Secure file uploads with cURL and client certificates
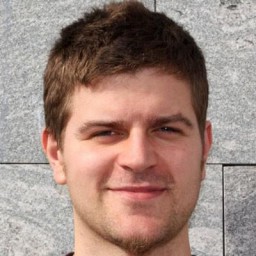
Secure file uploads are essential for protecting data during transfer over the internet. In this DevTip, we will explore how to securely upload files using cURL over HTTPS. We will guide you through setting up your environment, creating secure connections, and implementing best practices for cURL file uploads.
Introduction to cURL and file uploads
cURL is a powerful command-line tool used for transferring data with URLs. It is widely used by developers for testing APIs, transferring files, and debugging network connections. Understanding how to securely upload files using cURL is crucial for maintaining data integrity and security in your applications.
Understanding HTTPS and why it's essential for secure file transfers
HTTPS (HyperText Transfer Protocol Secure) is an extension of HTTP that provides secure communication over a computer network. It encrypts data transmitted between the client and server, preventing eavesdropping and tampering. When uploading files, employing HTTPS ensures your data remains encrypted during transit, safeguarding sensitive information from interception.
Setting up the environment: installing cURL on your system
Before you begin, ensure that cURL is installed on your system. Most modern operating systems come with cURL pre-installed. To verify the installation, run:
curl --version
If cURL is not installed, use the following commands:
- On macOS:
- Via Homebrew:
brew install curl
- Note: macOS typically comes with cURL pre-installed.
- Via Homebrew:
- On Linux (Debian/Ubuntu):
sudo apt update && sudo apt install curl
- On Windows:
- Windows 10 (1803) and later: cURL is pre-installed.
- For older versions: Download from https://curl.se/windows/
Creating secure connections using HTTPS
To securely upload files, establish a connection using HTTPS. This not only encrypts your data in transit but also protects it against potential interception. For enhanced security, you can also use client certificates, which authenticate the client to the server during the SSL/TLS handshake.
Step-by-step guide to uploading a file with cURL using HTTPS
Prerequisites
- cURL installed on your system
- Access to a server that accepts file uploads over HTTPS
- Optional: Client certificate and private key if the server requires client authentication
Uploading a file over HTTPS
Upload a file securely with the following command:
curl --upload-file /path/to/local/file.txt https://example.com/upload
This command securely uploads file.txt
to https://example.com/upload
over HTTPS.
Using client certificates for enhanced security
For scenarios that require client authentication, include your client certificate and private key with the cURL command:
# Basic client certificate usage
curl --cert client.crt --key client.key https://example.com/
# Specify certificate type explicitly
curl --cert-type PEM --cert client.crt --key client.key https://example.com/
# Using a combined cert+key file
curl --cert combined.pem:password https://example.com/
IMPORTANT: Always protect your private key with appropriate permissions. For example:
chmod 600 client.key # Only the owner can read/write the key
chmod 644 client.crt # The certificate can be world-readable
Handling certificate passphrases
If your private key is secured with a passphrase, cURL will prompt you when executing the command. For automated scripts, avoid including the passphrase inline due to security concerns. Instead, consider using a key without a passphrase secured by strict file permissions.
Verifying server certificates
By default, cURL checks the server's SSL certificate against the system's trusted certificate
authorities (CAs). If the server uses a self-signed certificate or one issued by a private CA,
provide the CA certificate using the --cacert
option:
curl --cacert /path/to/ca.crt \
--upload-file /path/to/local/file.txt \
https://example.com/upload
Troubleshooting common issues
Certificate verification failed
curl: (60) SSL certificate problem: unable to get local issuer certificate
Solution: Ensure your system's CA certificates are up-to-date or specify the appropriate CA certificate:
curl --cacert /path/to/ca.crt https://example.com
Client certificate errors
curl: (58) could not load PEM client certificate
Solution: Validate the certificate format and adjust file permissions:
openssl x509 -in client.crt -text -noout # Verify the certificate
chmod 600 client.key # Correct the permissions
Security best practices
-
Certificate Validation: Always verify the server's certificate. Disable certificate verification only in controlled testing environments:
# Validate certificates (recommended) curl https://example.com/ # Disable certificate validation (only for testing purposes) curl -k https://example.com/
-
Private Key Protection:
- Store private keys with strict file permissions (e.g.,
chmod 600 client.key
). - Never commit private keys to version control.
- Consider using secure vaults or environment variables for sensitive credentials.
- Store private keys with strict file permissions (e.g.,
-
Certificate Handling:
- Keep your CA certificates updated.
- Use the system CA store whenever possible, or specify custom CA certificates with the
--cacert
option.
-
Protocol Security:
- Ensure you are using TLS 1.2 or later (specify with
--tlsv1.2
when possible). - Avoid including sensitive data in URLs.
- Regularly update your cURL and OpenSSL libraries.
- Ensure you are using TLS 1.2 or later (specify with
Version compatibility
The examples in this post have been tested with:
- curl 8.5.0 (current stable release)
- OpenSSL 3.0.13
- Ubuntu 22.04 LTS and later
- macOS 13 and later
- Windows 10 (1803) and later
Conclusion
Securing file uploads is crucial for protecting sensitive data in your applications. By using cURL over HTTPS and implementing client certificates, you can ensure that your file transfers are both secure and authenticated. Following these best practices will help maintain a robust file upload system.
If you're looking for a simpler way to handle secure file uploads and processing in your applications, consider using Transloadit, which offers robust solutions for file uploading and processing with built-in security features.