Verify file integrity with Go and SHA256
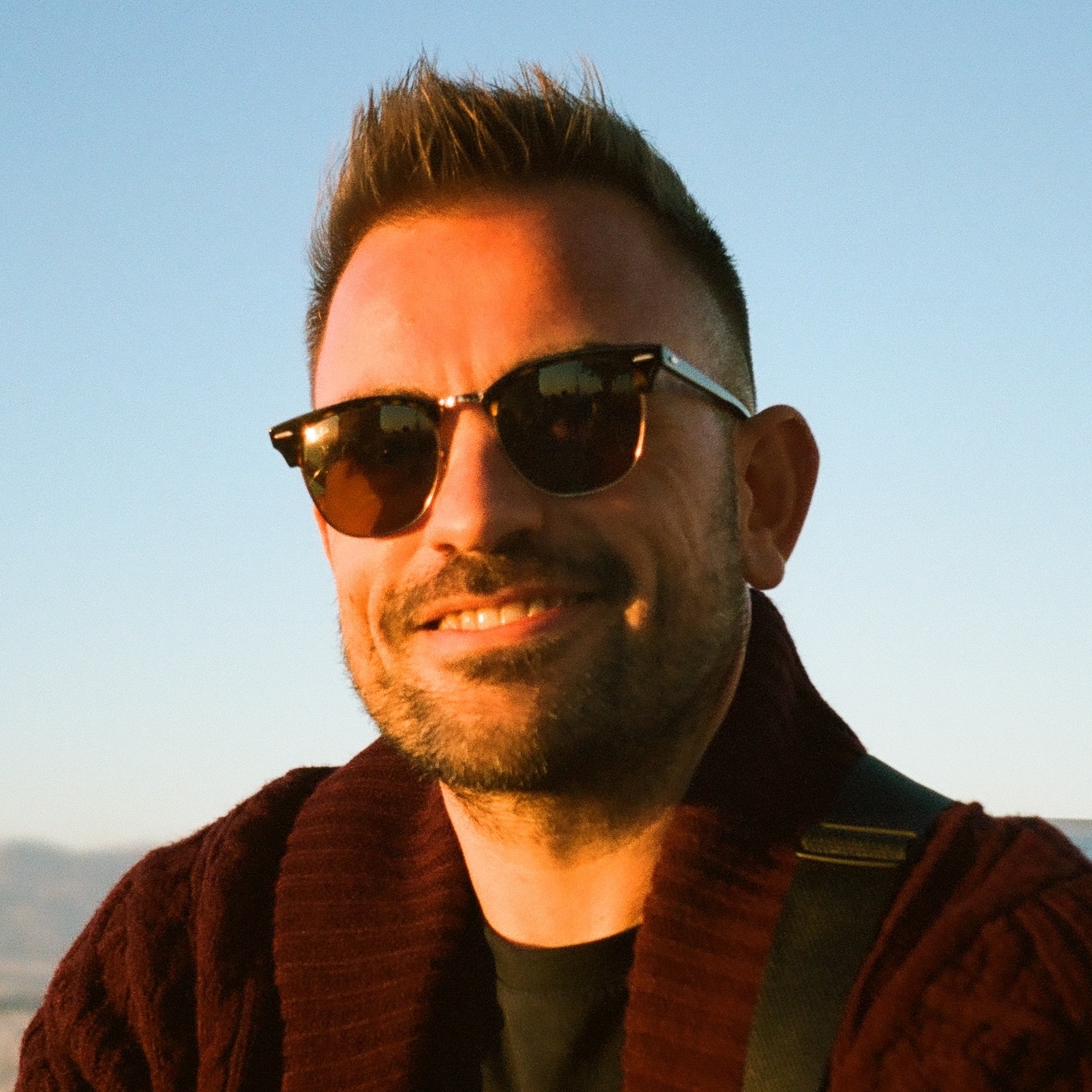
Ensuring file integrity is crucial for developers, especially when handling sensitive data or distributing software. One reliable method to verify file integrity is by using cryptographic hashing algorithms like SHA256. In this DevTip, we'll explore how to implement SHA256 hashing in Go, efficiently handle large files, and discuss best practices for secure file handling.
