Secure image upload API with Node.js, Express, and Multer
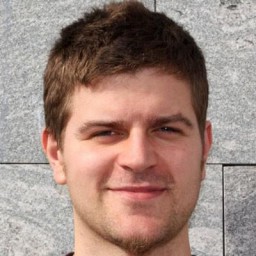
Creating a secure and efficient image upload API is essential for modern web applications. Handling file uploads requires careful consideration of security risks like arbitrary file execution, denial-of-service attacks, and malware propagation. In this DevTip, we'll explore how to build a robust image upload API using Node.js, Express, and Multer, ensuring security through input validation, file type restrictions, size limits, rate limiting, virus scanning, and secure storage practices.
Introduction to image upload APIs
An image upload API allows users or client applications to send images to your server. A secure API
must validate incoming data, restrict potentially harmful files, limit resource consumption, scan
for malware, and store files safely. We'll use Node.js, the Express framework, and the Multer
middleware for handling multipart/form-data
, which is primarily used for uploading files.
Setting up the Node.js environment
First, ensure you have Node.js and npm (Node Package Manager) installed. You can check their versions using:
node -v
npm -v
Next, create a new project directory and initialize it with npm:
mkdir image-upload-api
cd image-upload-api
npm init -y
Installing and configuring Express and Multer
Install the necessary packages: Express for the web server, Multer for file uploads,
express-rate-limit
for preventing abuse, clamscan
for virus scanning (requires ClamAV installed
on the server), cors
for handling cross-origin requests, helmet
for security headers, and
morgan
for logging. We pin versions for better stability and security:
npm install express@^4.18.2 multer@^1.4.5-lts.1
npm install express-rate-limit@^7.1.5 clamscan@^2.1.2 cors@^2.8.5 helmet@^7.1.0 morgan@^1.10.0
# Note: the 'crypto' module is built-in to Node.js
Now, create your main application file, app.js
:
const express = require('express')
const multer = require('multer')
const path = require('path')
const crypto = require('crypto') // Built-in Node.js module
const fs = require('fs')
const rateLimit = require('express-rate-limit')
const NodeClam = require('clamscan')
const cors = require('cors')
const helmet = require('helmet')
const morgan = require('morgan')
const app = express()
const PORT = process.env.PORT || 3000
// --- Security Middleware ---
app.use(helmet()) // Apply security headers
app.use(cors({ origin: '*' })) // Configure CORS - Be more restrictive in production!
app.use(morgan('dev')) // HTTP request logger (use 'combined' in production)
// Create secure upload directory outside web root if it doesn't exist
// IMPORTANT: Ensure this path is NOT directly accessible via your web server configuration
const uploadDir = path.join(__dirname, '../secure-uploads/')
if (!fs.existsSync(uploadDir)) {
// Create directory with restricted permissions (owner rwx, group rx, others ---)
fs.mkdirSync(uploadDir, { recursive: true, mode: 0o750 })
}
// --- Multer Configuration (will be defined below) ---
// --- Rate Limiting (will be defined below) ---
// --- Virus Scanning (will be defined below) ---
// --- API Endpoints (will be defined below) ---
// --- Error Handling (will be defined below) ---
app.listen(PORT, () => console.log(`Server running on port ${PORT}`))
Creating the basic API endpoint structure
We'll define the Multer storage configuration first. Storing files outside the web root and using randomized filenames are crucial security measures.
// Configure secure file storage
const storage = multer.diskStorage({
destination: (req, file, cb) => {
// Store files in the secure directory created earlier
cb(null, uploadDir)
},
filename: (req, file, cb) => {
// Generate a secure random filename using crypto to prevent collisions and guessing
crypto.randomBytes(16, (err, buf) => {
if (err) {
return cb(err)
}
const uniqueSuffix = buf.toString('hex')
// Preserve the original file extension, converting it to lowercase
const extension = path.extname(file.originalname).toLowerCase()
cb(null, uniqueSuffix + extension)
})
},
})
Implementing input validation
Robust validation prevents malicious file uploads. We need to check both the MIME type (reported by the client, can be spoofed) and the file extension (less reliable but adds a layer).
// Define allowed file types (MIME types and extensions)
const allowedMimeTypes = ['image/jpeg', 'image/png', 'image/gif']
const allowedExtensions = /\.(jpg|jpeg|png|gif)$/i // Case-insensitive check
const fileFilter = (req, file, cb) => {
// Validate MIME type
const mimeTypeValid = allowedMimeTypes.includes(file.mimetype)
// Validate file extension
const extValid = allowedExtensions.test(path.extname(file.originalname).toLowerCase())
if (mimeTypeValid && extValid) {
// Accept the file
cb(null, true)
} else {
// Reject the file with a specific error message
cb(new Error('Invalid file type. Only JPEG, PNG, and GIF images are allowed.'), false)
}
}
Restricting file types and sizes with Multer
Now, configure Multer with the storage strategy, file filter, and size limits.
