Secure file uploads to Amazon S3 with Boto3: best practices
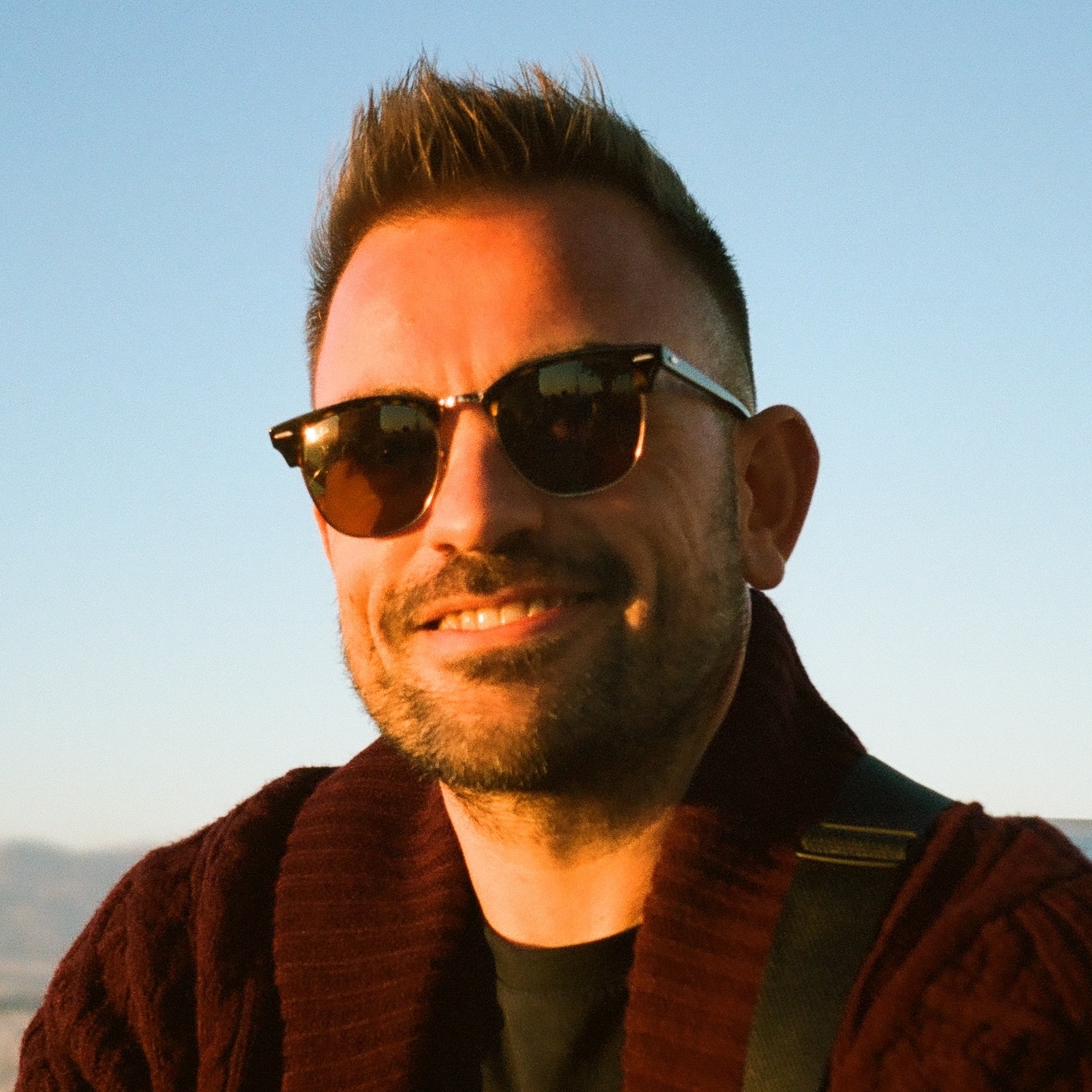
Uploading files to Amazon S3 is a common task for many applications. However, ensuring that these uploads are secure is crucial for protecting sensitive data and maintaining compliance with security standards. In this post, we'll explore how to use Boto3 to securely upload files to Amazon S3, focusing on encryption and access control mechanisms.
Why use Boto3 for S3 file uploads?
Boto3 is the Amazon Web Services (AWS) Software Development Kit (SDK) for Python, allowing you to interact programmatically with AWS services like S3. Using Boto3 provides a seamless way to manage file uploads, handle encryption, and manage access control policies directly from your Python applications.
Setting up your AWS environment
Before diving into code, ensure that you have the necessary environment set up:
-
Install Boto3:
pip install boto3
-
Configure AWS Credentials:
Boto3 uses AWS credentials to authenticate requests. These can be set up using the AWS CLI or by creating a configuration file.
aws configure
Alternatively, you can set environment variables:
export AWS_ACCESS_KEY_ID='YOUR_ACCESS_KEY' export AWS_SECRET_ACCESS_KEY='YOUR_SECRET_KEY' export AWS_DEFAULT_REGION='YOUR_PREFERRED_REGION'
To prevent security risks, never hard-code your AWS credentials in your code.
Uploading a file to S3 with Boto3
Below is a secure example of uploading a file to an S3 bucket using Boto3 with robust error handling:
import boto3
from botocore.exceptions import ClientError
import logging
import os
def upload_file(file_name, bucket, object_name=None):
if object_name is None:
object_name = os.path.basename(file_name)
s3_client = boto3.client('s3')
try:
s3_client.upload_file(file_name, bucket, object_name)
# By default, Amazon S3 encrypts your files using SSE-S3 with AES256
return True
except ClientError as e:
logging.error(e)
return False
Implementing file encryption on S3
Amazon S3 now encrypts all new objects by default using server-side encryption (SSE-S3) with AES256 at no additional cost. This means you do not need to specify encryption parameters when uploading files unless you require custom settings.