Why use SHA256 for file verification?
SHA256 is a cryptographic hash function that generates a unique 256-bit (32-byte) signature, often represented as a 64-character hexadecimal string, for any given input. It's widely used due to its collision resistance, meaning it's computationally infeasible to find two different inputs that produce the same hash. This makes SHA256 ideal for verifying that a file hasn't been altered accidentally or maliciously.
Unlike older algorithms like MD5 or SHA1, which are now considered cryptographically broken due to known collision vulnerabilities, SHA256 remains secure against such attacks. This makes it the preferred choice for modern file integrity verification and other security-sensitive applications.
Implementing SHA256 hashing in Go
Go provides built-in support for SHA256 hashing through its crypto/sha256
package. Here's a
straightforward example of hashing a file using io.Copy
, which is generally the recommended
approach:
package main
import (
"crypto/sha256"
"fmt"
"io"
"os"
)
// hashFileSHA256 computes the SHA256 hash of a file.
func hashFileSHA256(filePath string) (string, error) {
// Use os.Open for read-only access.
file, err := os.Open(filePath)
if err != nil {
return "", fmt.Errorf("failed to open file: %w", err)
}
// Ensure the file is closed even if errors occur later.
// Capture the close error only if no other error occurred.
defer func() {
if cerr := file.Close(); cerr != nil && err == nil {
err = fmt.Errorf("failed to close file: %w", cerr)
}
}()
// Create a new SHA256 hash interface.
hash := sha256.New()
// io.Copy efficiently copies data from the file to the hash function.
// It handles buffering internally.
if _, err = io.Copy(hash, file); err != nil {
return "", fmt.Errorf("failed to copy file content to hash: %w", err)
}
// Get the resulting hash sum as a byte slice and format it as hex.
// hash.Sum(nil) appends the hash to a nil slice.
hashInBytes := hash.Sum(nil)
hashString := fmt.Sprintf("%x", hashInBytes)
return hashString, err // err will be nil unless file.Close() failed
}
func main() {
// Example usage: Replace "example.txt" with your file path.
// Ensure "example.txt" exists or handle the error appropriately.
filePath := "example.txt"
// Create a dummy file for the example if it doesn't exist
if _, err := os.Stat(filePath); os.IsNotExist(err) {
dummyData := []byte("This is a test file for SHA256 hashing.\n")
if writeErr := os.WriteFile(filePath, dummyData, 0644); writeErr != nil {
fmt.Println("Error creating dummy file:", writeErr)
return
}
defer os.Remove(filePath) // Clean up the dummy file
}
hash, err := hashFileSHA256(filePath)
if err != nil {
fmt.Println("Error hashing file:", err)
return
}
fmt.Printf("SHA256 hash of %s: %s\n", filePath, hash)
}
This code opens a file, computes its SHA256 hash using io.Copy
, and prints the hexadecimal
representation of the hash. Note the improved error handling for the file.Close()
operation within
the defer
block, which ensures any errors during closing are properly captured and returned if no
prior error occurred.
Efficiently handling large files
When dealing with large files (e.g., gigabytes or more), loading the entire file into memory is
impractical and inefficient. Go's io.Copy
function is designed to handle this scenario
effectively. It reads the file in chunks and writes them to the hash function, managing memory usage
automatically through internal buffering. This makes io.Copy
the preferred method for hashing
files of any size, especially large ones.