// Configure multer with security settings
const upload = multer({
storage: storage,
limits: {
fileSize: 5 * 1024 * 1024, // 5MB limit per file (adjust as needed)
},
fileFilter: fileFilter,
})
Implementing rate limiting
To prevent Denial-of-Service (DoS) attacks through excessive uploads, we implement rate limiting
using express-rate-limit
.
// Create a rate limiter for upload endpoints
const uploadLimiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 10, // Limit each IP to 10 uploads per windowMs (adjust as needed)
message: {
error: 'Too many upload attempts from this IP, please try again after 15 minutes.',
},
standardHeaders: true, // Return rate limit info in the `RateLimit-*` headers
legacyHeaders: false, // Disable the `X-RateLimit-*` headers
})
Virus scanning for uploaded files
Integrating virus scanning adds another layer of defense. This example uses ClamAV via the
clamscan
package. Ensure ClamAV daemon (clamd
) or the clamscan
binary is installed and
properly configured on your server. Using clamd
is generally faster.
// Initialize ClamAV scanner (adjust paths/sockets if necessary)
let clamscanInstance
const initializeClamScan = async () => {
try {
clamscanInstance = await new NodeClam().init({
removeInfected: true, // Automatically remove infected files
quarantineInfected: false, // Don't quarantine, just remove
scanLog: null, // Set to a file path for logging
debugMode: false,
clamscan: {
path: '/usr/bin/clamscan', // Adjust for your system
db: null,
scanRecursively: false,
},
clamdscan: {
socket: '/var/run/clamav/clamd.ctl', // Common socket path, adjust if needed
host: '127.0.0.1',
port: 3310,
timeout: 60000,
localFallback: true, // Use clamscan if clamdscan fails
path: '/usr/bin/clamdscan', // Adjust for your system
bypassTest: false,
},
preference: 'clamdscan', // Prefer clamdscan if available
})
console.log('ClamAV scanner initialized successfully.')
} catch (err) {
console.error('Error initializing ClamAV scanner:', err)
// Decide how to handle initialization failure (e.g., disable scanning, exit)
clamscanInstance = null // Ensure it's null if init fails
}
}
initializeClamScan() // Initialize scanner on application start
// Middleware for virus scanning after upload, before final response
const virusScanMiddleware = async (req, res, next) => {
if (!clamscanInstance) {
console.warn('ClamAV scanner not initialized. Skipping virus scan.')
return next() // Skip scan if initialization failed
}
if (!req.file) {
// No file uploaded, proceed
return next()
}
try {
console.log(`Scanning file: ${req.file.path}`)
const { isInfected, file, viruses } = await clamscanInstance.scanFile(req.file.path)
if (isInfected) {
// File is infected. `removeInfected: true` should have removed it.
console.warn(
`Infected file detected and removed: ${req.file.path}, Viruses: ${viruses.join(', ')}`,
)
// Reject the request - file is already removed by clamscan
return res.status(400).json({
error: `Malware detected: ${viruses.join(', ')}. File rejected.`,
})
}
console.log(`File clean: ${req.file.path}`)
// File is clean, proceed to the next middleware/handler
next()
} catch (error) {
console.error('Virus scan error:', error)
// Attempt to clean up the uploaded file if scan fails and file exists
if (req.file && req.file.path) {
fs.access(req.file.path, fs.constants.F_OK, (err) => {
if (!err) {
fs.unlink(req.file.path, (unlinkErr) => {
if (unlinkErr) console.error('Error removing file after scan error:', unlinkErr)
})
}
})
}
// Pass a generic error to the global error handler
next(new Error('Virus scanning failed.'))
}
}
Defining the upload endpoint with error handling
Combine Multer middleware, rate limiting, virus scanning, and detailed error handling for the
/upload
endpoint.
