Automate file integrity with 'Sha512sum' in ci/cd
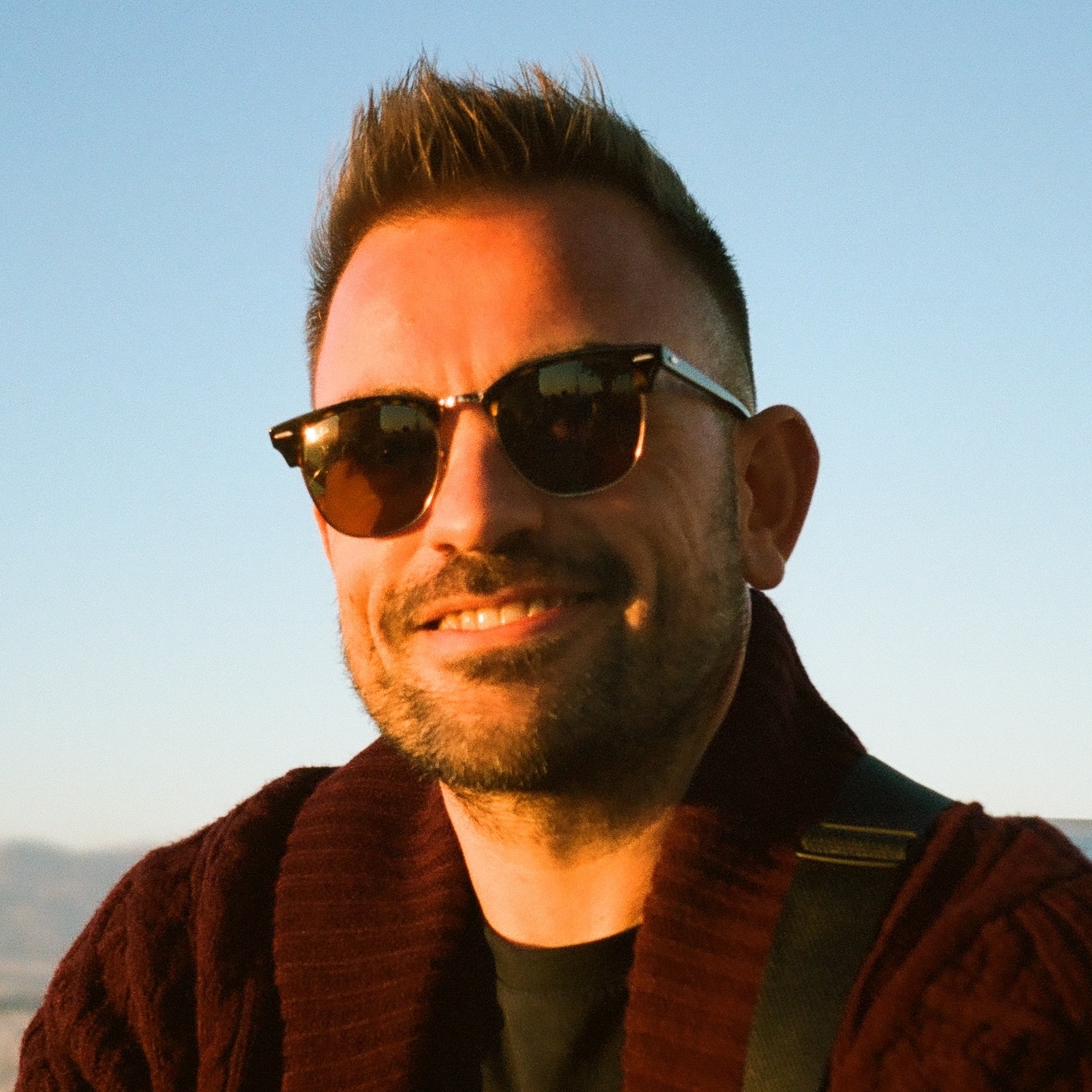
Ensuring file integrity is crucial for developers who want to maintain security and reliability throughout their projects. In this DevTip, we'll explore how to automate file integrity verification using cryptographic hash functions, focusing primarily on SHA-512 while also discussing SHA-256 as a robust alternative.
Why file integrity is important for developers
File integrity refers to the assurance that files have not been altered or corrupted. For developers, maintaining file integrity is essential to:
- Prevent security breaches: Modified files can introduce vulnerabilities
- Ensure consistent builds: Corrupted dependencies can lead to unexpected behavior
- Maintain trust: Users rely on the authenticity of your software
- Verify downloads: Confirm that downloaded files match their original source
Understanding cryptographic hash functions
The sha512sum
tool computes SHA-512 hashes of files, generating a unique fingerprint for each
file. SHA-256 is a viable alternative—especially on 32-bit systems—that also belongs to the SHA-2
family. Both algorithms are designed to detect even the slightest file modifications, providing
strong assurance that your files remain unaltered.
File verification workflow
Generating hashes
Create checksums for your files:
# Single file
sha512sum filename.ext > checksums.sha512
# Multiple files
find . -type f -not -name "checksums.sha512" -exec sha512sum {} \; > checksums.sha512
Verifying files
Verify files against known hashes:
sha512sum -c checksums.sha512
Error handling and common issues
During file verification, you may encounter errors due to permission restrictions, differences in file modes, or mismatches between expected and actual hashes. Below are some common issues and ways to address them:
Permission issues
If you encounter permission errors when generating or verifying checksums, adjust the file permissions and ownership. For example:
# Fix permission errors by setting appropriate permissions
chmod 644 /path/to/files/*
chown $(whoami) /path/to/files/*
# If issues persist on systems with restricted permissions, try using sudo:
sudo chown $(whoami) /path/to/files
Hash mismatch troubleshooting
When the generated checksum does not match the expected value, consider the following checks:
# Compare files byte by byte
cmp file1 file2
# Normalize line endings to resolve potential differences
dos2unix file1
sha512sum file1 > new-checksum.sha512
Ci/cd integration
Integrating file integrity checks into your CI/CD pipelines helps maintain consistency and security across builds.
Github Actions example
Below is an example GitHub Actions workflow that generates and verifies file checksums:
name: Verify File Integrity
on: [push, pull_request]
jobs:
verify:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v4
- name: Generate checksums
run: |
sha512sum assets/* > checksums.sha512
- name: Verify integrity
run: |
sha512sum -c checksums.sha512
continue-on-error: false
Gitlab CI example
Similarly, here is an example configuration for GitLab CI:
verify_integrity:
script:
- sha512sum assets/* > checksums.sha512
- sha512sum -c checksums.sha512
artifacts:
paths:
- checksums.sha512
Secure docker implementation
FROM alpine
# Create non-root user
RUN adduser -D appuser
WORKDIR /app
# Copy files with correct ownership
COPY --chown=appuser:appuser . .
# Switch to non-root user
USER appuser
# Generate and verify checksums
RUN sha512sum important-file.ext > checksums.sha512
CMD ["sha512sum", "-c", "checksums.sha512"]
Performance considerations
When choosing between SHA-512 and SHA-256:
- SHA-256 performs better on 32-bit systems
- SHA-512 can be faster on 64-bit systems
- Both provide strong security guarantees
- File size impacts processing time linearly
Always consider your system architecture and file sizes when choosing between SHA-512 and SHA-256 to balance performance and security.
Conclusion
Implementing file integrity checks with cryptographic hashes enhances your development workflow's security and reliability. By incorporating these checks into your CI/CD pipeline, you ensure consistent builds and safeguard against file corruption. For further enhancements, consider exploring additional file processing tools that integrate file verification with your automation workflows.