However, if you need explicit control over the buffering strategy (perhaps for specific performance
tuning or integration with other buffered I/O), you can use a bufio.Reader
with a custom buffer
size:
package main
import (
"bufio"
"crypto/sha256"
"fmt"
"io"
"os"
)
// bufferedHashFileSHA256 computes the SHA256 hash using manual buffering.
func bufferedHashFileSHA256(filePath string) (string, error) {
file, err := os.Open(filePath)
if err != nil {
return "", fmt.Errorf("failed to open file: %w", err)
}
defer func() {
if cerr := file.Close(); cerr != nil && err == nil {
err = fmt.Errorf("failed to close file: %w", cerr)
}
}()
hash := sha256.New()
// Use a buffered reader for potentially optimized reads.
reader := bufio.NewReader(file)
// Research suggests 128KB buffer often provides good performance for disk I/O.
buf := make([]byte, 1024*128)
for {
// Read a chunk of the file into the buffer.
n, readErr := reader.Read(buf)
if n > 0 {
// Write the chunk read into the hash function.
// Only write the actual number of bytes read (buf[:n]).
if _, writeErr := hash.Write(buf[:n]); writeErr != nil {
// hash.Write should not return error according to docs, but check defensively.
return "", fmt.Errorf("failed to write chunk to hash: %w", writeErr)
}
}
// Check for errors after processing the read chunk.
if readErr != nil {
// If it's the end of the file, break the loop.
if readErr == io.EOF {
break
}
// Otherwise, return the read error.
return "", fmt.Errorf("failed during file read: %w", readErr)
}
}
hashInBytes := hash.Sum(nil)
hashString := fmt.Sprintf("%x", hashInBytes)
return hashString, err // err will be nil unless file.Close() failed
}
// main function would be similar to the previous example, calling bufferedHashFileSHA256
// func main() { ... }
This approach explicitly manages the buffer size. A 128KB buffer is often cited as a good starting
point for balancing memory usage and disk I/O efficiency on many systems. However, the optimal size
can vary depending on the hardware and operating system. For most use cases, the simpler io.Copy
approach is recommended as it handles these optimizations internally and often performs just as well
or better.
Performance considerations
While io.Copy
is generally recommended, understanding the performance implications can be useful.
Let's look at a simple benchmark comparing the two approaches:
package main_test // Use a test package
import (
"crypto/rand"
"os"
"testing"
// Assume hashFileSHA256 and bufferedHashFileSHA256 are in the 'main' package
// Adjust import path if necessary, e.g., "your_module_path"
// main "your_module_path"
)
// Helper function to create a temporary file for benchmarking
func createTempFile(size int) (string, error) {
data := make([]byte, size)
if _, err := rand.Read(data); err != nil {
return "", err
}
tmpfile, err := os.CreateTemp("", "hashtest_*.tmp")
if err != nil {
return "", err
}
if _, err := tmpfile.Write(data); err != nil {
tmpfile.Close()
os.Remove(tmpfile.Name())
return "", err
}
if err := tmpfile.Close(); err != nil {
os.Remove(tmpfile.Name())
return "", err
}
return tmpfile.Name(), nil
}
func BenchmarkHashFile(b *testing.B) {
// Create a reasonably sized test file (e.g., 10MB)
fileSize := 10 * 1024 * 1024
filePath, err := createTempFile(fileSize)
if err != nil {
b.Fatalf("Failed to create temp file: %v", err)
}
defer os.Remove(filePath) // Clean up the file after benchmarks
b.Run("io.Copy", func(b *testing.B) {
b.ReportAllocs() // Report memory allocations
b.SetBytes(int64(fileSize)) // Report throughput (Bytes/op)
b.ResetTimer()
for i := 0; i < b.N; i++ {
// Replace with actual function call if needed
// _, err := main.hashFileSHA256(filePath)
_, err := hashFileSHA256(filePath) // Assuming functions are accessible
if err != nil {
b.Fatalf("hashFileSHA256 failed: %v", err)
}
}
})
b.Run("buffered-128KB", func(b *testing.B) {
b.ReportAllocs()
b.SetBytes(int64(fileSize))
b.ResetTimer()
for i := 0; i < b.N; i++ {
// Replace with actual function call if needed
// _, err := main.bufferedHashFileSHA256(filePath)
_, err := bufferedHashFileSHA256(filePath) // Assuming functions are accessible
if err != nil {
b.Fatalf("bufferedHashFileSHA256 failed: %v", err)
}
}
})
}
// Dummy implementations for benchmark to run standalone if needed
// In a real scenario, these would be imported from the main package.