// Define the POST endpoint for image uploads
app.post(
'/upload',
uploadLimiter,
(req, res, next) => {
// Use multer's single file upload middleware
upload.single('image')(req, res, (err) => {
// Handle Multer-specific errors first
if (err instanceof multer.MulterError) {
console.warn('Multer error:', err.code)
switch (err.code) {
case 'LIMIT_FILE_SIZE':
return res.status(400).json({ error: 'File too large. Maximum size is 5MB.' })
case 'LIMIT_UNEXPECTED_FILE':
return res
.status(400)
.json({ error: 'Unexpected field name. Use "image" for the file field.' })
// Add other Multer error codes if needed (e.g., 'LIMIT_FILE_COUNT')
default:
return res.status(400).json({ error: `Upload error: ${err.message}` })
}
} else if (err) {
// Handle file filter errors or other unexpected errors during Multer processing
console.error('Non-Multer upload error:', err)
// Check if it's our custom file type error
if (err.message.startsWith('Invalid file type')) {
return res.status(400).json({ error: err.message })
}
// Pass other errors to the global handler
return next(err)
}
// Check if a file was actually uploaded after Multer processing
if (!req.file) {
return res.status(400).json({
error:
'No file uploaded or file rejected by filter. Please include a valid image file named "image".',
})
}
// If upload is successful up to this point, proceed to the next middleware (virus scanning)
next()
})
},
virusScanMiddleware,
(req, res) => {
// This final handler runs only if upload succeeded and virus scan passed
console.log(`Successfully processed file: ${req.file.filename}`)
res.status(201).json({
message: 'File uploaded and scanned successfully.',
file: {
filename: req.file.filename, // The secure, randomized filename
originalname: req.file.originalname, // Original filename (for reference)
size: req.file.size,
mimetype: req.file.mimetype,
// Optionally, construct a URL to access the file if serving locally
// url: `/images/${req.file.filename}` // See secure serving endpoint below
},
})
},
)
Secure storage strategies
Local storage security
Storing files locally requires careful permission management and serving files through a controlled endpoint to prevent direct access or path traversal attacks.
// Endpoint to securely serve uploaded images stored locally
app.get('/images/:filename', (req, res, next) => {
const { filename } = req.params
// Validate the filename format strictly (hexadecimal characters + allowed extension)
// This prevents path traversal (e.g., trying to access ../../etc/passwd)
if (!/^[a-f0-9]{32}\.(jpg|jpeg|png|gif)$/i.test(filename)) {
return res.status(400).send('Invalid filename format.')
}
// Construct the full path securely using path.join
const filePath = path.join(uploadDir, filename)
// Check if the file exists *within the secure directory* and is readable
fs.access(filePath, fs.constants.R_OK, (err) => {
if (err) {
// Log the error for debugging, but send a generic 404 to the client
// Avoid revealing specific file system errors
console.error(
`File access error for ${filename}:`,
err.code === 'ENOENT' ? 'Not Found' : err.code,
)
return res.status(404).send('File not found.')
}
// Set appropriate Content-Type header based on file extension
// Consider using a library like 'mime-types' for more robust mapping
res.type(path.extname(filename))
// Stream the file to the client for efficiency
const fileStream = fs.createReadStream(filePath)
fileStream.on('error', (streamErr) => {
console.error('Error streaming file:', streamErr)
// Pass to global error handler if streaming fails
next(new Error('Error serving file.'))
})
fileStream.pipe(res)
})
})
Cloud storage integration (e.g., AWS s3)
For scalability and durability, cloud storage like AWS S3 is often preferred. This requires the
@aws-sdk/client-s3
package.
npm install @aws-sdk/client-s3@^3.500.0 # Use a recent v3 SDK version
// Example using AWS S3 SDK v3
const { S3Client, PutObjectCommand } = require('@aws-sdk/client-s3')
// Configure S3 client (best practice: use IAM roles or environment variables for credentials)
const s3Client = new S3Client({
region: process.env.AWS_REGION, // e.g., 'us-east-1'
// Credentials will be automatically sourced from env vars, shared config, or IAM role
})
// Use Multer memory storage when uploading directly to cloud to avoid temp files
const memoryStorage = multer.memoryStorage()
const uploadToMemory = multer({
storage: memoryStorage,
limits: { fileSize: 5 * 1024 * 1024 }, // 5MB limit
fileFilter: fileFilter, // Reuse the same file filter
})
// Endpoint for uploading directly to S3
// Note: Virus scanning needs adjustment for memory storage.
// You might scan the buffer before uploading or use an S3-integrated scanning service (e.g., Lambda trigger).
app.post('/upload-s3', uploadLimiter, uploadToMemory.single('image'), async (req, res, next) => {
if (!req.file) {
return res.status(400).json({ error: 'No file uploaded or file rejected by filter.' })
}
// Generate a unique filename for S3
const uniqueFilename = `${crypto.randomBytes(16).toString('hex')}${path.extname(req.file.originalname).toLowerCase()}`
const s3Key = `uploads/${uniqueFilename}` // Store in an 'uploads/' prefix (folder)
const params = {
Bucket: process.env.S3_BUCKET_NAME, // Ensure this env var is set
Key: s3Key,
Body: req.file.buffer, // Use the buffer from memoryStorage
ContentType: req.file.mimetype, // Set the correct content type for S3
// Consider setting Cache-Control, ACL (or use bucket policy), etc.
