimeo</artifactId>
<version>2.0</version>
</dependency>
</dependencies>
For Gradle, add to build.gradle
:
repositories {
mavenCentral()
}
dependencies {
implementation 'com.clickntap:vimeo:2.0'
}
Configuring and authenticating your application
Create a VimeoUploader class to handle video uploads:
import com.clickntap.vimeo.Vimeo;
import com.clickntap.vimeo.VimeoResponse;
public class VimeoUploader {
private final Vimeo vimeo;
public VimeoUploader(String accessToken) {
this.vimeo = new Vimeo(accessToken);
}
public String uploadVideo(File videoFile, String name, String description) throws Exception {
validateVideo(videoFile);
String videoEndpoint = vimeo.addVideo(videoFile);
if (name != null || description != null) {
vimeo.updateVideoMetadata(
videoEndpoint,
name,
description,
"", // license
"anybody", // privacyView
"public", // privacyEmbed
false // reviewLink
);
}
return videoEndpoint;
}
private void validateVideo(File videoFile) {
if (!videoFile.exists()) {
throw new IllegalArgumentException("Video file does not exist");
}
long maxSize = 250L * 1024 * 1024 * 1024; // 250GB
if (videoFile.length() > maxSize) {
throw new IllegalArgumentException("File size exceeds Vimeo's 250GB limit");
}
}
}
Error handling and retries
Implement robust error handling with retries:
public class VimeoUploader {
// ... previous code ...
public String uploadWithRetry(File videoFile, String name, String description, int maxRetries) {
int attempts = 0;
Exception lastException = null;
while (attempts < maxRetries) {
try {
return uploadVideo(videoFile, name, description);
} catch (Exception e) {
lastException = e;
attempts++;
if (attempts == maxRetries) {
break;
}
try {
// Exponential backoff with jitter
long backoff = (long) (Math.pow(2, attempts) * 1000 + Math.random() * 1000);
Thread.sleep(backoff);
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
throw new RuntimeException("Upload interrupted", ie);
}
}
}
throw new RuntimeException("Upload failed after " + maxRetries + " attempts", lastException);
}
}
Testing your implementation
Create a test class to verify your implementation:
public class VimeoUploaderTest {
public static void main(String[] args) {
String accessToken = System.getenv("VIMEO_ACCESS_TOKEN");
if (accessToken == null) {
throw new IllegalStateException("VIMEO_ACCESS_TOKEN environment variable not set");
}
VimeoUploader uploader = new VimeoUploader(accessToken);
File videoFile = new File("path/to/video.mp4");
try {
String videoEndpoint = uploader.uploadWithRetry(
videoFile,
"Test Upload",
"Test upload description",
3
);
System.out.println("Video uploaded successfully: " + videoEndpoint);
} catch (Exception e) {
System.err.println("Upload failed: " + e.getMessage());
e.printStackTrace();
}
}
}
Best practices for video uploads
- Compress videos before uploading to reduce transfer time
- Use supported formats (MP4, MOV, WMV, AVI)
- Implement proper error handling and retries
- Store video endpoints for future reference
- Monitor upload quotas and limits
- Use environment variables for sensitive credentials
Enhancing workflows with Transloadit's Vimeo export Robot
For advanced video processing before uploading to Vimeo, Transloadit's 🤖 /vimeo/store Robot offers additional features like encoding, watermarking, and thumbnail creation. The Robot handles the entire process of preparing and uploading videos to Vimeo.
Transloadit's service can automatically handle:
- Video encoding and optimization
- Thumbnail generation
- Watermark application
- Direct export to Vimeo
This approach simplifies your workflow and ensures optimal video quality for Vimeo playback.
Conclusion
This guide demonstrates how to efficiently export videos to Vimeo using Java. For advanced video processing needs, consider using Transloadit's Video Encoding Service and 🤖 /vimeo/store Robot to streamline your workflow.