func hashFileSHA256(filePath string) (string, error) { /* ... implementation ... */ return "", nil }
func bufferedHashFileSHA256(filePath string) (string, error) { /* ... implementation ... */ return "", nil }
(Note: You'd need to place the actual hashFileSHA256
and bufferedHashFileSHA256
functions where
the benchmark test can access them, typically by putting the benchmark in a _test.go
file within
the same package, or importing the package containing them.)
Running benchmarks like this (go test -bench=. -benchmem
) often shows that io.Copy
performs
comparably to, or sometimes even better than, manual buffering with common buffer sizes like 128KB,
while requiring less code and handling edge cases internally.
Best practices for hashing large files
- Use
io.Copy
when possible: It's the idiomatic Go way, simpler, less error-prone, and handles buffering efficiently for files of all sizes. - Choose appropriate buffer sizes: If using manual buffering (
bufio.Reader
), start with 128KB as a generally good default, but consider benchmarking for your specific workload if performance is critical. - Handle errors gracefully: Always check for errors when opening files (
os.Open
), reading data (io.Copy
orreader.Read
), and especially when closing files (file.Close()
). Usedefer
for reliable cleanup. - Verify hashes securely: When comparing a computed hash against an expected hash, use a constant-time comparison function to prevent timing attacks.
Practical example: verifying file integrity
To verify file integrity, you compute the hash of the file you received or downloaded and compare it against a known, trusted hash value (e.g., one provided by the software distributor on a secure webpage). It's crucial to perform this comparison securely using a constant-time algorithm.
package main
import (
"crypto/sha256" // For hash function
"crypto/subtle" // For constant-time comparison
"encoding/hex"
"fmt"
"io"
"os"
)
// Re-use hashFileSHA256 function from earlier example
func hashFileSHA256(filePath string) (string, error) {
file, err := os.Open(filePath)
if err != nil { return "", fmt.Errorf("failed to open file: %w", err) }
defer func() {
if cerr := file.Close(); cerr != nil && err == nil {
err = fmt.Errorf("failed to close file: %w", cerr)
}
}()
hash := sha256.New()
if _, err = io.Copy(hash, file); err != nil { return "", fmt.Errorf("failed to copy file content: %w", err) }
return fmt.Sprintf("%x", hash.Sum(nil)), err
}
// verifyFileIntegrity computes the file's SHA256 hash and compares it
// securely against an expected hash string.
func verifyFileIntegrity(filePath, expectedHashHex string) (bool, error) {
// Compute the hash of the actual file.
computedHashHex, err := hashFileSHA256(filePath)
if err != nil {
// If hashing fails (e.g., file not found), integrity cannot be verified.
return false, fmt.Errorf("failed to compute hash: %w", err)
}
// Decode the expected hash from hex string to byte slice.
expectedHashBytes, err := hex.DecodeString(expectedHashHex)
if err != nil {
// If the expected hash string is invalid hex, report error.
return false, fmt.Errorf("invalid expected hash format: %w", err)
}
// Decode the computed hash from hex string to byte slice.
computedHashBytes, err := hex.DecodeString(computedHashHex)
if err != nil {
// This should ideally not happen if hashFileSHA256 works correctly.
return false, fmt.Errorf("invalid computed hash format: %w", err)
}
// Compare the byte slices using constant-time comparison.
// subtle.ConstantTimeCompare returns 1 if equal, 0 otherwise.
// Both slices must have the same length for a valid comparison.
