Implementing file uploads with Bootstrap 5
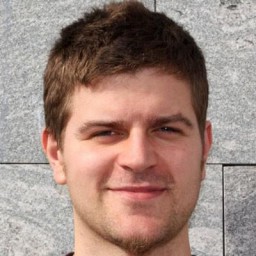
File uploads are essential in modern web applications. Bootstrap 5 provides tools to create sleek and user-friendly file upload interfaces. This guide will walk you through implementing file uploads using Bootstrap 5, including drag-and-drop functionality and custom upload buttons.
Introduction to Bootstrap 5 file uploads
Bootstrap 5 offers a variety of components for building responsive web interfaces. While it doesn't provide a dedicated file upload component, you can use Bootstrap's form controls to create custom file upload forms that integrate seamlessly into your application.
In this tutorial, we'll cover:
- Setting up a Bootstrap 5 environment
- Creating a simple file upload form
- Enhancing it with drag-and-drop functionality
- Styling the file upload button
- Handling file uploads on the server side
- Testing and debugging common issues
Setting up a Bootstrap 5 environment
To get started, set up a basic Bootstrap 5 environment. You can include Bootstrap via CDN or install it locally.
Including via CDN:
Add the following links to your HTML file's <head>
section:
<link
href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/css/bootstrap.min.css"
rel="stylesheet"
/>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/js/bootstrap.bundle.min.js"></script>
Including via NPM:
If you're using a build tool, install Bootstrap via NPM:
npm install bootstrap@5.3.2
Creating a simple file upload form
Let's create a basic file upload form using Bootstrap's form controls:
<form action="/upload" method="post" enctype="multipart/form-data">
<div class="mb-3">
<label for="formFile" class="form-label">Choose a file to upload</label>
<input class="form-control" type="file" id="formFile" name="file" />
</div>
<button type="submit" class="btn btn-primary">Upload</button>
</form>
This form includes a file input and a submit button styled with Bootstrap classes.
Enhancing with drag-and-drop functionality
To improve user experience, let's add drag-and-drop functionality. This feature allows users to simply drag files from their file explorer and drop them into the designated area, making the upload process more intuitive and efficient:
<div class="mb-3">
<label for="formFile" class="form-label">Upload your file</label>
<div id="dropZone" class="border border-primary rounded p-4 text-center">
Drag and drop a file here or click to select
</div>
<input type="file" id="formFile" name="file" class="d-none" />
</div>
<script>
const dropZone = document.getElementById('dropZone')
const formFile = document.getElementById('formFile')
dropZone.addEventListener('click', () => formFile.click())
dropZone.addEventListener('dragover', (e) => {
e.preventDefault()
dropZone.classList.add('bg-light')
})
dropZone.addEventListener('dragleave', () => {
dropZone.classList.remove('bg-light')
})
dropZone.addEventListener('drop', (e) => {
e.preventDefault()
dropZone.classList.remove('bg-light')
formFile.files = e.dataTransfer.files
updateDropZoneText()
})
formFile.addEventListener('change', updateDropZoneText)
function updateDropZoneText() {
dropZone.textContent =
formFile.files.length > 0
? formFile.files[0].name
: 'Drag and drop a file here or click to select'
}
</script>
This script allows users to click the drop zone to open the file picker or drag and drop a file. The selected file's name is displayed in the drop zone, providing immediate feedback to the user.
Styling the file upload button
Customize the file upload button for a better user experience:
<div class="mb-3">
<label class="form-label">Upload your file</label>
<div>
<button type="button" class="btn btn-secondary" id="customButton">Choose File</button>
<span id="fileName" class="ms-2">No file chosen</span>
</div>
<input type="file" id="formFile" name="file" class="d-none" />
</div>
<script>
const customButton = document.getElementById('customButton')
const fileName = document.getElementById('fileName')
const formFile = document.getElementById('formFile')
customButton.addEventListener('click', () => formFile.click())
formFile.addEventListener('change', () => {
fileName.textContent = formFile.files.length > 0 ? formFile.files[0].name : 'No file chosen'
})
</script>
This approach replaces the default file input with a custom-styled button and displays the name of the selected file, enhancing the visual appeal and usability of the upload interface.
Handling file uploads on the server side
Here's an example of handling file uploads using Node.js and Express:
const express = require('express')
const multer = require('multer')
const app = express()
const upload = multer({ dest: 'uploads/' })
app.post('/upload', upload.single('file'), (req, res) => {
if (!req.file) {
return res.status(400).send('No file uploaded.')
}
res.send('File uploaded successfully.')
})
app.listen(3000, () => {
console.log('Server started on http://localhost:3000')
})
This server accepts file uploads and stores them in the uploads/
directory.
Testing and debugging common issues
When testing your file upload form, consider these tips:
- File Size Limits: Configure appropriate size limits on both client and server sides.
- File Type Validation: Implement file type checks to prevent unwanted uploads.
- Error Handling: Provide clear feedback for upload failures due to network issues or server errors.
- Cross-Browser Testing: Ensure compatibility across different browsers and devices.
Conclusion
By leveraging Bootstrap 5 and custom JavaScript, you can create a user-friendly file upload feature for your web application. Implementing drag-and-drop functionality and customizing the upload button significantly enhances the user experience, making file uploads more intuitive and efficient for your users.
These techniques allow you to create a modern, responsive file upload interface that integrates seamlessly with your Bootstrap-based design. Remember to thoroughly test your implementation across various devices and browsers to ensure a consistent experience for all users.
For a robust solution to handle file uploads and processing at scale, consider Transloadit. Transloadit offers reliable file uploading and encoding services that can streamline your development process and handle complex file processing tasks.