// CacheControl: 'max-age=31536000', // Example: cache for 1 year
}
try {
const command = new PutObjectCommand(params)
const data = await s3Client.send(command)
console.log(`Successfully uploaded to S3: ${s3Key}, ETag: ${data.ETag}`)
// Construct the S3 object URL (ensure bucket/object permissions allow access)
const fileUrl = `https://${process.env.S3_BUCKET_NAME}.s3.${process.env.AWS_REGION}.amazonaws.com/${s3Key}`
res.status(201).json({
message: 'File uploaded to S3 successfully.',
file: {
filename: uniqueFilename,
originalname: req.file.originalname,
size: req.file.size,
mimetype: req.file.mimetype,
url: fileUrl, // URL of the uploaded file in S3
},
})
} catch (error) {
console.error('S3 upload error:', error)
// Pass a generic error to the global error handler
next(new Error('Failed to upload file to S3.'))
}
})
Security best practices: headers, logging, metadata
Security headers
We already added the helmet
middleware near the top of app.js
. It sets various HTTP headers
(like X-Content-Type-Options: nosniff
, X-Frame-Options: DENY
, Strict-Transport-Security
, etc.)
to help protect your application from common web vulnerabilities.
Logging
We added the morgan
middleware for HTTP request logging. In production, consider using the
'combined'
format for more detailed logs and directing output to a file or logging service.
Metadata stripping
Image files often contain metadata (EXIF, IPTC) that might include sensitive information (GPS
location, device details). Consider stripping this metadata after upload, before storing or serving
the image. This typically involves using an image processing library (like sharp
) or a dedicated
tool (like exiftool-vendored
) as part of your post-upload processing workflow. This step is beyond
the scope of basic upload handling but is important for privacy and security.
Error handling
Implement a global error handler as the very last middleware to catch any unhandled errors from your routes or other middleware.
// Global error handler - must be defined LAST, after all other app.use() and routes
app.use((err, req, res, next) => {
console.error('Unhandled API Error:', err.stack || err)
// Clean up uploaded file if an error occurs after upload but before response,
// and it wasn't handled by specific error handlers (like virus scan)
if (req.file && req.file.path && !res.headersSent) {
// Check if file still exists before trying to unlink
fs.access(req.file.path, fs.constants.F_OK, (accessErr) => {
if (!accessErr) {
fs.unlink(req.file.path, (unlinkErr) => {
if (unlinkErr)
console.error('Error removing file during global error handling:', unlinkErr)
else console.log(`Cleaned up file ${req.file.path} due to error.`)
})
}
})
}
// Avoid sending detailed stack traces or sensitive error messages in production
const statusCode = err.status || err.statusCode || 500 // Use status code from error if available
const message =
process.env.NODE_ENV === 'production' && statusCode === 500
? 'Internal Server Error'
: err.message || 'An unexpected error occurred.'
// Ensure response is sent only once
if (!res.headersSent) {
res.status(statusCode).json({ error: message })
}
})
Testing the API with cURL
You can test your local upload endpoint using curl
. Make sure the server is running
(node app.js
).
# Test successful local upload (replace 'path/to/your/image.jpg' with an actual image file)
curl -X POST -F "image=@path/to/your/image.jpg" http://localhost:3000/upload
# Test file too large (using a large file)
curl -X POST -F "image=@path/to/large_image.png" http://localhost:3000/upload
# Test invalid file type (e.g., a text file)
curl -X POST -F "image=@path/to/document.txt" http://localhost:3000/upload
# Test S3 endpoint (if configured and env vars are set)
# cURL -x post -f "image=@path/to/your/image.png" http://localhost:3000/upload-s3
Check the server logs and the secure-uploads
directory (or your S3 bucket) to verify the results.
Conclusion
Building a secure image upload API involves multiple layers of defense: robust input validation (MIME type and extension), strict file size limits, secure filename generation, storing files outside the web root or in cloud storage, rate limiting, virus scanning, proper error handling, and security headers. By implementing these measures using Node.js, Express, and Multer, you can create a reliable and secure REST API for handling file uploads.
For more advanced file processing needs, including transformations, optimization, and complex workflows directly after upload, consider exploring services like Transloadit.