// SHA256 hashes are always 32 bytes long.
if len(expectedHashBytes) != sha256.Size || len(computedHashBytes) != sha256.Size {
// If lengths don't match (e.g., truncated hash provided), they are not equal.
return false, nil
}
hashesMatch := subtle.ConstantTimeCompare(expectedHashBytes, computedHashBytes) == 1
return hashesMatch, nil
}
func main() {
// Example usage
filePath := "example.txt"
// Assume this is the known good hash obtained securely
// This hash corresponds to "This is a test file for SHA256 hashing.\n"
expectedHash := "f2ca1bb6c7e907d06dafe4687e579fce76b37e4e93b7605022da52e6ccc26fd2"
// Create the dummy file again for this example
if _, err := os.Stat(filePath); os.IsNotExist(err) {
dummyData := []byte("This is a test file for SHA256 hashing.\n")
if writeErr := os.WriteFile(filePath, dummyData, 0644); writeErr != nil {
fmt.Println("Error creating dummy file:", writeErr)
return
}
defer os.Remove(filePath) // Clean up
}
match, err := verifyFileIntegrity(filePath, expectedHash)
if err != nil {
fmt.Println("Error verifying file integrity:", err)
} else {
if match {
fmt.Printf("File '%s' integrity verified successfully.\n", filePath)
} else {
fmt.Printf("File '%s' integrity check failed: Hashes do not match.\n", filePath)
}
}
}
Using subtle.ConstantTimeCompare
is critical here. A naive byte-by-byte comparison might return
early if a mismatch is found near the beginning. Attackers could potentially measure the time taken
for the comparison to leak information about the expected hash. Constant-time comparison takes the
same amount of time regardless of where the mismatch occurs (or if there's no mismatch), mitigating
this risk.
Security considerations
When implementing file integrity verification, keep these security points in mind:
- Use secure hash algorithms: Always prefer SHA256 or stronger algorithms (like SHA3 variants or SHA512) over deprecated ones like MD5 or SHA1, which are vulnerable to collision attacks.
- Implement constant-time comparisons: As shown above, use
subtle.ConstantTimeCompare
(or equivalent secure comparison functions in other languages) when checking hashes to prevent timing attacks. - Secure hash distribution: Ensure that the expected hash values are obtained and distributed securely. If an attacker can tamper with the expected hash, the integrity check becomes meaningless. Use trusted channels like HTTPS websites, signed manifests, or secure communication protocols.
- Protect against hash manipulation: If storing expected hashes (e.g., in a database or configuration file), ensure these stored values are protected against unauthorized modification through access controls and potentially cryptographic signing.
- Consider using HMAC: For verifying data authenticity and integrity, especially when a shared secret key is involved, consider using HMAC (Hash-based Message Authentication Code), such as HMAC-SHA256. HMAC combines a secret key with the hash, ensuring that only parties with the key could have generated the hash.
Common pitfalls
- Ignoring file close errors: Failing to check the error returned by
file.Close()
can mask underlying issues like data not being fully flushed to disk. Usedefer
with proper error checking. - Using weak hashing algorithms: Relying on MD5 or SHA1 for security-sensitive integrity checks is dangerous due to known vulnerabilities.
- Loading entire files into memory: Reading large files completely into memory before hashing
can lead to excessive memory consumption and program crashes. Use streaming approaches like
io.Copy
or buffered reading. - Insecure hash comparison: Using simple string or byte slice equality checks (
==
) for hash comparison can expose your application to timing attacks. Always use constant-time comparison functions. - Not validating input paths: Failing to sanitize or validate file paths provided by users or
external systems can lead to directory traversal vulnerabilities (
../../etc/passwd
).
By following these guidelines and using Go's standard library features, you can confidently implement robust SHA256 hashing to verify file integrity, ensuring your files remain secure and unaltered during storage or transmission.
For robust file handling and processing in the cloud, services often rely on strong hashing mechanisms like SHA256 for tasks ranging from ensuring upload integrity to deduplication and cataloging; you can explore options like Transloadit for managed file processing workflows.