For additional security using AWS Key Management Service (KMS) keys, include encryption parameters like this:
response = boto3.client('s3').upload_file(
file_path,
bucket_name,
key,
ExtraArgs={
'ServerSideEncryption': 'aws:kms',
'SSEKMSKeyId': 'your-kms-key-id'
}
)
Note: If you do not specify encryption parameters, Amazon S3 uses SSE-S3 (AES256) by default.
Managing access control policies for secure uploads
Controlling access to your S3 resources is crucial for security. Here are some best practices:
Bucket policies
Enforce HTTPS-only access by applying a bucket policy. For example:
import json
bucket_policy = {
"Version": "2012-10-17",
"Statement": [
{
"Sid": "DenyHTTP",
"Effect": "Deny",
"Principal": "*",
"Action": "s3:*",
"Resource": [
"arn:aws:s3:::your-bucket-name",
"arn:aws:s3:::your-bucket-name/*"
],
"Condition": {
"Bool": {
"aws:SecureTransport": "false"
}
}
}
]
}
bucket_policy_json = json.dumps(bucket_policy)
s3_client = boto3.client('s3')
s3_client.put_bucket_policy(
Bucket="your-bucket-name",
Policy=bucket_policy_json
)
S3 object ownership and acls
Note that as of April 2023, S3 Object Ownership is set to "Bucket owner enforced" by default, which disables ACLs. Rely on bucket policies to manage access control. If you specifically need ACLs, ensure they are enabled in your bucket settings.
If you must use ACLs, you can include them like this:
# Warning: use acls only if your bucket settings allow them
response = boto3.client('s3').upload_file(
file_name,
bucket_name,
key,
ExtraArgs={
'ACL': 'private'
}
)
Automating file upload processes with Python scripts
The following script demonstrates how to securely upload multiple files from a directory with proper error handling and logging:
import os
import boto3
from botocore.exceptions import ClientError
import logging
logging.basicConfig(level=logging.INFO)
def upload_directory(directory_path, bucket_name, s3_prefix=''):
s3_client = boto3.client('s3')
uploaded_files = []
errors = []
for root, dirs, files in os.walk(directory_path):
for filename in files:
local_path = os.path.join(root, filename)
relative_path = os.path.relpath(local_path, directory_path)
s3_path = os.path.join(s3_prefix, relative_path).replace("\\", "/")
try:
s3_client.upload_file(local_path, bucket_name, s3_path)
uploaded_files.append(s3_path)
logging.info(f'Successfully uploaded {local_path} to s3://{bucket_name}/{s3_path}')
except ClientError as e:
errors.append({'file': local_path, 'error': str(e)})
logging.error(f'Error uploading {local_path}: {e}')
return uploaded_files, errors
Troubleshooting common issues
Access denied errors
Ensure you apply the principle of least privilege by using specifically scoped IAM policies. For example:
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": ["s3:PutObject", "s3:GetObject", "s3:ListBucket"],
"Resource": ["arn:aws:s3:::your-bucket-name/*", "arn:aws:s3:::your-bucket-name"]
}
]
}
Transfer acceleration
For faster uploads over long distances, enable S3 Transfer Acceleration:
s3_client = boto3.client('s3',
config=boto3.session.Config(
s3={'use_accelerate_endpoint': True}
)
)
Advanced S3 features
Beyond basic file uploads, AWS S3 offers several advanced features to enhance your workflows:
- S3 Object Lambda: Process and transform the data returned by S3 GET requests on the fly without modifying the stored object.
- S3 Lifecycle policies: Automate the transition of objects to more cost-effective storage classes, or schedule their deletion based on defined rules.
- S3 Event Notifications: Monitor bucket events and trigger actions such as invoking Lambda functions, sending messages via SNS or SQS, when new objects are created.
For example, you can configure S3 Event Notifications with Boto3 as follows:
import boto3
s3_client = boto3.client('s3')
notification_configuration = {
'LambdaFunctionConfigurations': [
{
'LambdaFunctionArn': 'arn:aws:lambda:us-east-1:123456789012:function:ProcessS3Event',
'Events': ['s3:ObjectCreated:*'],
'Filter': {
'Key': {
'FilterRules': [
{'Name': 'suffix', 'Value': '.jpg'}
]
}
}
}
]
}
s3_client.put_bucket_notification_configuration(
Bucket='your-bucket-name',
NotificationConfiguration=notification_configuration
)
Conclusion
Secure file uploads to Amazon S3 require a thorough understanding of modern security features and best practices. By leveraging Boto3 alongside AWS S3's built-in security features, you can build robust and reliable file upload solutions.
Key takeaways:
- Use robust error handling to ensure reliable uploads
- Benefit from default SSE-S3 encryption (AES256) or opt for AWS KMS for customized security
- Rely on bucket policies instead of ACLs, as ACLs are disabled by default in new buckets
- Utilize S3 Transfer Acceleration and advanced features like Object Lambda, Lifecycle policies, and Event Notifications to enhance performance and manageability
By following these best practices, you'll improve both the security and efficiency of your file upload processes.
Looking to simplify your file upload processes even further? Check out Transloadit for powerful file uploading and encoding solutions.